MongoDB - Part 3
In this article, we will have a look at deeper MongoDB operations and build upon our previous articles. This is the third article of the series on MongoDB database tutorial, so, do check out the earlier tutorials.
Here, we will study about modifying schema of individual documents, embedded documents, Upserts & Inserts and deleting data from collections.
Advanced Operations
In this article, we will be reviewing more advanced operations we earlier did in our tutorials. To start with, we will remove fields from our existing documents. (Please note that we will be using same Students and Books collections we used in our earlier tutorials)
Remove fields from Documents
We initially thought that we will be needing book’s cover color, but we never use it. The $unset operator can be used to remove specified fields.
> db.potions.update(
{}, // Query for all books
{"$unset": {"color": ""}}, // Value we pass doesn't matter
{"multi": true} // Update all books.
)
Updating a field name with $rename
We can use $rename to change field names. So, to convert from:
{
"_id": ObjectId(...),
"name": "Harry Potter",
"score": 84,
...
}
to:
{
"_id": ObjectId(...),
"name": "Harry Potter",
"grade": 84,
...
}
All we need to do is:
> db.potions.update(
{},
{"$rename": {"score": "grade"}},
{"multi": true}
)
Now, let us consider a situation in which we need to list all the chapters in a book instead of chapter numbers. Let’s consider a magical book which contains some secret chapters. They needs to be listed now as well. So, earlier chapters looked like:
"chapters": ["hippo", "secret", "mouse feet"]
To update the secret chapter, we need to do a $set operation as shown:
> db.books.update(
{"chapters": "secret"},
{"$set": {"chapters.1": "hallow"}}
)
As clear, "chapters.1" will update the 2nd element of the array to specified value and the output will be:
WriteResult({"nMatched": 1,"nUpserted": 0,"nModified: 1})
Updating multiple array values
We need to change “secret” in multiple documents, but the location isn’t always the same for every book. In this case we can use positional parameter.
Positional Parameter
The positional operator is a placeholder that will set the proper position for the value specified in the query parameter.
> db.potions.update(
{"ingredients": "secret"},
{"$set": {"chapters.$" : "hallow}},
{"multi": true}
)
In above expression:
- The $ is a placeholder for the matched value.
- Multi is true to make the change to all documents.
- Only updates the first match per document.
Updating an Embedded value
We can update using the dot notation to specify the field we want to update. If we want to
"ratings": {
"difficult": 1,
"topics": 5
}
To update the "difficult" value, we can execute a command as:
> db.potions.update(
{"name": "Harry"},
{"$set": {"ratings.difficult" : 5}}
)
Output, as expected:
WriteResult({"nMatched": 1,"nUpserted": 0,"nModified": 1})
Useful Update operators
MongoDB provides a variety of ways to modify the values of fields.
- $max : Updates if new value is greater than current or inserts if empty.
- $min : Updates if new value is less than current or inserts if empty.
- $mul : Multiplies current eld value by speci ed value. If empty, it inserts 0.
Modifying Arrays
We’ve added categories to the books but need a way to easily manipulate the values. Right now, list of categories is:
"categories": ["scientific", "magical"]
Removing first or last value in Array
The $pop operator will remove either the first or last value of an array.
>>> Note : -1 Removes the first element and 1 Removes the last element.
> db.books.update(
{"name": "Demons & Angels"},
{"$pop": {"categories": 1}}
)
Result will be:
"categories": ["scientific"]
Adding values to end of Array
The $push operator will add a value to the end of an array.
> db.potions.update(
{"name": "Demons & Angels"},
{"$push": {"categories": "thriller"}}
)
Result will be:
"categories": ["scientific", “thriller”]
Adding unique values to Array
The $addToSet operator will add a value to the end of an array unless it is already present.
> db.potions.update(
{"name": "Demons & Angels"},
{"$addToSet": {"categories": "thriller"}}
)
Value already exists, so it doesn’t get added again. Result will be the same:
"categories": ["scientific", “thriller”]
Removing values from an Array
The $pull operator will remove any instance of a value from an array.
> db.potions.update(
{"name": "Demons & Angels"},
{"$pull": {"categories": "scientific"}})
Result will be:
"categories": [“thriller”]
Filters and Queries in MongoDB
We’ve received a new feature request to allow users to filter books based on multiple criteria.For example, only show books written by Hillary that have a difficulty of 5.
Querying on multiple criteria
We can query based on multiple criteria by passing in comma-separated queries.
We can pass in more than 1 query, as shown:
> db.books.find(
{"author": "Hillary",
"ratings.difficulty": 5}
)
Queries of equality are great, but sometimes we’ll need to query based on conditions like “Search for books with a price less than 200”.
We can use comparison query operators to match documents based on the comparison of a specified value. Common comparison operators are:
- $gt : greater than
- $gte : greater than equal
- $ne : not equal to
- $lt : less than
- $lte : less than or equal to
We can match the appropriate documents by using the $lt comparison operator for the above example,
> db.books.find({"price": {"$lt": 200}})
Did you see that? It was as simple as that.
Finding books within a range
We can query with a range by combining comparison operators. This means, just use a combination of operators and get the results. Let’s look at an example by combining operators,
> db.books.find({"price": {"$gt":100, "$lt": 200}})
Above command filters all books with a price less than 200 and greater than 100.
Range queries on an Array
Each book has a stock field that contains an array of available books. We can use $elemMatch to make sure at least 1 element matches all criteria.
Let’s workout a quick example to filter books based on stocks:
> db.books.find(
{"stock" : {"$elemMatch": {"$gt": 1, "$lt": 10}}}
)
We need to be careful with Array filters. Each value in the array is checked individually. If at least 1 array value is true for each criteria, the entire document matches.
Conclusion
In this article, we took a look at deeper MongoDB operations and built upon our previous articles. This was the third article of the series on MongoDB database tutorial.
In the next article of the series, we will deep dive in using comparison operators and dangers of replication in MongoDB.
Recent Stories
Top DiscoverSDK Experts
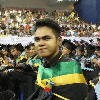
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment