Control Flow in Kotlin
Welcome to part 2 of our intro to Kotlin series. In the last installment, we gave you a quick overview of Kotlin and covered some basic types. Today, we’ll take a look at control flow in Kotlin and give you some examples for various types of control flow. Let’s just jump right into it with the classic...
If Else
Kotlin is a functional language. This means that, just like in every other functional language, if is an expression and not just a keyword. An if expression will return a value whenever necessary. Similar to other programming languages, an if-else block is used as an operator for initial conditional checking. Let’s turn to our first code example in which we’ll compare two different variables and see what the output we get is.
fun main(args: Array<String>) {
val a:Int = 99
val b:Int = 21
var max: Int
if (a > b) {
max = a
} else {
max = b
}
print("The maximum between a or b is " +max)
// As an expression
// val max = if (a > b) a else b
}
This little codeblock gives us the following output:
The maximum between a or b is 99
Note that there is another line of code that shows how to use the if statement as an expression.
Use of When
Assuming that you’re familiar with one or two other programming languages, you’re probably familiar with switch statements. These are conditional operators where multiple conditions can be easily applied to a variable. Kotlin doesn’t have a switch, but it does have a when, which is kind of like a switch on steroids.
The when operator compares the value of the variable against the branch conditions. If it meets a branch condition then it will execute the statement inside of that particular scope. Let’s look at an example that should clarify it:
fun main(args: Array<String>) {
val x:Int = 5
when (x) {
3 -> print("x = = 3")
4 -> print("x = = 4")
else -> { // Notice the block
print("x is neither 3 nor 4")
}
}
}
This code block returns the following output:
x is neither 3 nor 4
In the example above, the Kotlin compiler compares the value of x with the two branches. Since the variable does not match either of the branches, it will execute the else block. Practically speaking, when is equivalent to multiple if blocks. Kotlin provides another flexibility to the developer, where the developer can provide multiple checks in the same line by placing a comma in the checks. Let’s change the previous example to see how that looks:
fun main(args: Array<String>) {
val x:Int = 5
when (x) {
3,4 -> print(" The value of x is either 3 or 4")
else -> { // Note the block
print("x is neither 3 nor 4")
}
}
}
Run the updated code and see what you get. It should be:
x is neither 3 nor 4
For Loop
Loops, as you’re probably well aware, are an important part of any programming language as they allow us to iterate through any kind of data structure. Like any other programming language, Kotlin provides us with many kinds of looping options and the for loop is probably the most important among them. The implementation and use of for loops in Kotlin is conceptually similar to that of Java’s for loop. Since you’re undoubtedly familiar with for loops, let’s just jump right into an example:
fun main(args: Array<String>) {
val items = listOf(1, 2, 3, 4)
for (i in items) println("array values: "+i)
}
In this little piece of code, we’ve declared a list called “items” and used a for loop that we are iterating through. It defines a list and prints out its values. Here is what you should see upon running the code:
array values: 1
array values: 2
array values: 3
array values: 4
Here’s another example with a for loop using a library function that will make our life as a developer that much easier:
fun main(args: Array<String>) {
val items = listOf(8, 13, 21, 34)
for ((index, value) in items.withIndex()) {
println("the element at $index has a value of $value")
}
}
Run the code above in your local environment or just in a Kotlin playground and you’ll get back the following:
the element at 0 has a value of 8
the element at 1 has a value of 13
the element at 2 has a value of 21
the element at 3 has a value of 34
Let’s move on to our next types of loops.
While Loop and Do-While Loop
The loops while and do-while work just like they do in pretty much any other programming language. The notable difference between these two loops is, in case of do-while, the condition is tested at the end of the loop. The following example shows the usage of the while loop:
fun main(args: Array<String>) {
var x:Int = 0
println("Example of a while loop:")
while(x <= 7) {
println(x)
x++
}
}
This code block returns the following output:
Example of a while loop:
0
1
2
3
4
5
6
7
10
This brings us to the do-while loop. Here, the body of the loop will be executed only once, and then the condition is checked. If you didn’t quite wrap your head around that, a quick example should clear it up. Here’s a do-while loop in Kotlin:
fun main(args: Array<String>) {
var x:Int = 0
do {
x = x + 10
println("I am inside a do block: "+x)
} while(x <= 50)
}
Get it? Here, the Kotlin compiler executes the do block and then goes back to check the condition. This is the output you should receive upon running this piece of code:
I am inside a do block: 10
I am inside a do block: 20
I am inside a do block: 30
I am inside a do block: 40
I am inside a do block: 50
I am inside a do block: 60
Using Return, Break, and Continue
Control flow is more than just loops. If you are familiar with other programming languages, then you should know about a few common keywords that allow us to implement good control flow in our applications. The three most important of these keywords are: return, break, and continue. Let’s look at how each one can be used to control the loop or any other type of control flow in Kotlin.
Return: Return is a keyword that, well, returns something! Usually, it returns a value to the calling function from the called function. In this next example, we’ll implement the scenario in question:
fun main(args: Array<String>) {
var x:Int = 50
println("The value of x is: "+doubleMe(x))
}
fun doubleMe(x:Int):Int {
return 2*x;
}
In the code example above, we are calling another function and multiplying the input by 2. Then, we’re returning the value we receive to the called function which is our main function. Kotlin defines the function in another manner; we will explore this topic in a future article. For now though, it’s enough to understand that the code example here will return following output:
The value of x is: 100
Continue & Break: Continue and break are critical elements to logic and control flow in Kotlin. The break keyword terminates the control flow if a particular condition has failed and continue does just the opposite—it resumes the flow. All these operations happen with immediate visibility. Kotlin is more advanced than other programming languages in that the programmer can apply more than one label for visibility. The following example shows how we implement this label in Kotlin.
fun main(args: Array<String>) {
println("Break and Continue Examples")
myLabel@ for(x in 1..10) { // appling the custom label
if(x == 5) {
println("I am inside an if block with a value of "+x+"\n— as such the operation will be closed")
break@myLabel //specifying the label
} else {
println("I am inside an else block with a value of "+x)
continue@myLabel
}
}
}
The piece of code above returns the following output:
Break and Continue Examples
I am inside an else block with a value of 1
I am inside an else block with a value of 2
I am inside an else block with a value of 3
I am inside an else block with a value of 4
I am inside an if block with a value of 5
— as such the operation will be closed
As you can see, the controller continues the loop, until and unless the value of x is equal to 5. Once the value of x hits 5, it executes the if block and then, once the break statement is reached, the entire control flow terminates the program execution.
That’s it for this week. Check back soon for the next article in the series.
Previous Article: Kotlin Intro & Basic Types | Next Article: Classes & Object in Kotlin |
Recent Stories
Top DiscoverSDK Experts
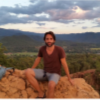
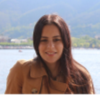
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment