Introduction To Generic Programming With Java
Object oriented programming languages are improving day by day and coding looks more like the work of humans than the work of machines. Somewhere in the unstoppable journey of improving the way we program, generic programming was invented. Generic programming helps us to not repeat code that looks the same except for some data types. We the humans are lazy to write more, we always want to have more work done with less code. Generic programming is a style of programming in which you can have more work done by writing less. Repeating the similar sections of code or copy/pasting them over and over again is a pain and helps bugs to creep into your program. Generic programming can help you get out of such nightmares.
Enough talk! Now, we going to move to the discussion of generic programming in java. Without just sticking to theories I would like to show you problems and solutions to them and in that way I would like to introduce to the world of generic programming in java.
Setting Up
To get started with coding shown in this article you need to have latest JDK installed on your system. You are free to use any code editor or IDE you like. I am going to use IntelliJ IDEA from JetBrains. Create a class called JavaGeneric with main method inside your project. Our initial code should look like the following:
public class JavaGeneric {
public static void main(String[] args){
}
}
The Problem
Let's talk about a common problems and find solutions to them with the help of generic programming. Except for the character array we cannot get a our desired output with other types of arrays from System.out.println(). Let's see the proof.
public class JavaGeneric {
public static void main(String[] args){
System.out.println(new char[]{'a', 'b', 'c', 'd'});
System.out.println(new int[]{1, 2, 3, 4});
}
}
Outputs:
abcd
[I@4554617c
See? It can give our desired output when we put a character array, but it fails to provide a pretty output for an integer array.
We need to create a method that will be able to print all types of arrays. For now, let's say we want to create a method that will be able to print an array of character, integer, double, string in our desired format. To make a common method for all of them we can create overloaded methods with the same name. Let's name the method as printArray(). Here's how the method should look like.
public class JavaGeneric {
public static void printArray(char[] cr){
String out = "";
for(char ch : cr){
out += ch + ", ";
}
System.out.println(out);
}
public static void printArray(int[] ir){
String out = "";
for(int i :
ir){
out += i + ", ";
}
System.out.println(out);
}
public static void printArray(double[] dr){
String out = "";
for(double d : dr){
out += d + ", ";
}
System.out.println(out);
}
public static void printArray(String[] sr){
String out = "";
for(String s : sr){
out += s + ", ";
}
System.out.println(out);
}
public static void main(String[] args){
char[] cr = {'a', 'b', 'c', 'd'};
int[] ir = {1, 2, 3, 4};
double[] dr = {1.2, 2.3, 3.4};
String[] sr = {"Str 1", "Str 2", "Str 3"};
printArray(cr);
printArray(ir);
printArray(dr);
printArray(sr);
}
}
Output:
a, b, c, d,
1, 2, 3, 4,
1.2, 2.3, 3.4,
Str 1, Str 2, Str 3,
But that is a whole lot of code and the same pattern of code is repeated throughout the methods.
The Solution
We the programmers are lazy, but we are intelligent lazy people. We need a better way to do it. We also hate copy/paste. So, let's do the same with generics in Java.
Warning: generic programming in Java does not allow for primitive data types. But that is not a big deal. We have equivalent classes for boxing primitive data types, i.e. Integer, Double, Character, etc.
public class JavaGeneric {
public static <T> void printArray(T[] arr){
String out = "";
for(T e : arr){
out += e + ", ";
}
System.out.println(out);
}
public static void main(String[] args){
Character[] cr = {'a', 'b', 'c', 'd'};
Integer[] ir = {1, 2, 3, 4};
Double[] dr = {1.2, 2.3, 3.4};
String[] sr = {"Str 1", "Str 2", "Str 3"};
printArray(cr);
printArray(ir);
printArray(dr);
printArray(sr);
}
}
The output is the same as before, but we have written one quarter of the previous code. This is the magic of generic programming. There was always a comma after the list of elements and I did not remove it because I was lazy to fix that for all four overloaded methods. But now I can fix it without much effort.
public class JavaGeneric {
public static <T> void printArray(T[] arr){
String out = "";
int count = 0;
for(T e : arr){
count++;
if (count == arr.length){
out += e;
}else{
out += e + ", ";
}
}
System.out.println(out);
}
public static void main(String[] args){
Character[] cr = {'a', 'b', 'c', 'd'};
Integer[] ir = {1, 2, 3, 4};
Double[] dr = {1.2, 2.3, 3.4};
String[] sr = {"Str 1", "Str 2", "Str 3"};
printArray(cr);
printArray(ir);
printArray(dr);
printArray(sr);
}
}
Outputs:
a, b, c, d
1, 2, 3, 4
1.2, 2.3, 3.4
Str 1, Str 2, Str 3
Perfect!
Explanation
In the previous block of code we see a new strange syntax. That is . We call <> the diamond operator. It is used to specify an arbitrary type in our code. It can be applied on the whole class or a specific method during declaration. An identifier is used inside of it. It is advised to use a single uppercase character as the arbitrary type specifier.
The type specified inside the diamond operator is not determined before using that method. In the main method when we invoked printArray() with cr as the argument, the compiler replaced that T with Character everywhere that T occurred in the method. Same happened for printArray(ir), printArray(dr) and printArray(sr) according to the array type.
Generic Method
We already covered generic method in the above sections. A generic method is the method that has a generic type(s) declared in with the method signature. The scope of the generic type is only that specific method. That means in the above code the T will only be available to printArray() method. You may create another method and put another with it, but that will be a different T.
Generic Class
A generic class is a class that has a generic type(s) declared during the class declaration. The scope of the type(s) is the whole class—that means, every method in that class will have access to the specific generic type(s).
Let's create a generic class.
class GenClass <T>{
public void dummyMethod(T e){
System.out.println();
}
}
Notice, the generic type T is now accessible from a method called dummyMethod() inside that class. That means, every method in the class can access that same generic type.
Conclusion
Generic programming in Java is a large topic and I have only introduced you to the very basics in this article. In future articles we’ll go into more detail and cover more advanced topics. In the meantime, keep practicing and making yourself comfortable with the concept of generic programming.
For more Java, check out our guide to concurrency in Java and stop by the homepage to find the perfect software component for your next project.
Recent Stories
Top DiscoverSDK Experts
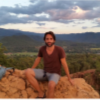
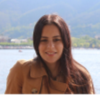
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment