MongoDB - Data Retrieval: part 1
In the last article we created a collection, a database, and even a document. We also saw how each document in Mongo is basically a JSON object and that we need to create it before we put it into the database. It’s pretty easy to create a document, but the question remains as to how we retrieve data.
The base of the query structure is find. Don’t forget that we need to “use” the database—that is to say, I’ll assume you’ve already done use test (if test is the name of your database). After this we need to type the name of our collection. Perhaps you forgot the name of your collection? Using show collections will show you all of your collections. Find is the method we’ll use. If we don’t use an argument then we’re just using a general query that will return everything—pretty similar to SELECT * that you’re probably familiar with.
Confused? Try this:
db.”testData.find()
This line will return all of the documents in the collection named testData. It will look something like this:
{ "_id" : ObjectId("541d89baefb5139de0d37abe"), "name" : "ran" }
Hold on a minute there! What’s that “_id”? We didn’t give an _id when we created the document, right? So where did it come from? MongoDB gives it a name automatically. We’ll get to this later on—for now just don’t be alarmed when you see it. If we enter in more data, we’ll see all of it when we use find. So let’s practice! The following query puts in a few documents with names to a collection called testData:
db.testData.insert([{"name":"Omri"},{"name":"Kfir"},{"name":"Daniel"},{"name":"Michal"}])
Those of you who know a little Javascript and JSON won’t be confused by the syntax. It’s just a collection of objects that go into the insert method as an argument. If it seems a bit confusing, just have a closer look at the code for a bit and you’ll understand—instead of putting the object inside of a variable and then putting the variable inside the insert method, like in the last article, we simple created a few objects and put them right into insert. Our database now looks like this:
> db.testData.find()
{ "_id" : ObjectId("541d89baefb5139de0d37abe"), "name" : "ran" }
{ "_id" : ObjectId("541d9187efb5139de0d37abf"), "name" : "Omri" }
{ "_id" : ObjectId("541d9197efb5139de0d37ac0"), "name" : "Kfir" }
{ "_id" : ObjectId("541d9197efb5139de0d37ac1"), "name" : "Daniel" }
{ "_id" : ObjectId("541d9197efb5139de0d37ac2"), "name" : "Michal" }
Let’s assume we want to search for the user named Kfir. All we need to do is use find to pass an object with whatever we want to be returned. If we want to receive all of the objects with the name Kfir, we’ll search for:
db.testData.find({"name" : "Kfir"})
In this instance I’ll receive just one of course.
We can also pass more sophisticated things as arguments to the find method. Let’s say we want to return all the names that have an “r” in them. In MySQL with have the LIKE command. But in MongoDB we can use regular expression. For example:
db.testData.find({name : /r/})
This returns all the documents with the letter r. If we want all of the name that start with r:
db.testData.find({name : /^r/})
And if we want all the names that end with r:
> db.testData.find({name : /r$/})
Obviously we can use RegEx that is more complicated than just one word. But of course we can also search for more complicated things using LIKE, just as we would in MySQL.
So let’s make a collection that’s a bit more sophisticated. As stated previously, the MongoDB shell behaves just like in JavaScript. This means we can define functions. Here we’ll define a function that creates a random string of characters:
function randomString() {
var chars = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXTZabcdefghiklmnopqrstuvwxyz";
var randomstring = '';
var string_length = 100 + Math.floor(Math.random() * 200);
for (var i = 0; i < string_length; i++) {
var rnum = Math.floor(Math.random() * chars.length);
randomstring += chars.substring(rnum, rnum + 1);
}
return randomstring;
}
Take this whole thing (I know, it’s long) and paste it into the console. From this point on, until you exit, you’ll be able to call the randomString function. Now let’s create a collection called clients like so:
for (var i = 0; i < 100; i++) {
db.clients.insert({
x: i,
name: randomString(),
value: _rand() * 100
});
}
If you know some JavaScript, this is pretty simple—a simple loop that creates a document that has x, a name, and a value. Running this things yields a collection called clients that contains 100 documents—something along these lines:
{ "_id" : ObjectId("541d9aee784269c2e9f3c092"), "x" : 17, "name" : "gIZxF4ALvwiMMtvtZC8G6ExsmxQum8KFAQws4z6un9nMrol8QCCue7T7rCLVvnNM0dfVzW3VKZgEeS5PLKA6RNI7lZbWT1ySl8v1xb77nmCasF3I8Vr5Vu3Bqa0uDv8umXkbreihE1DXl2OrudxEmUPMf0PKSdQhHe5zqqQ90LOBBwd6lNBGhmeLkI1ramDC4i7JnnfmTrX8v61e", "value" : 34.991921903565526 }
{ "_id" : ObjectId("541d9aee784269c2e9f3c093"), "x" : 18, "name" : "e683H4QlB2sHLGUtvnftDpWQTJJgZ2Ev92TRTEK5oiCbLxsz2d5tS0hx4fKZqzfITMrtlDJkI4R2RRMhvT4wngOulcTnb9t8SnfDytSIplJUhly", "value" : 25.878882687538862 }
{ "_id" : ObjectId("541d9aee784269c2e9f3c094"), "x" : 19, "name" : "HIIWTKsT8guLtyMkgf3KVmCzJNUviArxVT9GaT4vI3Bkg3AZLWzMoMGVynoQz1qp0elVk0oyxdx7fUUFFRWNRtUJipf95WhKGQu3UDkRb2XFigmuhGnVc5WNbCg1w", "value" : 96.42805075272918 }
With this we can now practice a few queries. Let’s say we want everything with a value above 95—how do we do it? We’ll use the find method from our collection, which you should already be familiar with. But which argument do we need to pass? What will the object look like? It goes like this:
db.clients.find({value: {$gt:95}})
The gt stands for greater than, which we use in this case for everything with a value greater than 95.
More on collections in the next article.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
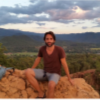
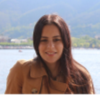
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment