Job Schedulers in Android
If you have some heavy work to do and it doesn’t need to be done right now, than using JobScheduler API will be a very wise choice for your apps and more importantly, your app users.
Basics
JobScheduler API was introduced in Android Lollipop and it guarantees to get your work done but since it operates at system level, it can use several factors to intelligently schedule your background work to run with the jobs scheduled from other apps as well and that means we can minimise things like radio use, which is a clear battery win!
WIth JobScheduler API,
- Work isn’t done on a time schedule but when the right conditions are met. This means, you can actually set conditions for your Job scheduler.
- Runs on the main thread.
With the API 24, JobScheduler even considers the memory pressure which is overall a clear win all the apps and their users.
Cool JobScheduler API
Right now, there are two ways to schedule a task in Android. They are :
- AlarmManager API (almost outdated).
- JobScheduler API.
JobScheduler API is very cool because it doesn’t work solely based on time, but rather based on conditions. This API allows to batch jobs when the device has more resources available. You can input a signal or a trigger to define the condition you want to wait for like:
- Set jobs to be done when a user is on metered vs an unmetered connection like a Wifi.
- You can also use it for jobs to be persisted that you want to persist a potential reboot of the Android device.
- With the latest updates, you can even schedule jobs based on update to a Content provider.
All of the conditions can be defined when you’re actually creating a job. For example, to create a Job, you create a JobInfo object as shown below:
JobInfo discoverSdkJobScheduler = new JobInfo.Builder(jobNumber, jobService)
//we care about battery
.setRequiresCharging(true)
.setRequiredNetworkType(JobInfo.NETWORK_TYPE_UNMETERED)
.build();
In the above code, we create a JobInfo object and set two constraints that this job should only run when the device is plugged in and is connected to an unmetered connection.
While creating a JobInfo object, there are two things you will need every time:
- A job number to help you distinguish which this is.
- A job service which is actually going to be a class which extends the JobService class where you define the work you’ll be doing when your Job actually runs.
As clear when I said to extend another class, JobService class, you will need to implement a few methods as well. A simple JobService class will look like this:
import android.app.job.JobParameters;
import android.app.job.JobService;
public class DiscoverJobService extends JobService {
@Override
public boolean onStartJob(JobParameters jobParameters) {
return false;
}
@Override
public boolean onStopJob(JobParameters jobParameters) {
return false;
}
}
- onStartJob() is called by the system when it is time for your Job to execute. This method executes on the MAIN THREAD. Now, this is tricky. As it is the MAIN THREAD, use this method to either perform a simple (and short) task or just kickoff a background thread (like an AsyncTask) for complicated work.
- If you use a background thread, you’ll need to return TRUE.
- If you don’t use a background thread and it is performing the task in the onStartJob() itself, go ahead and return FALSE.
- Return value is just to let the system know if there is any ongoing work still.
- onStopJob() is called by the system when if your job is CANCELLED before being finished, perhaps the conditions are no longer being met like the device has been unplugged. So, use this for safety checks and cleanup and then return TRUE if you would like the system to reschedule your job or FALSE if it doesn’t matter and the job will be dropped.
- Now there is another method you don’t override. jobFinished() is a method you won’t override and the system won’t call it, and that’s because you need to call it once your service has finished working on the job. This is how the system knows that your job was really done and it can release your WakeLock.
This is important, if you forgot to call jobFinished(), your app is going to look very guilty in the battery stats lineup. jobFinished() methods take 2 parameters:
- Job Number : This is so the system knows which job we’re talking about.
- needsReschedule (boolean): True if this job should be rescheduled according to the back-off criteria specified at schedule-time. False otherwise.
With any service, you will need to define your JobService in the Manifest.
<service android:name=".DiscoverJobService"
android:permission="android.permission.BIND_JOB_SCHEDULER" >
</service>
What’s different though is that you need to add a permission that will allow the JobScheduler to call your jobs and be the only one that can access your JobService. Finally, you need to add your job to JobScheduler queue, like shown:
JobScheduler discover = this.getSystemService(JobScheduler.class);
discover.schedule(discoverSdkJobScheduler);
Batching job execution in the above shown fashion allows the device to enter and stay in sleep states longer and so, preserving battery life for a longer time.
Not so scary, is that?
AlarmManager vs JobScheduler
You should only use AlarmManager API for tasks that must be executed at a specific time, but do not require the other, more robust, execution conditions that JobScheduler allows you to specify, such as device idle and charging detect.
While AlarmManager is critical system service that runs all the time. And if your application scheduled something and was killed, then AlarmManager may start application again (via PendingIntent).
JobScheduler is way easier than setting up a SyncAdapter and much more flexible than the AlarmManager API!
Conclusion
In this tutorial, we had a smart look at the JobScheduler API. Though there are several pieces to be sure of, and you’ll need to think carefully about when and what should trigger your job and what should happen if it fails for some reason. But overall, JobScheduler was designed to be easy to work with.
So give it a try and build better apps.
Recent Stories
Top DiscoverSDK Experts
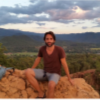
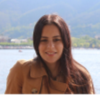
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment