Node.js Backend Development - Global Objects
In the previous article we learned about sending static files to the browser. We are learning Node.js very fast by building up an application and a micro framework of our own. Before we continue further, we also need to learn about the Global Objects available in Node.js so that we can use them where necessary and make our life easier. The code from all the articles can be found on the Github repository Learn Node.js With Sabuj. Code for each article is separated into branches on the repository. Codes for this current article can be found in the branch named 009_global_objects.
So, what are global objects? Global objects are objects that are available in all the modules or scripts without importing them from anywhere else. By object, I do not mean that all of them are plain old JavaScript objects. These are functions, strings, and plain old JavaScript objects. We are not cover all of the global objects here in this article—we are just going to talk about the ones that we might need the most in our backend web development projects.
Before we proceed using these objects let's create a view and set a URL pattern to access that view. We do not want to see the output on the console, instead we want to see them on the browser. Create a file called globalo.js and export a function named globalo from it.
globalo.js
exports.globalo = function(request, response, url){
}
routes.js
var home = require("./views/home").home;
var form = require("./views/form").form;
var process_form = require("./views/process_form").process_form;
var view_404 = require("./views/view_404").view_404;
var static_files = require("./views/static_files").static_files;
var globalo = require("./views/globalo").globalo;
var routes = [
{
pattern: '',
handler: home
},
{
pattern: 'form',
handler: form
},
{
pattern: 'submit-form',
handler: process_form
},
{
pattern: 'static/',
handler: static_files
},
{
pattern: 'globalo',
handler: globalo
}
]
exports.routes = routes;
exports.view_404 = view_404;
So, we will need to browse to http://localhost:5000/global to see our results.
We do not want to serve HTML to the browser, instead we want to serve pure plain text. So, we will need to tell user agents through a Content-Type header that we are serving plain text. Also, we need to provide a 200 status. Let's write the head.
exports.globalo = function(request, response, url){
response.writeHead(200, {
'Content-Type': 'text/plain'
});
var result = "";
response.end(result, "utf8");
}
Our setup is ready. Now we will keep putting our results in the result variable.
__filename
filename is the file name of script that is being executed. By this I do not mean that it is the file name of the main.js. It is the file name in which you use the filename varibale. If you use it inside main.js then it is its file name, if you use it inside globalo.js then it is file name of globalo.js. By file name I mean the fully qualified file name. Let's use it inside globalo.js*.
exports.globalo = function(request, response, url){
response.writeHead(200, {
'Content-Type': 'text/plain'
});
var result = "";
result += "File name of the current script is: " + __filename + "\n";
response.end(result, "utf8");
}
Fire up the terminal, start the main.js, go to the browser and browse to http://localhost:5000/globalo.
It will output something similar to the following:
File name of the current script is: D:\Learn-NodeJS-with-Sabuj\views\globalo.js
As I told you, it gives you the fully qualified file name.
__dirname
dirname is similar to filename. It gives the directory name where the executing script is living. Let's see it's use in action.
exports.globalo = function(request, response, url){
response.writeHead(200, {
'Content-Type': 'text/plain'
});
var result = "";
result += "__filename: " + __filename + "\n";
result += "__dirname: " + __dirname + "\n";
response.end(result, "utf8");
}
Restart the application and you will see the following result.
__filename: D:\Learn-NodeJS-with-Sabuj\views\globalo.js
__dirname: D:\Learn-NodeJS-with-Sabuj\views
console
In our development we often use the console object to output data to the console or take input from the console. The most used method of this object is log(), info(), and error(). It also has other methods like, warn(), dir(), time(), trace() and assert(). I am not showing any demo of it as I have used it numerous times in my previous articles on JavaScript.
setTimeout
This function is used to schedule a work item after some milliseconds. As the first parameter, we pass a callback function that will be called after the specified milliseconds have passed. The second parameter is the number of milliseconds after which the callback will be called.
Keep in mind that it is not guaranteed that the callback will be called after the exact amount of milliseconds. It is supposed to be executed after that amount of time, but it can be more. If you have any asynchronous code in your application that takes a lot time to process then you will see a significant lag in the execution of the callback. For example, you are processing hundreds of megabytes of text by loading it in the memory (as a string). In this case it is no longer an io operation and you cannot avoid its synchronous behavior.
I am not going to show any demo of it on the browser. Here is a simple example of it.
setTimeout(function(){
console.log("One second passed");
}, 1000);
setInterval
This is similar to the previous function, but in this case the callback function will continue to run in a loop after the provided number of milliseconds.
clearInterval and clearTimeout
After scheduling a callback with setTimout or setInterval a value is returned that can be used to cancel that schedule anytime. Try it yourself.
Conclusion
There are other objects that I am not going to discuss in this article. We will often see dirname and filename in our future backend web application development. Also, keep in mind that in this discussion I had no intention to cover objects like Data and Math, as they deserve their own article. Keep practicing with JavaScript and Node.js, and keep updated with this blog.
About the Author
My name is Md. Sabuj Sarker. I am a Software Engineer, Trainer and Writer. I have over 10 years of experience in software development, web design and development, training, writing and some other cool stuff including few years of experience in mobile application development. I am also an open source contributor. Visit my github repository with username SabujXi.
Recent Stories
Top DiscoverSDK Experts
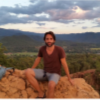
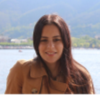
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment