Building A User Interface with ReactJS
In the midst of the night, I decided to highlight the features of a powerful Javascript library... React.js... while teaching you to build a user interface within a process.
In my previous article on Javascript Frameworks, I have mentioned about React.js -- which is an open-source Javascript library used for building views that is rendered as HTML in your web browser. React provides views which can be used for any interactive graphics creation, including but not limited to, sidebars, tabs and most importantly: user interfaces.
The React.js code is merged with your HTML code in the front-end side, so when you load the page on the browser, then it will automatically use the external libraries (as long as you put the reference to it in the start).
React.js allows you to create rendering objects and graphics (i.e., widgets, UIs, etc.). All you need to do is design the declarative views (that are extremely easy to do!) for each state in your application, and so the React will automatically update and render the right components (when the data changes) without any extra efforts!
React.js is maintained by Facebook, Instagram and the independent developers that participate in the development of React.js. It has already been used in Netflix, Airbnb and Yahoo!. This assures the developers that React.js promise the long-term solution and isn't going to die sooner or later. Thanks to its open-source nature, you can download the whole source code, modify it per your needs, and even share your changes to the worldwide!
It doesn't make sense to talk about React.js without giving the introduction on it and some examples using React.js plus the guide on building a user interface, so I thought it would be a great idea to write an article on it, as that will further attract the audience to the amazing power of React.js. Are you ready?
Building a user interface using React.JS
Let's talk about its features
Before we move into the examples of the rendering of views, I should highlight the features it support, so you can get the glimpse of the power of the React.
- One-way data flow - In React.JS, the components are immutable (i.e., unable to be changed) and the data within them is unable to change. React.JS supports only the data that is flowing downstream in one direction. so, for example, if you want the data to flow in another direction, then you need additional features (or use external utilities). It listens only to the data that is flowing from upstream and not the data that is coming from each other. This is why the components always remain synchronized and operating smoothly, thus making React.js the most efficient Framework for creating UIs.
- Components based - In React.js, everything is composed of the components that are separated from each other, so you need to think everything in terms of components from now on. This helps you to maintain the large projects, as it reduces the need to write boilerplate code. This also enables you to use external libraries for UI building or something else, as every functionality can be added as the components.
- Lightweight Virtual DOM - The cool thing about React.js is that it creates its own virtual DOM (Document Object Model), which enhances the performance dramatically. Basically, the components live in the Virtual DOM (think of the DOM as the instances of the same HTML page), and React does not update it on the fly when the data is changed, because virtual DOM is actually the data in this case and not the rendered content. It first determines the details of changes in the DOM and then the updated DOM will be re-rendered (this is very useful for building user interfaces!), in that sense, React simply acts as an intermediate representation of the DOM, so the users can decide which elements need to be changed and see the results.
- Can run on server through Node - React can also render on the server using Node. This means not only you can use the React for the front-end side, but also for the back-end side. This means that the same knowledge of React can be applied to the back-end side as well, thus reducing your time that you may spend learning the other technologies.
- JSX - React.js supports JSX language for writing markup components or binding events. This is more or less like XML language. It is a preferable choice for web developers, considering that it requires less lines of code for getting the same results. It changes the inner HTML property of a DOM element in order to achieve results. In simpler words, JSX allows you mix Javascript with HTML through simple functions, although you can still use Javascript solely for whatever needs you have, but JSX functions help in performing certain operations efficiently.
- Huge SEO support - There has been several complaints in the Javascript community that Javascript Frameworks are not SEO friendly because they don't allow the SEO techniques to employ in the web page. As React works with virtual DOM, the Node is used for running it on the server, this means that the virtual DOM will be rendered as normally and returned to the web browser as if it's a regular web page!
- React Native - The libraries called "React Native" are supported by the React developers that provide the React architecture to native iOS and Android applications, which means you can build mobile applications using Javascript through the same design as React. It builds on the principle of "Learn once, Write anywhere" as you need to learn the React only once and easily port the applications to mobile platforms.
- Facebook support - The success of any technology is highly dependent on the support it receives from the big organizations. As React is developed by Facebook, plus it got the support from Instagram, you can understand that it targets the highly professional environment, so if you use it, you are using the technology of a giant company! And, it's an open-source, so you can always add your contributions!
Prerequisite
Before moving on to building the user interface, you need to first download the required files. Go the Getting Started (https://facebook.github.io/react/docs/getting-started.html) page and download the "Starter Pack". You can find several examples in the folder, all you need to do to see the "action" is just click on the "index.html" file in every example folder, and you will see the results in your web browser.
If you copy those files somewhere else (i.e., outside the folder), then they won't run successfully, because they use certain Javascript React files that are present in the original folder, so you need to either copy those files somewhere or change the address to the current directory. For example, let's look at the example in "basic" folder:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Basic Example</title>
<link rel="stylesheet" href="../shared/css/base.css" />
</head>
<body>
<h1>Basic Example</h1>
<div id="container">
<p>
To install React, follow the instructions on
<a href="https://github.com/facebook/react/">GitHub</a>.
</p>
<p>
If you can see this, React is <strong>not</strong> working right.
If you checked out the source from GitHub make sure to run <code>grunt</code>.
</p>
</div>
<h4>Example Details</h4>
<p>This is written in vanilla JavaScript (without JSX) and transformed in the browser.</p>
<p>
Learn more about React at
<a href="https://facebook.github.io/react" target="_blank">facebook.github.io/react</a>.
</p>
<script src="../../build/react.js"></script>
<script src="../../build/react-dom.js"></script>
<script>
var ExampleApplication = React.createClass({
render: function() {
var elapsed = Math.round(this.props.elapsed / 100);
var seconds = elapsed / 10 + (elapsed % 10 ? '' : '.0' );
var message =
'React has been successfully running for ' + seconds + ' seconds.';
return React.DOM.p(null, message);
}
});
// Call React.createFactory instead of directly call ExampleApplication({...}) in React.render
var ExampleApplicationFactory = React.createFactory(ExampleApplication);
var start = new Date().getTime();
setInterval(function() {
ReactDOM.render(
ExampleApplicationFactory({elapsed: new Date().getTime() - start}),
document.getElementById('container')
);
}, 50);
</script>
</body>
</html>
Here, the "<script src...." element contains the default address, so if you move the file to somewhere, then you need to change the directory to the folder you have downloaded the "Starter Pack" (basically the "build" folder), or just copy the files in "build" folder wherever your file is moved (though make sure the address don't have "build" in it anymore as it will read files from the same location where the DOM files are present).
This is a very simple example. Basically, it's a simple HTML page where the <script> element contains the React.js code written in Javascript. It uses the render() function here which contains few mathematical functions, and then it returns the result to the DOM. Later, the DOM pushes the result to the 'container' Id. This is a short example of how React processes between DOM and the front-end page!
In this manner, you can test every examples. Note that here we are using the web browser to render the Javascript content. In order to execture the Javascript files standalone, then you would need to download the npm (package manager for Javascript) which is included in the Node.js environement, which you can download from here (https://nodejs.org/en/download/) [Download the .msi installer for the Windows and NOT the binary, as this will allow you to use npm commands in command-line of the Windows).
Once the downloading is finished, create a new file, "main.js" and write the following code:
console.log("Hello, World!")
Then open the command-line, and write "npm main.js". It should print "Hello, World!" on the console if the installation is successful. So, now you can write standalone Javascript programs without embedding the Javascript code in the HTML pages!
Some basics of React.js
React only cares for the view layer, and the DOM is updated when the data is changed to reflect in the rendering. Think of React as the "V" in the MVC (Model–view–controller). React covers only the view layer of the app though, so you need to choose other technologies to get a complete toolset for the development. For this reason, React attracts the audience that desire the powerful front-end technology and already understand the other technologies.
Basically, you integrate the React.js code in your normal HTML pages within the script element. You can write the code in either Javascript or JSX. If you open the examples, then you will see the render and event functions and may get the gist of how React works (in case you have alredy understood my previous example).
Previously, you have seen the standalone examples in the "Starter Pack", but what if you wanted to do the React development on your web server? React requires some dependencies on the modules to operate correctly, so you need something that automatically downloads the dependencies for you. Fortunately, there are already several options that do the job pretty well, including, browserify and webpack.
The cool thing about web servers is that they allow you to update the code on the fly and see the results simultaneously! (as the update happens synchronously!). Regarding dependencies: Mostly you need two dependencies: react.js and react-dom.js, so let's see how to use React through npm (considering it is installed on your computer). We will use webpack for the modules and babel as the compiler for Javascript or for transpiling the React / JSX code to Javascript code:
1) Installing webpack:
Open your command-line, and write the following commands:
C:\Users\yourname>npm install webpack --save
C:\Users\yourname>npm install webpack-dev-server --save
2) Installing react.js and react-dom.js:
Open your command-line, and write the following commands:
C:\Users\yourname\Desktop\reactExample>npm install react --save
C:\Users\yourname\Desktop\reactExample>npm install react-dom --save
3) Installing babel plugins
Open your command-line, and write the following commands:
C:\Users\yourname\Desktop\reactExample>npm install babel-core
C:\Users\yourname\Desktop\reactExample>npm install babel-loader
C:\Users\yourname\Desktop\reactExample>npm install babel-preset-react
C:\Users\yourname\Desktop\reactExample>npm install babel-preset-es2015
4) Creating a folder and files
Now, you need to create a new folder for the project:
C:\Users\yourname\Desktop\reactExample>touch index.html
C:\Users\yourname\Desktop\reactExample>touch App.jsx
C:\Users\yourname\Desktop\reactExample>touch main.js
C:\Users\yourname\Desktop\reactExample>touch webpack.config.js
Following are the content of the files:
webpack.config.js:
var config = {
entry: './main.js',
output: {
path:'./',
filename: 'index.js',
},
devServer: {
inline: true,
port: 8080
},
module: {
loaders: [
{
test: /\.jsx?$/,
exclude: /node_modules/,
loader: 'babel',
query: {
presets: ['es2015', 'react']
}
}
]
}
}
module.exports = config;
index.html:
<!DOCTYPE html>
<html lang = "en">
<head>
<meta charset = "UTF-8">
<title>React App</title>
</head>
<body>
<div id = "app"></div>
<script src = "index.js"></script>
</body>
</html>
App.jsx
import React from 'react';
class App extends React.Component {
render() {
return (
<div>
Hello World!
</div>
);
}
}
export default App;
main.js:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App.jsx';
ReactDOM.render(<App />, document.getElementById('app'));
5) Running the server
You can start your server by running the following command:
C:\Users\username\Desktop\reactApp>npm start
In my case, it says that the server is hosted at http://localhost:8090 , so whatever address it gives, just open it in the browser, and you will see the results.
In this example, I have created four files: webpack.config.js, index.html, App.jsx, main.js. Let's see why I have created them!
The webpack.config.js MUST be created, because it is a configuration file for the webpack, as it mentions the requirments and dependencies of the project. Without it, you can't run your project.
index.html is a single HTML page for single-page applications (which contains nothing but "app" id and script calling of "index.js") which is the file that is output by the webpack. You can add as much additional code as you want, but you need to call the other files within it.
App.jsx contains the render() function which has the actual Javascript code.
Finally, the main.js contains the render() function which renders the content to the web page from the DOM. It imports two files: 'react' which is the main source-code, and the 'react-dom' which is the rendering engine.
Some thoughts on JSX
If you see the render() function, then you will see that I have written HTML code within the <div> element. Basically, JSX isn't really much different from the HTML. For example, you can use h1, h2, h3, etc. headings too or any nested elements of the HTML (with some exceptions). I wouldn't talk much about JSX at this moment, because there are plenty of tutorials online already about it (or I may write about it later).
You need Components...
Most important thing in the JSX (that will help in the buildig of user interface) is the components. Open the App.jsx file, and change the code to the following:
App.jsx
import React from 'react';
class App extends React.Component {
render() {
return (
<div>
<Header/>
<Content/>
</div>
);
}
}
class Header extends React.Component {
render() {
return (
<div>
<h1>Header</h1>
<p>This is the header text</p>
</div>
);
}
}
class Content extends React.Component {
render() {
return (
<div>
<h2>Content</h2>
<p>This is the content text { 3 + 2} </p>
</div>
);
}
}
export default App;
Here, we are using render() function again, but with different components, so you need to call reactDOM.render() in your main.js (which you already did previously), so you just refresh your browser page and see the results.
Here, in our <app> element, we have defined two elements: <Header> and <Content>, and in these elements, we have used the headings elements. So, you can see that components are nothing but the way to write nested elements in the JSX. So, you can write the HTML-like code within the functions or ES6 scripts, and babel will transcompile it to standard Javascript code. This allows you to organize your code in a powerful fashion, as now everything can be defined separately and then nested into the HTML.
Some thoughts on states and events...
Logic of the programm can be built using the states and events in React. Think of the states as the switches or the state of the program that calculates the progress of something, let's say you want to calculate the time since your program is running. It gets updated through DOM, which means you get extremely fast performance.
If you open the "basic-click-counter" example, you will realize that it uses the "<button onClick={this.handleClick}>" to check the clicks and then increment the "count" variable accordingly.
Building a user interface
React itself doesn't provide any functions to create UIs on its own, as the developers wanted to make the library as flexible as possible. As the React provides components, which allows you to add any functionality you want as if it's separate from other functionalities, it shouldn't be hard to add GUI features in the components. There are several libraries for building UIs, including, but not limited to Belle, Elemental UI, Material-UI, Stardust, React Islands, Semantic UI, etc.
In this guide, I will show you the guide to build UI through Material-UI and Belle. So, let's get started!
Through Material-UI
Firstly, install the material-ui plugin:
$npm install material-ui
Material-UI requires a theme to be provided. The theme will be used a placeholder for adding different components. Let's see the example to test the plugin:
App.jsx
import React from 'react';
import ReactDOM from 'react-dom';
import MuiThemeProvider from 'material-ui/styles/MuiThemeProvider';
import RaisedButton from 'material-ui/RaisedButton';
const App = () => (
<MuiThemeProvider>
<RaisedButton label="Default" />
</MuiThemeProvider>
);
export default App;
main.js
import React from 'react';
import ReactDOM from 'react-dom';
import injectTapEventPlugin from 'react-tap-event-plugin';
import App from './App.jsx';
injectTapEventPlugin();
ReactDOM.render(<App />, document.getElementById('app'));
index.html
<!DOCTYPE html>
<html lang = "en">
<head>
<meta charset = "UTF-8">
<title>React App</title>
</head>
<body>
<div id = "app"></div>
<script src = "index.js"></script>
</body>
</html>
package.json
{
"name": "reactapp",
"version": "1.0.0",
"description": "app",
"main": "1",
"scripts": {
"test": "console.",
"start": "webpack-dev-server --hot"
},
"repository": {
"type": "git",
"url": "0"
},
"keywords": [
"0"
],
"author": "danyal",
"license": "ISC",
"devDependencies": {
"babel-core": "^6.3.26",
"babel-loader": "^6.2.4",
"babel-preset-es2015": "^6.3.13",
"babel-preset-react": "^6.3.13",
"html-webpack-plugin": "^2.7.2",
"react-hot-loader": "^1.3.0",
"transfer-webpack-plugin": "^0.1.4",
"webpack": "^1.12.9",
"webpack-dev-server": "^1.14.0"
},
"dependencies": {
"material-ui": "^0.15.0",
"react": "^15.0.1",
"react-dom": "^15.0.1",
"react-tap-event-plugin": "^1.0.0"
}
}
webpack.config.js
var config = {
entry: './main.js',
output: {
path:'./',
filename: 'index.js',
},
devServer: {
inline: true,
port: 8080
},
module: {
loaders: [
{
test: /\.jsx?$/,
exclude: /node_modules/,
loader: 'babel',
query: {
presets: ['es2015', 'react']
}
}
]
}
}
module.exports = config;
Once you have created these files in a folder, then run the following commnads:
$npm install
$npm start
It shouldn't do much but show the plain page with a small default button, but it will prove that the download has been successful. "npm install" will install all the modules required for this project, and "npm start" will run the server (your console screen should output the local host address where the page is loaded, so you can go the address to see the results!).
Now we are going to design a page which will have some widgets.
1) Download Roboto Font
Firstly, you need to download the Roboto font, as Material-UI recommend this font for designing UIs. Go to this Google Web Fonts link(https://www.google.com/fonts#UsePlace:use/Collection:Roboto:400,300,500), use the appropriate settings for the font, and link your HTML page to this website and then finally add the CSS script. In my case:
Link code:
<link href='https://fonts.googleapis.com/css?family=Roboto:400,300,500' rel='stylesheet' type='text/css'>
CSS code:
font-family: 'Roboto', sans-serif;
Short example
Here's the short example of using Roboto in your HTML code.
font.html
<!DOCTYPE html>
<html>
<head>
<link href='https://fonts.googleapis.com/css?family=Roboto:400,300,500' rel='stylesheet' type='text/css'>
</head>
<style>
h1 {
font-family: 'Roboto', sans-serif;
}
</style>
<h1>Roboto Font example</h1>
</html>
This will output "Roboto Font example" with this font.
2) Building components
Material-UI uses different components for different widgets and features of the UIs. Go to the Components (http://www.material-ui.com/#/components) page and you can see the different components with the example code of every component. Building any kind of user interaction on your website is as easy as copying the component code from the site and adding it to the section of your page.
3) UI example
I won't build a complete UI in this article, but I will build a page which will give you the "gist" of how Material UI works. Let's get started!
You need to only change the "App.jsx" content. I have added some components in it.
App.jsx
import React, {Component} from 'react';
import RaisedButton from 'material-ui/RaisedButton';
import Dialog from 'material-ui/Dialog';
import {deepOrange500} from 'material-ui/styles/colors';
import FlatButton from 'material-ui/FlatButton';
import getMuiTheme from 'material-ui/styles/getMuiTheme';
import MuiThemeProvider from 'material-ui/styles/MuiThemeProvider';
import CircularProgress from 'material-ui/CircularProgress';
import AppBar from 'material-ui/AppBar';
import IconButton from 'material-ui/IconButton';
import IconMenu from 'material-ui/IconMenu';
import MenuItem from 'material-ui/MenuItem';
import MoreVertIcon from 'material-ui/svg-icons/navigation/more-vert';
import NavigationClose from 'material-ui/svg-icons/navigation/close';
import Checkbox from 'material-ui/Checkbox';
import ActionFavorite from 'material-ui/svg-icons/action/favorite';
import ActionFavoriteBorder from 'material-ui/svg-icons/action/favorite-border';
const CheckStyles = {
block: {
maxWidth: 250,
},
checkbox: {
marginBottom: 16,
},
};
const styles = {
container: {
textAlign: 'center',
paddingTop: 200,
},
};
const ButtonStyle = {
margin: 12,
};
const muiTheme = getMuiTheme({
palette: {
accent1Color: deepOrange500,
},
});
class App extends Component {
constructor(props, context) {
super(props, context);
this.handleRequestClose = this.handleRequestClose.bind(this);
this.handleTouchTap = this.handleTouchTap.bind(this);
this.state = {
open: false,
};
}
handleRequestClose() {
this.setState({
open: false,
});
}
handleTouchTap() {
this.setState({
open: true,
});
}
render() {
const standardActions = (
<FlatButton
label="Ok"
primary={true}
onTouchTap={this.handleRequestClose}
/>
);
return (
<MuiThemeProvider muiTheme={muiTheme}>
<div style={styles.container}>
<h1>Menu Bar Example</h1>
<AppBar title="Menu Bar"
iconElementLeft={<IconButton><NavigationClose /></IconButton>}
iconElementRight={
<IconMenu
iconButtonElement={
<IconButton><MoreVertIcon /></IconButton>
}
targetOrigin={{horizontal: 'right', vertical: 'top'}}
anchorOrigin={{horizontal: 'right', vertical: 'top'}}
>
<MenuItem primaryText="Refresh" />
<MenuItem primaryText="Help" />
<MenuItem primaryText="Sign out" />
</IconMenu>
}/>
<Dialog
open={this.state.open}
title="Super Secret Password"
actions={standardActions}
onRequestClose={this.handleRequestClose}
>
1-2-3-4-5
</Dialog>
<h1>Flat Button Example</h1>
<FlatButton label="Default" />
<FlatButton label="Primary" primary={true} />
<FlatButton label="Secondary" secondary={true} />
<FlatButton label="Disabled" disabled={true} />
<h1>Raised Button</h1>
<div>
<RaisedButton label="Default" style={ButtonStyle} />
<RaisedButton label="Primary" primary={true} style={ButtonStyle} />
<RaisedButton label="Secondary" secondary={true} style={ButtonStyle} />
<RaisedButton label="Disabled" disabled={true} style={ButtonStyle} />
<br />
<br />
<RaisedButton label="Full width" fullWidth={true} />
</div><h1>Circular Progress Example</h1><div>
<CircularProgress />
<CircularProgress size={1.5} />
<CircularProgress size={2} />
</div>
</div>
</MuiThemeProvider>
);
}
}
export default App;
Here, within the class "App", I have added several component code which is included in render() function. This "App" class is then exported for using in "main.js" file.
If you are not aware of it, this is actually a modified version of the example given here (https://github.com/callemall/material-ui-webpack-example/blob/master/src/app/Main.js). With the same logic, you can add / modify as much as you want. You just need some simple Javascript knowledge for additional functionalities.
Through Belle
Now, let's do the similar task through Belle, although this time, I won't explain how functions (i.e., render(), etc.) are working as I have already explained earlier. I will focus on the changes that need to be done.
1) Installing the Belle
Firstly, you need to install the Belle. It's as simple as running the following command in the directory of your project:
$npm install belle
2) Building components
Once it is installed, you need to copy all the contents of the folder of your previous project (Material-UI Example Project) and paste the content in a new folder for the Belle. Then open the "App.jsx" file and paste the following content:
App.jsx
var React = require('react');
var belle = require('belle');
var TextInput = belle.TextInput;
var Button = belle.Button;
var ComboBox = belle.ComboBox;
var Option = belle.Option;
var Toggle = belle.Toggle;
var Rating = belle.Rating;
var App = React.createClass({
render: function() {
return (
<div>
<h1>Text Input</h1>
<TextInput defaultValue="Write Here" />
<h1>Button</h1>
<Button primary>Follow</Button>
<Button>Follow</Button>
<h1>Toggle Button</h1>
<Toggle defaultValue/>
<h1>ComboBox</h1>
<ComboBox placeholder="Choose a State">
<Option value="Alabama">Alabama</Option>
<Option value="Alaska">Alaska</Option>
<Option value="Arizona">Arizona</Option>
<Option value="Arkansas">Arkansas</Option>
</ComboBox>
<h1>Rating</h1>
<Rating defaultValue={3}></Rating>
</div>
);
}
});
export default App;
Now, run the following command:
$npm start
You should be able to see the running example on the local host address (which will be provided on your console screen).
Here, we are doing nothing but using different components together within the <div> section. You can add as many components as you want by visiting their components page (http://nikgraf.github.io/belle/#/component/), and even add the external HTML / CSS coding to give the combined flavor. Make sure to import the correct component and assign it to the variable, like "var Button = belle.Button;", otherwise it won't run correctly.
If you want to see the big example of a sophisticated Belle project, then look no further than the Belle project (https://github.com/nikgraf/belle/tree/master/examples) page. You can git clone it to your drive and run the example to see the "power" of Belle.
Conclusion
So... finally, I have highlighted pretty much every feature of the React.js and the guide to build a user-interface. Additionally, I have mentioned the examples and the power of React.js so you can see the use cases of it. Arguably, React.js proved to be the best library for rendering the views for the building UIs, as the library is specifically designed for this purpose, so developers put the best efforts to ensure the best quality.... plus the fact that it is supported by Facebook! What else you need?
If you think I might have missed something, or you want to ask questions, then please do so in the comment section below!
Recent Stories
Top DiscoverSDK Experts
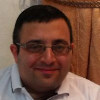
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment