PyMongo - A simple wrapper in python for MongoDB
MongoDB is a cross-platform, document oriented database that provides, high performance, high availability, and automatic scalability. Interacting with MongoDB using Python can be a straightforward process if you have the right tools and methods. In this tutorial we will learn how to simplify our MongoDB database operations by using a python distribution called PyMongo. This is a highly recommended way to work with MongoDB from python. For this tutorial, we are assuming that you have already installed MongoDB and its up and running on port 27017.
Installation of PyMongo
- Install pip on your system
Download the get-pip.py . Then run it from the command prompt.
$ python get-pip.py
- Install pymongo python package using pip
$ pip install pymongo
Getting started with PyMongo
First you need to start the MongoDB server so we can connect PyMongo to MongoDB adapter. Run this command to start your mongodb server.
$ cd path/to/mongo/bin
$ mongod --dbpath /path/to/data/dir
Now we will connect to this mongo server which is running on port number 27017 from python code using PyMongo module’s MongoClient() method. In PyMongo 2.4.1 the Connection() method has been deprecated. So we are using MongoClient() method.
import pymongo
if __name__ == '__main__':
# Connection to MongoDB
try:
conn=pymongo.MongoClient(‘localhost’,27017)
print "Connection established successfully!!!"
except pymongo.errors.ConnectionFailure, e:
print "Could not connect to MongoDB: %s" % e
Retrieving data from MongoDB
Reading a document from MongoDB is very much easy. All you have to do is call a find method on the collection and it will return all the documents in the collection. Then you can iterate through all the documents using a for loop.
-
Selecting the database
db = conn.mydb ← Your database name
print db
Output:
Connected successfully!!!
Database(MongoClient(host=['localhost:27017'], document_class=dict, tz_aware=False, connect=True), u'mydb')
-
Selecting the collection
collection = db.users ← Your collection name
print collection
Output:
Connected successfully!!!
Collection(Database(MongoClient(host=['localhost:27017'], document_class=dict, tz_aware=False, connect=True), 'mydb'), 'business')
-
Fetching the documents
docs = collection.find()
for doc in docs:
print doc
Output
Connected successfully!!!
{
"_id": {"$oid": "58a990f713e4367839a0243c"},"gender": "male", "age": "23", "name": "akash", "stream": "CSE"
},
{
"_id": {"$oid": "58a9914713e4367839a0243d"}, "gender": "male", "age": "24", "name": "Rajan", "stream": "VLSI"
},
{
"_id": {"$oid": "58a99beb13e4367839a0243e"}, "gender": "male", "age": "19", "name": "alex", "stream": "Network"
}
-
Getting a single document using find_one()
The find_one() method selects and returns a single document from a collection and returns that document (or None if there are no matches). This method is very useful when you know there is only one matching document, or you are only interested in the first match.
doc = collection.find_one()
print doc
Output
{
"_id": {"$oid": "58a990f713e4367839a0243c"},"gender": "male", "age": "23", "name": "akash", "stream": "CSE"
}
Querying by fields
Querying the collections to find any particular document is also easy. To build a query, you just need to mention a dictionary with the properties you wish the results to match. For example, this query will match all documents in the Users collection with name = ‘akash’.
doc = collection.find({‘name’:’akash’}) ← Specify all conditions in this dict
Output
{
"_id": {"$oid": "58a990f713e4367839a0243c"},"gender": "male", "age": "23", "name": "akash", "stream": "CSE"
}
Here is one more example for you—this query will fetch every document which has age greater than 20.
doc = collection.find({age: {‘$gte’ : ‘20’} })
Output
{
"_id": {"$oid": "58a990f713e4367839a0243c"},"gender": "male", "age": "23", "name": "akash", "stream": "CSE"
},
{
"_id": {"$oid": "58a9914713e4367839a0243d"}, "gender": "male", "age": "24", "name": "Rajan", "stream": "VLSI"
}
Inserting new documents
To insert a document into a collection we can use the insert_one() method:
# Insert one document
doc = {'name':'Sue','age':'22','gender':'female','stream':'IT'}
collection.insert_one(doc)
Output
Connected successfully !!!
Document inserted successfully
Updating the documents
Pymongo can update the documents in a several ways. To update any document, we need its primary key to identify the document uniquely which is ‘_id’ in this case. Suppose we want to update the age of a user whose name is akash. Let’s find that document first.
doc = collection.find({‘name’:’akash’})
Output
{
"_id": {"$oid": "58a990f713e4367839a0243c"},"gender": "male", "age": "23", "name": "akash", "stream": "CSE"
}
From here we can get the value of _id field to update this document. Add this code in your script for updating.
#Update the documents collection.update({"_id":ObjectId('58a990f713e4367839a0243c')},{"age":"25"})
Output
Connected successfully!!!
{'age': '25', '_id': ObjectId('58a990f713e4367839a0243c')}
Use this approach with caution because it will update the whole document. Have a look at this output.
If you observe this output properly then you will notice that all other fields are gone! Only the updated field is there in the document. If you don’t want to lose this data then we have to use another approach to update the document.
# Update using $set operator
collection.update({"_id":ObjectId('58abb6a586e76105aea02ee1')},{"$set":{"age":"25"}})
print collection.find_one({"_id":ObjectId('58abb6a586e76105aea02ee1')})
Output
Connected successfully!!!
{'gender': 'male', 'age': '25', '_id': ObjectId('58abb6a586e76105aea02ee1'), 'name': 'Akash', 'stream': 'CSE'}
Multi operatorUpdate Operators
By default MongoDB only modifies the first document that matches the query. If you want to modify all documents that match the query add multi=True.
- Inc operator
The inc operator increments a value by a specified amount if the field is present in the document. If the field does not exist, $inc sets the field to the number value.
# Increment the value of age field by 9
collection.update({"_id":ObjectId('58abb6a586e76105aea02ee1')},{"$inc":{"age":9}})
- Rename operator
The rename operator updates the name of a field. The new field name must differ from the existing field name.
# Deletes the age field from document.
collection.update({"_id":ObjectId('58abb6a586e76105aea02ee1')},{"$rename":{"gender:"sex"}})
- Unset operator
The unset operator deletes the specific field. If documents match the initial query but do not have the field specified in the unset operation, then the statement will have no effect on the document.
# Deletes the age field from document.
collection.update({"_id":ObjectId('58abb6a586e76105aea02ee1')},{"$unset":{"age:""}})
Deleting data using PyMongo
You can remove databases, collections and documents using Pymongo very easily.
- Removing database
# Remove database mydb
conn.drop_database("mydb")
- Removing collection
# Remove collection Users
conn.drop_collection("Users")
- Removing documents
# Remove document with particular ID
collection.remove({"_id":ObjectId('58abb6a586e76105aea02ee1')})
Get statistics of your databaseSome other useful methods
db.command({'dbstats': 1})
Output:
{
"storageSize": 151552.0,
"ok": 1.0,
"avgObjSize": 423.5,
"db": "mydb",
"indexes": 5,
"objects": 22,
"collections": 5,
"numExtents": 0,
"dataSize": 9317.0,
"indexSize": 131072.0
}
- Get statistics of any collection
db.command({'collstats': 'Users'})
In this tutorial, we saw how to use MongoDB Insert, Read, Update, and Delete Using Python. It demonstrates how to implement the basic CRUD operation in MongoDB using Python. Hope you liked it and feel free to leave a comment below.
Recent Stories
Top DiscoverSDK Experts
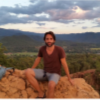
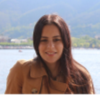
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment