Design Patterns in JavaScript - Singleton
The Singleton Pattern is perhaps one of the most common design patterns that is being implemented with a broad range of computer programming languages. So let’s dive into the basics what is Singleton? From the name itself “single” simply means that it is an object that can be instantiated only once. It can also be repeatedly called by its constructor to return the same object ensuring that the developer doesn’t actually create another instance inside a user application.
Singletons differ from static classes, as we can call their initialization in a later part. This is true in most cases where some information from your application may not be available during the initialization time.
In JavaScript, Singleton is used to isolate code from the global namespace to prevent them from being overridden by other points of access or functions.
Here’s a relatively simple example that starts with the basic of Singleton pattern.
var person = {
firstName: 'James',
lastName : 'Harden',
callFullName : function() {
return this.firstName + ' ' + this.lastName;
}
}
console.log(person.callFullName());
The code above is Singleton. Once you create an object literal in JavaScript you are already creating a small reserve piece of memory that is unique. Depending on the scope of your local variable it can be used almost anywhere in your application. One example is jQuery in which you just place the $ and you can use it anywhere in your application.
Let’s take another example. Perhaps if you have a series of functions that is constantly being used everywhere in your application.
function lock(){
// lock account code goes here
}
function login(){
// login account code goes here
}
function logout(){
// logout account goes here
}
The functions we have created above are creating garbage, since they are still floating around the page with no concrete use whatsoever. Without being declared explicitly they don’t have a parent object to be attached with rather they will be defaulted to the globally available window object. Another possibility is that these functions will be overwritten accidentally if another developer will try to write a function named login or included a plugin that utilizes the same function name as the example above. It will generally overwrite the original function you have created ending in a really tedious debugging,
Enter the Singleton pattern, it creates only one instance of the global object without it floating around or the threat of it being overwritten
var MYAPP = {};
MYAPP.login = function(){
// lock account code goes here
}
MYAPP.logout = function(){
// login account code goes here
}
MYAPP.lock = function(){
// logout account goes here
}
As long as there is no developer who will be using the word MYAPP our code will be safe. From here on out, we can create a lot of different functions. We can also nest out other object literals to create constructor functions
MYAPP.reCaptcha = function(challenge){
// do recaptcha challenge here
console.log(challenge);
}
var newWidget = new MYAPP.reCaptcha('a123asf');
Here’s another example.
// Shopping App
var ShoppingApp = {
ITEMS_INVENTORY : 200,
locateProduct : function(productID){
// Locate product code here
},
addProduct : function(productID){
// Add product code here
},
// Initialize app
init : function(){
}
}
// Initialize once the page loads
initAppEvent(ShoppingApp.init);
// Shopping ITEMS_INVENTORY
var ShoppingInventory = {
FORM_ID: 0,
FORM_ITEM_NAME : "",
addProduct : function (){
// add product here
},
deleteProduct: function(){
// delete product here
},
displayProduct: function(){
// display products here
},
init : function(){
}
}
// Initialize once the page loads
initAppEvent(ShoppingInventory.init);
From the example above we have two Singleton objects namely the Shopping Application which holds the application properly when it comes to adding products or locating them. The other example is the Shopping Inventory which functions as the adding form of products to be included in the Shopping application.
You can also create a Singleton with a self-executing function which have private members
var MachineShop = {};
MachineShop.Singleton = (function(){
// Private Members
var Tools = ['Jackhammer','Screwdriver','Saw'];
// Private Method
function useTool(tool){
// use tool code here
}
// Private Method
function returnTool(tool){
// return tool code here
}
return{
// Public Member
MachineShopName : "Tom's Shop",
// Public Method
buyTools: function(){
// todo
}
}
})();
So let’s modify the code to create a lazy load singleton from the example above.
MachineShop.Singleton = (function(){
function constructor(){
// Private Members
var Tools = ['Jackhammer','Screwdriver','Saw'];
// Private Method
function useTool(tool){
// use tool code here
}
// Private Method
function returnTool(tool){
// return tool code here
}
return{
// Public Member
MachineShopName : "Tom's Shop",
// Public Method
buyTools: function(){
// todo
}
}
}
})();
Both of the codes above are technically the same. However, we modified the second code below to handle lazy load.
The Singleton pattern is widely used in most web applications since we tend to often use the same functions all over again.
Recent Stories
Top DiscoverSDK Experts
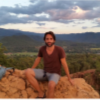
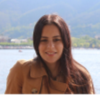
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment