Enums In Java Explained
In the beginning of computer programming there were binary numbers to provide machines (computers) with instructions. Genius computer programmers devised another mechanism to code. They assigned symbols, characters, words for each set of binary instructions making life a lot easier for programmers. But that was just the beginning and he human being has insatiable taste for perfection.
The language that carried out its tasks with symbols instead of binary numbers was called assembly language. But it was still hard to create large programs with it. In the search for perfection, more and more languages started to come into life and each with with new and interesting features. At some point in time this progressed into the creation of Object Oriented Programming languages. In the modern age Java is one of the most popular object oriented programming languages.
Since the beginning of the computer programming, programmers tried their best to make programming more intuitive. We’ve tried to to code more like humans and less like machines. OOP languages relieved us of various pains of coding like a machine and helped us think of code in earthly manner and we relate parts of our code to various earthly things.
Enum or Enumeration is one of those things that helped us think and act more like humans and less like machines while coding. When coding we tend to create constant variables of numbers to identify different things in a category. For example, if I tell you to represent months of the year in code, the first thing that will come to your mind is putting the month names as strings inside an array.
{"January", "February", "March", ...}
Now, if those month names are needed to be referenced inside the code repeatedly, we tend to keep them inside constant variables as shown below.
final String JANUARY = "January";
...
And often we assign integers instead of strings, because integers or numbers are easier to compare.
final int JANUARY = 1;
final int FEBRUARY = 2;
...
But there are some problems even if you are going to use the last method! What if you mistakenly put the same integer for two different variables? What if you compare any other integer that is not in the range that you used for indicating months? What if you compare the NORTH variable with JANUARY, where NORTH is used for direction and also of the type integer? A lot of other bad things can happen. In the end it will be very hard to hunt down the bug in your program.
Getting Started
To get started with code shown in this article you need to have JVM installed on your system. You can choose any code editor or IDE of your choice. I am going to use IntelliJ IDEA from JetBrains. Create a project and create a class called JavaEnum with a main method inside of it. So, our initial code should look like the following:
public class JavaEnum {
public static void main(String[] args){
// Our code starts here
}
}
Enum Is The Savior
The best way discussed above is the way of constant variables of integers or other types of numbers. But that is not a good way as you can see. We need something more intuitive and most important of all, something less error prone.
Enum or enumeration in Java is like class—that means it is a type. It acts as a set of things of a certain kind. It is type safe and every element in the set can be created like a variable. So, all the elements in the set are the values and you do not need to assign any other value to them. It is the most perfect and intuitive way of working with such things. Let's clarify with examples. Let's say that you want to represent four directions: north, south, east, and west. We have to create enum for it. An enum is created the same way as a class. Instead of the class keyword though, we use enum keyword. Create a file named EDirection.java and create an enum called EDirection. I am using an additional E to indicate it as an enum (this extra E has nothing to do with Java, it's a personal choice). So, the code in the file looks like the following:
public enum EDirection {
EAST,
WEST,
NORTH,
SOUTH
}
You have to use comma as a separator between the elements of the enum. If you have other methods defined inside the enum then you have to use a semicolon at the end of the last element.
Let's write some code with the enum now.
public class JavaEnum {
public static void main(String[] args){
// Declare a variable of the EDirection enum type
EDirection dir = EDirection.EAST;
if (dir == EDirection.EAST){
System.out.println("You are going in the right direction. It's EAST you are going");
} else if(dir == EDirection.WEST){
System.out.println("Wrong direction!");
}else if(dir == EDirection.NORTH){
System.out.println("Wrong direction!");
}else {
System.out.println("Wrong direction!");
}
}
}
Hit build and run to see the following output:
You are going in the right direction. It's EAST you are going
See: Now, we have our own type called EDirection. Previously we used type of int or String and that is not an specific type of our need. The height of type safety is really very high now.
Enum Is Special Kind Of Class
Enum in Java is more powerful than the enum found in any other languages. Actually an enum is a special kind of class. An enum can have constructor and other methods too. But remember, an enum cannot be instantiated with a new keyword. It is safe to say that enums are a kind of singleton. Each member inside the enum is an instance of that enum. So if we create a constructor, that constructor will be used to provide data to each member of the enum. That also means that we have to pass a value to each member of the enum during creation. Let's understand it with code.
public enum EDirection {
EAST("East direction"),
WEST("West direction"),
NORTH("North direction"),
SOUTH("South direction");
private String txt;
EDirection(String txt){
this.txt = txt;
}
public String toString(){
return this.txt;
}
}
In the main class:
public class JavaEnum {
public static void main(String[] args){
System.out.println(EDirection.EAST);
System.out.println(EDirection.WEST);
System.out.println(EDirection.NORTH);
System.out.println(EDirection.SOUTH);
}
}
Outputs:
East direction
West direction
North direction
South direction
Iterating Over Emun
We can iterate over the members of the enum. A values() method is automatically created for every enum in Java. Let's try to prove it with a foreach loop in Java. Use values() method on the enum to iterate over it.
public class JavaEnum {
public static void main(String[] args){
for(EDirection d : EDirection.values()){
System.out.println(d);
}
}
}
We get the same output that we got from the previous code.
Remember: Enums keep the order of their elements. During iteration you will get the same order of members of elements as you have put them during the enum’s creation.
Conclusion
Enums are a great feature in Java to work in an intuitive way. You do not need to put string or int and then take care of comparing them. Just put them as a symbol to use anywhere in your code. If you are not satisfied with the bare minimum functionality of enums, you can add more methods and use it as a class. Keep practicing with enums until you are comfortable with leaving the old way of using constant variables.
Check out our guide to concurrency in Java and stop by the homepage to find the perfect software component for your next project.
Recent Stories
Top DiscoverSDK Experts
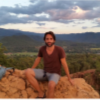
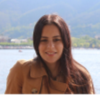
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment