Functions in TypeScript — Part 2
Welcome back to functions in TypeScript. Let’s jump right back into it!
Anonymous Functions
Anonymous functions in TypeScript are those that are not bound to an identifier i.e. a function name. Instead, they are declared dynamically at runtime. TypeScript anonymous functions can receive inputs and return outputs just like any other functions can. But anonymous functions usually are not accessible once they’ve initially been created.
When a variable is assigned an anonymous function, we call this type of expression a function expression. The syntax is like this:
var res = function( [arguments] ) { ... }
Here’s an example of a simple anonymous function:
var msg = function () {
return "DiscoverSDK.com";
}
console.log(msg())
This will simply print out our message to the console:
DiscoverSDK.com
Now have a look at an example of an anonymous function with parameters:
var res = function(a:number,b:number) {
return a*b;
};
console.log(res(15,5))
This anonymous function returns the product of the values that we passed to it.
The JavaScript after compiling is actually the same code. In the console you should see:
75
Function Expression vs Function Declaration: What’s the difference?
Function expression and function declaration are actually not the same. As opposed to function expressions, function declarations are bound by the function name.
The major difference between them is that function declarations are parsed before their execution. However, function expressions are only parsed once the script engine comes across them during execution.
If the JavaScript parser finds a function in the main code flow, it assumes it to be a function declaration. But, if a function comes as a part of a statement, then it’s a function expression.
The Function Constructor
TypeScript allows us to define a function with a built-in JavaScript constructor called Function(). This syntax looks like this:
var res = new Function( [arguments] ) { ... }.
Let’s look at a quick example:
var myFunction = new Function("x", "y", "return x * y");
var x = myFunction(10, 5);
console.log(x);
This new Function() is a call to the constructor. As a result of this, a function reference is created and returned.
The JavaScript is the same ad the output should be:
50
Recursion in TypeScript Functions
Recursion doesn’t have to be as scary as some people make it out to be. Really, it’s just a way to iterate over an operation by having a function call itself repeatedly until it arrives at a certain result. One of the best uses of recursion is when you need to call the same function repeatedly with different parameters from inside a loop. Here’s an example:
function factorial(number) {
if (number <= 0) { // the termination case
return 1;
}
else {
return (number * factorial(number - 1)); // the function invokes itself
}
}
;
console.log(factorial(5)); // outputs 120
This code will output:
120
Here’s another example of an anonymous function with recursion:
(function () {
var x = "DiscoverSDK!";
console.log(x)
})() // the function invokes itself using a pair of parentheses ()
The output will be:
DiscoverSDK!
Lambda Functions in TypeScript
You may be familiar with Lambda functions since they’re also known as arrow functions. They are a concise way to represent anonymous functions. We can break them down into three components:
- Parameters − A function may optionally have them
- The lambda notation (=>) − Also known as the ‘goes to’ operator
- Statements − these represent the function’s instruction set
It’s common to use a single letter parameter for a concise and precise function declaration.
TypeScript Lambda Expression
This is an anonymous function expression that points to a single line of code. The syntax looks like this:
( [param1, parma2,…param n] )=>statement;
Here’s an example:
var foo = (x:number)=>15 + x
console.log(foo(50)) //outputs 65
The code declares a lambda expression function. The function returns the sum of 15 and the argument we pass it.
The output after compiling should be:
65
Lambda Statements in TypeScript
Lambda statements are anonymous function declarations which point to a certain block of code. We use this syntax when the function spans multiple lines. The syntax looks like this:
( [param1, parma2,…param n] )=> {
//code block
}
Here’s our TypeScript lambda statement example:
var foo = (x:number)=> {
x = 15 + x
console.log(x)
}
foo(85)
We return the function’s reference and store it in the variable foo.
Upon compiling, the following JavaScript code will be generated:
"use strict";
var foo = (x) => {
x = 15 + x;
console.log(x);
};
foo(85);
And the output to the console should be:
100
Variations in Syntax
Parameter Type Inference
You don’t have to specify a parameter’s data type. If you don’t, then the data type will have a default parameter of any. Have a look at this code example:
var func = (x)=> {
if(typeof x=="number") {
console.log(x+" is a number")
} else if(typeof x=="string") {
console.log(x+" is a string")
}
}
func(15)
func("Arjun")
This will generate the following JavaScript:
"use strict";
var func = (x) => {
if (typeof x == "number") {
console.log(x + " is a number");
}
else if (typeof x == "string") {
console.log(x + " is a string");
}
};
func(15);
func("Arjun");
In the console you should see:
15 is a number
Arjun is a string
Optional parentheses for a single parameter
Here’s another example of a different syntax:
var display = x=> {
console.log("The function received "+x)
}
display(25)
Here’s the JavaScript:
"use strict";
var display = x => {
console.log("The function received " + x);
};
display(25);
And the output to the console:
The function received 25
That’s all for this time. Check back soon for the next article in the series where we’ll look at some number methods. See you then!
Next article: coming soon! |
Recent Stories
Top DiscoverSDK Experts
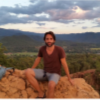
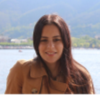
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment