New Working With Files In JavaScript With Node.js
For some very good reasons, JavaScript running on browsers cannot directly interact with the file system. But when we work on the server side with Node.js, working with the file system is a very common task. Nowadays, we create desktop, mobile, and IoT apps with the help of Node.js, so begin able to work with the file system via Node.js is very important for a JavaScript programmer. In this article I am going to show you different aspects and ways of working with the file system. Before diving into the discussion you need to setup your system.
Setting Up
Install the latest version of Node.js on your system. You will get relevant instructions on the official website of Node.js for your system. Make sure that it is available on your system path. Create a plain text file called practice_fs.js to write code in. Use any code editor or IDE you like. I am just going to use the default plain text editor on my system. Run the js file from command line by writing: node practice_fs.js. We need to require the builtin fs module to work with the file system. So, our initial code should look like the following:
var fs = require('fs');
Run the js file to check if everything is alright.
Reading Files
Create a plain text file named info.txt in the current working directory with the following contents.
Hello World!
I am nothing but plain text.
To read a file we need to use the readFile() method of fs. It is an asynchronous method from the fs module. The first parameter is the file name, the second parameter is options and the third parameter is a callback function. If we pass a string for the options parameter then that string is considered as the encoding for the file to be read.
The callback function accepts two parameters. The first parameter is for errors; if any error has occurred that error object is passed through the error parameter. The second parameter is for the data. The content of the file is passed as data upon the successful reading of the file.
The purpose of our code is to read the contents of the info.txt file and display them on the screen. The code looks like the following:
var fs = require('fs');
fs.readFile("info.txt", "utf-8", function(err, data){
if(err){
console.error("Error!! in reading the file");
console.error(err);
return;
}else{
console.log(data);
}
});
Run the js file to see the following output:
Hello World!
I am noting but plain text.
Let's do an intentional mistake by providing the file name as 'info .txt' instead of 'info.txt'. That file name does not exist in the current working directory. Run it to see the following output on the console.
Error!! in reading the file
{ Error: ENOENT: no such file or directory, open '****\info .txt'
at Error (native)
errno: -4058,
code: 'ENOENT',
syscall: 'open',
path: '****\\info .txt' }
Writing File
There is a method called writeFile() in the fs module. It accepts the path as the first parameter, data as the second parameter, options as the third parameter, and errors as the fourth parameter. Like readFile() the options parameter—when passed as a string—is considered to be the encoding for the file.
The callback function of the writeFile() method only accepts one parameter. If an error occurs during writing to the file, an error object is passed through the error parameter to the callback function.
Let's write the following text to a file called 'info2.tx'. Remember that it is not necessary for the file to already exist to be written into the file system. If the file is not present then it will automatically be created.
var fs = require('fs');
var data = "This line is the content for the info2.txt file.";
fs.writeFile("info2.txt", data, "utf-8", function(err){
if(err){
console.error("Error!! in writing the file");
console.error(err);
}
});
Run the js file. Go to the current working directory and you will see a new file named info2.txt. Open the file to verify that it contains the data we intended to write into it.
Deleting A File
There is no method called delete, rm or remove in the file system (fs) module. This may seem a bit not intuitive for some people. Don't worry, there is a method called unlink()—this is the one that follows your order and removes the file you want to be removed or deleted from the file system. Remember that this type of deletion of a file does not put it into the recycle bin.
var fs = require('fs');
fs.unlink("info.txt", function(err){
if(err){
console.error(err);
}
});
Run the js file to see that info.txt file is gone from the current working directory or the file system.
Notice that this method accepts the file path as the first parameter and a callback function as the second parameter. The callback function accepts an error parameter. If an error occurs during removing or unlinking of the file, an error object is passed to the callback function.
Renaming A File
Let's rename our 'info2.txt' file to 'info_two.txt'. How do we do that? The fs module has a method called rename() that accepts the old path name as the first parameter, the desired new path name as the second parameter and a callback function as the third parameter. The callback function accepts an error parameter. Our code should look like the following:
var fs = require('fs');
fs.rename("info2.txt", "info_two.txt", function(err){
if(err){
console.error(err);
}
});
Run the js file and go to you current working directory. See that there is no file called info2.txt but instead there is a file called info_two.txt. Open the file to verify that it is the file we renamed it to.
Conclusion
There are a lot of things we can do on the file system and the fs module contain a lot of methods for working on different aspects of the file system. All of them cannot be covered in a single article but I will write more articles on this topic in the near future. You are advised to take a look at the official API reference documentation of the fs module and try to practice with different methods. Remember that some methods are destructive; experiment with caution. If you delete a file or directory or modify it's contents, you cannot get that back from the recycle bin or from anywhere else. Keep practicing JavaScript with Node.js.
Recent Stories
Top DiscoverSDK Experts
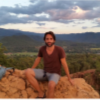
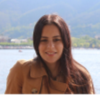
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment