React - Components
In the previous article, we learned about the basics in React—how to build a basic React application and creating our first working environment. All of the code that we’re writing at this stage is inside of HTML script tags which you’ll find it below where it’s written ALL THE CODE INSIDE HERE.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>My first react app</title>
<script src="https://unpkg.com/react@16/umd/react.development.js"></script>
<script src="https://unpkg.com/react-dom@16/umd/react-dom.development.js"></script>
<script src="https://unpkg.com/babel-standalone@6.15.0/babel.min.js"></script>
</head>
<body>
<div id="content"></div>
<script type="text/babel">
// ALL THE CODE INSIDE HERE
</script>
</body>
</html>
You don’t have to copy that HTML, paste it into an editor, save it somewhere, and refresh after every change. You can of course use CodePen, and then just click straight on the part with the JavaScript. If for example, you go to https://codepen.io/barzik/pen/JBWLZB, you can make changes directly in the JavaScript area, and then see the changes live on the lower part of the screen.
Last time we talked about how a React application constitutes the playing field on which the components get to play. But hold on. What exactly is a component? Components are the bread and butter of modern JavaScript development—the building blocks of UI. For example, if I have a list in HTML, we could say that it is divided into components. Let’s have a look:
<div> // "div" Component
<ul> // "ul" Component
<li>First Item</li> // "li" Component
<li>Second Item</li> // "li" Component
<li>Third Item</li> // "li" Component
</ul>
</div>
So really, we could compare components to elements in HTML. Each element is more or less a component that has its own particular functionality and importance, and one component can contain another. In React (in Angular as well), all the functionality is found inside the components and is not just spread around the code. Yet this doesn’t really hold water since in complex applications and sites there is functionality that must be divided between components with each one having its own use and importance. I’ll expand upon this in future articles, but for now you can take it as canon. Most Angular code is found inside a component.
So what do components look like? Just like a class. Take for example, if I want to make a Hello World component. I would do it like this:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>My first react app</title>
<script src="https://unpkg.com/react@16/umd/react.development.js"></script>
<script src="https://unpkg.com/react-dom@16/umd/react-dom.development.js"></script>
<script src="https://unpkg.com/babel-standalone@6.15.0/babel.min.js"></script>
</head>
<body>
<div id="content"></div>
<script type="text/babel">
class HelloWorld extends React.Component {
render() {
return <p>Hello world!</p>
}
}
const target = document.getElementById('content');
ReactDOM.render(
<HelloWorld/>,
target
);
</script>
</body>
</html>
What’s going on here? Easy really. The main component is represented as a regular class that inherits from React.Component. The name is of critical importance. What’s inside is the functionality of the component. In this case, not much really. It just returns JSX of Hello World. Easy, right?
class HelloWorld extends React.Component {
render() {
return <p>Hello world!</p>
}
}
Once we’ve declared a component like this, we can use it as we wish, just like in HTML. Yes, yes, it’s true. I can put the </HelloWorld> tag in ReactDOM.render just like I put all that other stuff in before. It’s really just that simple.
Play around with this a little on your own. If your not familiar with classes, then check out our article on the subject from our ES6 series.
We can of course put as many components as we want into ReactDOM.render—just make sure everything is wrapped as necessary. Why, you ask? If not we’re bound to encounter render errors. Try it and enjoy. In the next article, we’ll talk about components that take arguments and we’ll have some fun with a component that’s a little more interesting than Hello World. Till then.
Previous Article: intro to React | Next article: components with parameters |
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
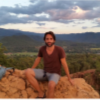
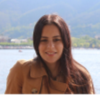
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment