ES2020 — Dynamic Import
A really cool new feature that’s part of the new JavaScript standard, ES2020, is dynamic import, which we can use with lazy loading (if you’re into that sort of thing).
But first off, let’s get things going with a very brief explanation of regular import. Standard JavaScript code is typically divided into various modules. These modules can be classes or functions, but at the end of the day we need to organize our code into sections. Each file is generally organized as a module that has an export. So for example, if I want to create a particular module, I’ll create it with export:
export default class MyModule {
constructor() {
this.myText = 'Hello World!';
}
sayHello() {
console.log(this.myText);
}
}
Then I can use it by doing something like this:
import MyModule from ('/path/to/MyModule.js');
const myModule = new MyModule;
myModule.sayHello();
This way, I can organize my code into several different files. There are of course a few different ways to do import/export, but in general it looks like that. So what’s the problem with this type of import? In short, it’s static. It’s found at the top of the file and is loaded by default. If you try to use it as a condition, you’ll get an error:
SyntaxError: ... 'import' and 'export' may only appear at the top level.
What that problem? If I want to delay the loading and have it be dependant upon an action taken by the user, I can’t do it. This delayed loading is known as lazy loading—loading that happens in accordance with a user action and prevents a long load time. If we want to use lazy loading in the front end, we’d need to use webpack to do so. That was the only solution, until ES2020 came along.
The new JavaScript standard allows us to use import as a function in any piece or part of our code. We’re talking about an asynchronous function that returns a promise. If you don’t know what a promise is, check out my article about promises in JavaScript here.
Now, we can use import in a variety of ways. First, we can just pass the name of the file that we want to pass to it. Then, it’s just as if we used script src. Ah, but wait—it will only load when we want it to. For instance, in this example the import only happens when the button is clicked on.
If there’s an export that we want to receive, here’s what the promise returns. For instance, this example from MDN:
let module = await import('/modules/my-module.js');
We can use async await’s syntax with a regular promise. For instance, if we take our example module that I mentioned earlier, something like this:
import('/path/to/MyModule.js')
.then((MyModule) => {
const myModule = new MyModule;
myModule.sayHello();
});
For the die-hard webpack users out there, this may look like the most useless thing ever. But still, this is a fantastic and important feature that’s now in the new JavaScript standard. For those writing simple scripts, it will make it easier to keep their modules organized and to use lazy loading in a more elegant way.
It’s not a complicated feature, but it is an important one.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
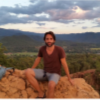
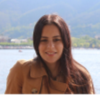
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment