JavaScript ES2020 — globalThis
The new ES2020 standard contains several important additions to JavaScript. One of the more interesting additions, which also happens to be one of the easiest to understand, is called globalThis. Sometimes, especially in test scripts (but not only!), we need to access the global scope. What’s the global scope you ask? Think of it as the highest scope in the program. If we’re writing JavaScript for a browser environment, in most cases this will be our window or the self. If we’re writing JavaScript in a Node.js environment, it could be this or global—depending.
Things would get complicated when writing extensions (e.g. for chrome). Many have tried to gain access to a global this by doing something like … this:
(new Function("return this")())
But if a site has a CSP Header that prevents eval (which most sites do) then this solution won’t work because of that eval. Then, we need to do something quite interesting if we want to understand what the global object is:
function foo() {
// If we're in a browser, the global namespace is named 'window'. If we're
// in node, it's named 'global'. If we're in a shell, 'this' might work.
(typeof window !== "undefined"
? window
: (typeof process === 'object' &&
typeof require === 'function' &&
typeof global === 'object')
? global
: this);
}
So like, is this code that we’re actually supposed to use?
In principle, using the global scope is usually a bad idea. There are a few things we need to be careful of so as not to contaminate the scope. The first is clashes. If we set a variable in the global scope anyone can easily overwrite it—even without knowing it. But we often do end up working with a global scope, or with natural methods in the environment where JavaScript runs (setinterval, or window.location for example).
A lot of programmers use this without even knowing it. For example, you could use setInterval instead of window.setInterval (in a browser environment) or global.setInterval (in Node.js). And this probably works, right up until it doesn’t because they’re running the code in a different environment. What do I mean by “different environment?”
Take for example automated testing where the environment can change (like in an automated test on a client-side app that runs in the browser but that we’re running from the terminal). Or code that’s a Node.js module and we want it to run in the browser.
In cases like these, where we use methods or variables that come from the global scope, it’s good practice to use globalThis that simply return the global object, whether we’re talking about window, self, or global. Using globalThis is easy as can be. Wherever we put window (in the browser) we place globalThis. Here’s an example:
globalThis.myVariable = 'Hi, this is your friendly global variable';
globalThis.document.querySelector('body').innerText = globalThis.myVariable;
It’s so straightforward that there’s really not much else to say. Here’s a codepen:
It’s really a good idea to use this every time you need to access the global scope. I wouldn’t go wild and start putting it everywhere, but in automated tests where you need to use emulation, it’s a great thing. So if you’re writing code and you need to access a global object, globalThis is the best way to do it in 2020.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen
Recent Stories
Top DiscoverSDK Experts
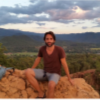
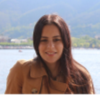
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment