Introduction to for-of loops in ES6
ES6 fulfilled many demands of JavaScript programmers and also surprised us with a lot of cool features. The for-of loop is one of those features that made coding in JavaScript more fun. JavaScript already had for, for-in and while loops. So, why would another loop be needed? What extra benefits does it provide and how do we use it? I will provide you all the details in this article.
Before we begin, I want to remind you again that I prefer using NodeJs for writing and practicing JavaScript code. JavaScript can be run seamlessly on browsers, NodeJs and other JavaScript engines in the same way unless you use any platform specific API. When you do not need any specific browser, NodeJs or other API, you should use NodeJs for coding. I explained why this is a good thing in my first article on JavaScript on this blog. ES6 code cannot directly run on older versions of browsers and NodeJs environment, if you intend to use JavaScript on those old environments you have to use Babel or other transpilers to transpile ES6 code to ES5 or below.
What is a for-of Loop and How to Use It
for-of is a special loop that loops over iterable objects like arrays, maps, sets, strings, etc. So, basically it is an iterator loop. It has a very simple syntax:
for (variable of iterable) {
statements
}
On each iteration over the iterable a value will be assigned to the variable. Let's say we have an array of numbers that we want to iterate over and print each number on the screen.
var arr = [1, 3, 5, 7];
for (let x of arr){
console.log(x);
}
Running the code will provide the following output:
1
3
5
7
A string is also an iterable object. Let's try to print every character of a string with for-of.
var st = "abcd efg";
for (let c of st){
console.log(c);
}
Outputs:
a
b
c
d
e
f
g
Let's try to iterate over a string that contains an 'a' and the unicode escape for closed umbrella:
var ustr = "a\ud83c\udf02";
for (let c of ustr){
console.log(c);
}
The result will be two characters, 'a' and the closed umbrella emoji.
Outputs:
a
🌂
Notice that it did not consider '\ud83c\udf02' as two separate characters. As it is a single character it was treated as a single character by the for-of loop. In another section you will see that with for-in and indexing the behavior will not be the same.
Maps are iterable objects and I want to try with them too. Let's have another example:
let a_map = new Map()
a_map.set('x', 10);
a_map.set('y', 9);
a_map.set('z', 0);
for (let pair of a_map) {
console.log(pair);
}
Outputs:
[ 'x', 10 ]
[ 'y', 9 ]
[ 'z', 0 ]
Later you will see that for-in does not work for maps and other new data structures.
Controlling for-of
Like other loops for-of can be tamed with break and continue. Using the break keyword will exit the loop and the continue keyword will continue the loop skipping whatever is below it. Let's try with an example:
var arr = [1, 3, 2, 5, 7, 9];
for (let x of arr){
if (x % 2 == 0){
continue;
}
if (x == 7){
break;
}
console.log(x);
}
Above code provides the following output:
1
3
5
In our code we wanted to skip even numbers and thus we used continue to carry out the operation that follows. That's why 2 was skipped and not printed, instead 5 was printed. Again, we told our loop to stop whenever it encounters 7. In our array 7 is before 9 and was not printed.
for-in vs for-of
for-in and for-of might look the same at the first glance. Concentrate on your result more to see the difference. Again, for-in is used only for enumerable objects and for-of is used for iterable objects. for-in does not provide a value, instead it provides the key or the index of the value we want to use. Let's try out the first example from the above section:
var arr = [1, 3, 5, 7];
for (let x in arr){
console.log(x);
}
Running the above code will provide the following output:
0
1
2
3
So, it provided the indexes only. To get the value we have to do extra work:
var arr = [1, 3, 5, 7];
for (let x in arr){
console.log(arr[x]);
}
Outputs:
1
3
5
7
Looping over a string will also provide the character indexes instead of the character itself. So far so good. Let's try our emoji example with a for-in loop and character indexing.
var ustr = "a\ud83c\udf02";
for (let c in ustr){
console.log(c);
}
It outputs:
0
1
2
That means for-in is considering the ustr as a collection of three characters instead of two unlike a for-of iterator loop.
Let's see what happens if we console.log() them by retrieving the characters with indexing:
var ustr = "a\ud83c\udf02";
for (let c in ustr){
console.log(ustr[c]);
}
Outputs:
a
�
�
That's not good! So, for-of is the winner for such tasks.
After this bad result let's try for-in with maps as we did for for-of:
let a_map = new Map()
a_map.set('x', 10);
a_map.set('y', 9);
a_map.set('z', 0);
for(let pair in a_map){
console.log(pair);
}
No result! So, for-in is not for new things. It will rot there with other old stuff in the world of JavaScript. The new day is for for-of only.
Conclusion
for-of loop is a new and powerful addition to JavaScript and with its help we can avoid various creepy behavior in JavaScript. For programmers who have JavaScript experience from before ES6 and are new to it, may easily confuse the for-in and for-of loop. So, beginner ES6 programmers or brand new JavaScript programmers should be careful of these things and avoid possible bugs in their JavaScript programs.
Recent Stories
Top DiscoverSDK Experts
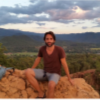
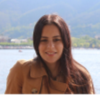
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment