ECMAScript 6 - Breaking Down Arrays
Arrays that contain data or objects are the heart of hearts of every JavaScript based application. So of course the new standard didn’t ignore this and contains methods and means to break down and handle them. In this article we’ll examine the new ways that ECMAScript 6 gives us to break down arrays and objects.
As we know, there are ways in JavaScript to build arrays and objects. For instance:
var obj = {};
obj.first = 'John';
obj.last = 'Doe';
We can also extract data from arrays or objects, like so:
var f = obj.first;
var l = obj.last;
So far there’s nothing new here. So where’s the innovation? In ECMAScript 6 we can extract from an array or object via a pattern, making everything more readable. For instance, in the example above, if we know the structure of the object we can extract the last and the first to the variable l and f very easily. Like so:
var object = {first: 'John', last: 'Doe'};
var {first: f, last: l} = object;
console.log(f); //"John"
console.log(l); //"Doe"
Much nicer, don’t you agree? It’s possible to do this with arrays too. ECMAScript 6 allows us to do this very easily:
var iterable = ['a', 'b'];
var [x, y] = iterable;
console.log(x); //a
console.log(y); //b
Of course we don’t need to take the entire array or object but rather just sections of it. Here for instance, we take only the first property from the object and the first member of the array:
var object = {first: 'John', last: 'Doe'};
var {first: f} = object;
console.log(f); //"John"
var iterable = ['a', 'b', 'c'];
var [x] = iterable;
console.log(x); //a
We can continue going wild if we like. We can even take a value (or array , or object) from a really complex object, so long as we state its value. For instance:
var complicatedObject = { a: [{ foo: 123, bar: 'abc' }, {}], b: true };
var { a: [{foo: newVarFromComplicatedObject}] } = complicatedObject;
console.log(newVarFromComplicatedObject); // f = 123
If of course the object doesn’t contain a or foo, we’ll receive undefined. And if we want a default value, well that’s possible too.
var emptyArray = [];
var [myValue = 5] = emptyArray;
console.log(myValue); //5 - because of default. otherwise - undefined
In the current example, I took an array and broke it down in order to put in the first value inside the variable myValue. Well, guess that array must be empty! What should have happened here is that myValue should have received undefined. But it’s gonna’ be OK—we used a default value (just like the default value of a function that we we covered in a previous article).
It’s also possible to work with a pattern, like we learned in a previous article. How? Like so:
var someArray = ['a', 'b', 'c'];
var [x,...y] = someArray;
console.log(x); //"a"
console.log(y); //["b", "c"]
What’s going on here? We’re taking the first member of the array, putting it in x, and then everything else is going in y. This works really well with objects as well.
We can also break things down as function variables. Here’s an example with an array, but we can also do this on objects:
var someArray = [1,2,3,4]
function f ([ x, y ]) {
console.log(x, y);
}
f(someArray); //1,2
Here are all the examples in one CodePen for you. Practice a bit and you’ll see just how easy it is.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
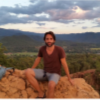
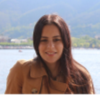
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment