ECMAScript 6 - Text Strings and Dates
One of the coolest things in ES6 is the way in which it allows us to work with text strings. Let’s jump right into an example:
var someName = 'Moshe',
price = '20$';
var message = 'Hi ' + someName + ', You owe me ' + price;
console.log(message); //"Hi Moshe, You owe me 20$"
Anyone who’s ever written anything substantial in JavaScript knows this story: in order to connect text strings, we need to use a sort of spaghetti code. There are a few libraries that do templating, but there is nothing inherent in JavaScript. That is to say, there wasn't, until ECMAScript 6 walked into our lives. Check this out:
var someName = 'Moshe',
price = '20$';
var message = `Hi ${someName}, You owe me ${price}`;
console.log(message); //"Hi Moshe, You owe me 20$"
Notice the quotation marks. We’re not talking about regular ol’ single or double quotation marks—this is the backtick (it’s on the same key as the tilda). Between them, we can put anything including complex objects.
var customer = { name: "Foo" }
var card = { amount: 7, product: "Bar", unitprice: 42 }
var message = `Hello ${customer.name},
want to buy ${card.amount} ${card.product} for
a total of ${card.amount * card.unitprice} bucks?`
Also notice that everything inside of the braces gets compiled. For instance, we could carry out computations:
var someName = 'Moshe',
price = 20;
var message = `Hi ${someName}, You owe me ${price + 30}`;
console.log(message); //"Hi Moshe, You owe me 50"
If you said that it reminds you of Angular, you’d be right. Inside of the braces you can run whatever you like.
We can take it one step farther with special functions whose sole purpose is to receive a string of a particular type and handle it. Here’s an example that’s I’ll explain afterward:
function stringManipulation (strings, ...values) {
console.log(strings[0]); //"foo\n"
console.log(strings[1]); //"bar"
console.log(strings.raw[0]); //"foo\\n"
console.log(strings.raw[1]); //"bar"
console.log(values[0]); //42
return 'someResult';
}
var myString = stringManipulation `foo\n${ 42 }bar`;
console.log(myString); //"someResult"
So what do we have here? We have a special function that I called stringManipulation, but it could be called anything. I pass my expression to it (in this case foo\n${ 42 }bar), and notice that it’s inside of those backticks. Also notice that I’m passing that text string in not the prettiest manner, but it’s necessary for functions that handle text strings. I print the result that I get from the function. In this case since the function returns someResult, that’s exactly what I’ll receive.
What’s interesting here is what’s happening inside the function, which uses the ES6 argument syntax that we covered earlier. We have access to everything inside the expression. From the simple text that’s passed that comes inside the array, to the values inside the braces that also come in an array. In the example I’m just printing them, but in reality I can do whatever I want and return whatever I want.
Now, isn’t it true that compared to generators, breaking down arrays, and for in loop, this is really easy and something we can use right away?
Well if you thought it can’t get any better, say hello to the i18n function. For those of you not familiar with this, we’re talking about a nickname for internationalization—between the i and the n there are 18 letters. An example of this internationalization is how in the USA the date is written with the month first, as opposed to the day first in most of the world. So in the US September first is written 09/01, where in the rest of the world it would be 01/09. Or regarding the order of alphabets, the Teutonic Germans put the ä right after the a, but the viking Swedes put it after the z. And there are a host of other little things like that which have been making people lose sleep for many a generation.
ECMAScript 6 helps us with this madness by way of the intl object. For those of you who want to take a peek at MDN, you’ll find there are a few interesting plans that can help with internationalization issues.
Let’s check out sorting order.
Using Intl.Collator we can set a different sort order for lists. This is more or less a sorting function customized to a langage. So let’s take a scene from real life. Let’s say we have an array with members in Hebrew and in English and we need to sort them. Obviously we’ll use sort on the array. But unfortunately it will put the Hebrew values first. But we can use collator and get result like these:
var array = [ "a", "b", "א" ]
var l10nHE = new Intl.Collator("he")
console.log(array.sort(l10nHE.compare)) // ["א", "a", "b"]
console.log(array.sort()) // ["a", "b", "א"]
This is really more relevant to other languages where the same letters appear in different orders. Remember the difference between German and Swedish? Here the Intl.Collator solves the problem with elegance:
var array = [ "ä", "a", "z" ]
var l10nDE = new Intl.Collator("de")
var l10nSV = new Intl.Collator("sv")
console.log(array.sort(l10nDE.compare)) // [ "a", "ä", "z" ]
console.log(array.sort(l10nSV.compare)) // [ "a", "z", "ä" ]
Another cool example is with numbers. In most places it customary to write numbers with a comma, as in 1000 is show 1,000. But not everywhere—in Germany for example they use a dot instead of a comma. Before ES6 we had to sweat blood with this one, but now it’s super easy. We just need to use the country and language codes according to the ISO and use the Intl object. We create a NumberFormat with the appropriate country code and then we can format any number according to our needs. Don’t believe me?
var l10nEN = new Intl.NumberFormat("en-US")
var l10nDE = new Intl.NumberFormat("de-DE")
console.log(l10nEN.format(1234567.89)); // "1,234,567.89"
console.log(l10nDE.format(1234567.89)); // "1.234.567,89"
We can now use our number example with currencies (cha-ching!). If we have the country’s currency code we can print out a price in the number format of the country. Check it out:
var l10nUSD = new Intl.NumberFormat("en-US", { style: "currency", currency: "USD" })
var l10nGBP = new Intl.NumberFormat("en-GB", { style: "currency", currency: "GBP" })
var l10nEUR = new Intl.NumberFormat("de-DE", { style: "currency", currency: "EUR" })
var l10nISR = new Intl.NumberFormat("he-IL", { style: "currency", currency: "ILS" })
console.log(l10nUSD.format(100200300.40)); // "$100,200,300.40"
console.log(l10nGBP.format(100200300.40)); // "£100,200,300.40"
console.log(l10nEUR.format(100200300.40)); //"100.200.300,40 €"
console.log(l10nISR.format(100200300.40)); //"100,200,300.40 ₪"
Now try and say this isn’t cool and easy.
We’ll finish up with dates. Some countries put... OK well, the USA puts the month first and then the date. Why? WHY?? God and the Americans alone know, and I’m not entirely sure that God really gets it. But that’s just how it is and we’ve got to just deal with it. So what do we do? Here too Intl gives us plenty of good options to change the date format in the same manner. Note that the format here takes the Date object and not the text string or the number.
var l10nEN = new Intl.DateTimeFormat("en-US")
var l10nDE = new Intl.DateTimeFormat("de-DE")
var l10nHE = new Intl.DateTimeFormat("he-IL")
console.log(l10nEN.format(new Date("2015-12-02"))) //"12/2/2015"
console.log(l10nDE.format(new Date("2015-12-02"))) //"2.12.2015"
console.log(l10nHE.format(new Date("2015-12-02"))) //"2.12.2015"
This right here, is a really really useful thing. Till next time.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
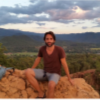
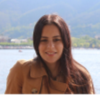
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment