New Object Methods in ES2017
Objects were always problematic to iterate over in JavaScript. This is mostly due to the prototypes that complicate matters of iteration, and also because there really aren’t any good methods for iterating over objects. ES2017 tries to ease the issue by using entries—methods that return all of the enumerable property [key, value] pairs of an object in an orderly fashion as an array. It’s called as an Object method. Here’s an example:
let myObj= {one: 1, two: 2, someProperty: 'Some Value'};
console.log( Object.entries(myObj) ) //[ ["one":, 1], ["two":, 2], ["someProperty":, "Some Value"] ]
As you can see, I have an object from which I simply extract all of the key/value pairs into an array using Object.entries. As funny as it sounds, until now there has never been a good way to do this in JavaScript. Notice that the properties we receive are those of the object alone and not properties that come from the prototype. This is great for iteration using for of. Here’s an example:
let myObj= {one: 1, two: 2, someProperty: 'Some Value'};
for (let [k,v] of Object.entries(myObj)) {
console.log(`${k} : ${v}`);
}
//"one : 1"
//"two : 2"
//"someProperty : Some Value"
It’s a bit like the conversion of an object to an array that contains all of the components of the object in the familiar key/value format. From here you can do whatever you like with the array. Run an iteration on it, or put it into a map object. Whatever you feel like.
If the keys don’t interest us and we want to only use the values, we can use the values method which does exactly the same thing but only with the values inside the object.
let myObj= {one: 1, two: 2, someProperty: 'Some Value'};
console.log( Object.values(myObj) ) //[1, 2, "Some Value"] ]
for (let k of Object.values(myObj)) {
console.log(k);
}
//1
//2
//"Some Value"
If you want to mess around with it, here is the codepen:
getOwnPropertyDescriptors
Another new feature of Object is getOwnPropertyDescriptors. It’s pretty easy—it just brings the descriptors of the object. If you’re not familiar with descriptors, I’ll quickly note that they are properties of an object. For example, the methods get and set, or other properties. When we use getOwnPropertyDescriptors we can receive information on all of these properties. Let’s look at an example:
const obj = {
somePropery: 123,
get getSomeProperty() { return this.somePropery },
};
console.log(Object.getOwnPropertyDescriptors(obj));
The result we receive is obj with a full description of the descriptors, not just the value of the properties, but also additional information about them. For instance:
Object
getSomeProperty:Object
configurable:true
enumerable:true
get:getSomeProperty()
set:undefined
somePropery:Object
configurable:true
enumerable:true
value:123
writable:true
We use this especially for the cloning of complex objects. For instance, assign to an complex object is likely to cause unpredictable behavior and loss of information about the properties. But the wise use of getOwnPropertyDescriptors can solve the problem. Here’s an example:
const obj = {
somePropery: 123,
get getSomeProperty() { return this.somePropery },
};
const target1 = {};
Object.assign(target1, obj);
const target2 = {};
Object.defineProperties(target2, Object.getOwnPropertyDescriptors(obj));
console.log(Object.getOwnPropertyDescriptors(obj));
console.log('-----------------');
console.log(target1);
console.log('+++++++++++++++++');
console.log(target2);
If you try this code in the console you’ll see that both instances are different from one another. The assign will only copy primitive values, but when using getOwnPropertyDescriptors additional information will also be copied. If this sounds theoretic and not related to anything useful, don’t sweat it.
Next time, template literals.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
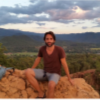
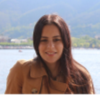
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment