MongoDB - Updating Data
In the last article we learned about more complex queries in MongoDB—not just how to search for documents with a particular value but instead to search for a range of values or for various values i.e. where both are true, or using ‘or.’ This time I’ll guide you through updating data in MongoDB.
In MongoDB, data is updated via the update method. This method alone is quite easy to use, at least at the beginning (of course we could say that about a lot of things in programming). So how do we start? With db. Don’t forget that we need to type ‘use test’ before we start to work with a database whose name is ‘test.’ And then? Our collection. If you don’t remember what it’s called, show collections will show us all of the collections in our database. OK, and then? Time for update. With this method we need to define what will be updated and how it will be updated.
In one of the previous articles we created a collection called testData:
> db.testData.find()
{ "_id" : ObjectId("541d89baefb5139de0d37abe"), "name" : "ran" }
{ "_id" : ObjectId("541d9187efb5139de0d37abf"), "name" : "Omri" }
{ "_id" : ObjectId("541d9197efb5139de0d37ac0"), "name" : "Kfir" }
{ "_id" : ObjectId("541d9197efb5139de0d37ac1"), "name" : "Daniel" }
{ "_id" : ObjectId("541d9197efb5139de0d37ac2"), "name" : "Michal" }
Let’s say we want to change the first document, from 'ran' with a little r to 'Ran' with a big R. How do we do this? First off, db.testData.update which takes two objects: $set and item. In the item object we define the conditions we want for the object and in $set we set what we're going to change. It looks like this:
db.testData.update({
"name": "ran"
}, {
$set: {
"name": "Ran"
}
})
If we run this thing, we see that ‘ran’ turned in to ‘Ran.’ Easy, no? So let’s complicate it a bit. We can, if we want to, check and see if a value exists. If it does, we can update it and if not we’ll insert it. But how? Like so:
db.testData.update({
"name": "Ran"
}, {
"name": "ran"
}, {
upsert: true
})
Here we first look for a value called ‘Ran.’ If we find it, we change it to ‘ran’, and if not we insert it.
If we want to update more than one value, nothing could be easier—all we need to do is just add multi: true to a normal update. For instance:
db.testData.update({"name":/a/},{$set:{"name":"name with a"}},{multi: true})
So what’s happening here? It’s quite simple really, we’re using update. The first condition is like any other condition, but we know it will return a few results. The $set is the same like we saw in the example above. In this instance it simply replaces the names of those values that meet the first condition (those with an ‘a’) to the string we gave. What’s special here is that we used multi: true and that’s it. Instead of updating just one document—even if there are a billion that meet the condition—it will update all documents that meet the condition.
That's all well and good, but what happens when we want to erase data? Well nothing could be easier—we just use remove when the object that we pass is just a condition.
> db.testData.remove({"name":/a/})
Notice that all document that meet this condition will be erased. If we want only one document to be erased we simply pass true, and then the document that will be erased is the first to be found.
db.testData.remove({"name":/a/},true)
If we want to erase all the documents in the collection, we can just pass a blank object.
db.testData.remove({})
And in order to completely get rid of the collection, so that when we run show collections we won’t see it, all we have to do is use:
db.testData.drop()
So that's all for now on updates! In the next guide we’ll dive a bit deeper and talk about data modeling in MongoDB, particularly when compared to relational data structures.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
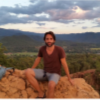
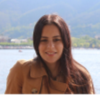
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment