Advancing into ES6 Classes
In my previous post, I introduced you to the concept of classes in EcmaScript 6. In this article we will dig deeper into classes to learn more. Without further ado, let's begin.
Getter and Setter
In object oriented programming, getters are the methods used to retrieve value from attributes (or values generated dynamically) and setters are used to set the value of the attributes. In Java getters and setters are methods and need to be called like methods, i.e. method name and the pair of parentheses. But, it will be more convenient if we could just use them like attributes. In ES6 it is possible to use getter and setter methods without calling them like methods. The JavaScript engine itself will call the method for us.
class Square{
constructor(width){
this._width = width;
}
get area(){
return this._width * this._width;
}
}
var s1 = new Square(2);
console.log("The area of the square is: " + s1.area)
Outputs:
The area of the square is: 4
Notice that we did not call area, i.e s1.area(), instead we used it like an attribute.
We can use a setter method in a similar manner. Let's create a setter method for width, so that we can set the width of the square after creating the s1 object.
class Square{
constructor(width){
this._width = width;
}
get area(){
return this._width * this._width;
}
set width(w){
this._width = w;
}
}
var s1 = new Square(2);
console.log("The area of the square is: " + s1.area)
s1.width = 4;
console.log("The area of the square after change of width is: " + s1.area)
Outputs:
The area of the square is: 4
The area of the square after change of width is: 16
Notice again that we did not call s1.width(4), instead we used it as an attribute to set the value.
Instance and Static Methods
Throughout the examples we are using instance methods with class. Instance methods are the methods associated with an object and cannot be used directly from classes. The magic variable this is also available inside instance methods.
On the other hand static methods are the methods that can be used directly from the classes and without any object created from that class. The magic variable this is not available inside static methods.
To create a static method you need to precede the method name with the keyword static. Let's see an example.
class MathC{
static square(w){
return w * w;
}
}
console.log("Square of 4 is : " + MathC.square(4));
Outputs:
Square of 4 is : 16
Notice that we used the square() function without instantiating an object of the class first.
Super
Inheritance is an unavoidable part of object oriented programming. We inherit a class into another class with the help of the extends keyword. In the child class we may need to override the methods of the parent class. But what if we need to call the parent class's method? We need to use super in this case.
Constructor is also a method. In the introductory article on ES6 classes we did not write any constructor for Doc. What if we want to add extra value to the Dog constructor and still keep the functionality of the parent Animal class? Let's try with super call in Dog.
class Animal{
constructor(name, type){
this.name = name;
this.type = type;
}
myInfo(){
console.log("My name is: " + this.name + " and I am of type: " + this.type);
}
}
class Dog extends Animal{
constructor(name, type, age){
super(name, type);
this.age = age;
}
bark(){
console.log("Woof");
}
}
var d1 = new Dog("Dog 1", "Hybrid", 6);
d1.myInfo();
d1.bark();
Outputs:
My name is: Dog 1 and I am of type: Hybrid
Woof
So, we provided an extra parameter called age and we did not have to do the work of the Animal class again. The super call did that for us. But, wait! The myInfo() call is not providing the age information of the dog. So, we can override the myInfo() method inside the Dog class. But we do not want to repeat code. We want to use what myInfo() did inside the Animal class and we also want to print the age information of the dog. So, we will use super again to call the myInfo() method of Animal from myInfo() method of Dog. Let's clarify with an example:
class Animal{
constructor(name, type){
this.name = name;
this.type = type;
}
myInfo(){
console.log("My name is: " + this.name + " and I am of type: " + this.type);
}
}
class Dog extends Animal{
constructor(name, type, age){
super(name, type);
this.age = age;
}
bark(){
console.log("Woof");
}
myInfo(){
super.myInfo();
console.log("My age is: " + this.age);
}
}
var d1 = new Dog("Dog 1", "Hybrid", 6);
d1.myInfo();
d1.bark();
Outputs:
So, we did not repeat our code and still served our purpose. That's the magic of super.
Hoisting Issues
In JavaScript or EcmaScript functions are hoisted. That means you can use or call a function when the function is defined later in source code than where it is being called. For example:
f();
function f(){
console.log("I am f");
}
It will output:
I am f
The function is called first and after that the function was defined. JavaScript engines go through a compilation phase and in that compilation phase it does not run anything. Instead it collects all the functions there and load them in memory with their name. So, it seems like that those functions are moved at the top of the source code.
Hoisting does not takes place for ES6 classes. Let's try to execute the following code.
var o1 = new ACls();
class ACls{}
It will throw an error:
ReferenceError: ACls is not defined
Experienced JavaScript programmers can also make the mistake by thinking that classes in ES6 are hoisted.
Conclusion
In this post I tried to cover some advanced topics that were not covered in the introductory article. In the future I will demonstrate various use of ES6 classes in various other articles, tutorials or guides. Keep coding with ES6 and advance your knowledge of its great new features.
Previous article: Introduction to ES 6 Classes |
Next article: coming soon! |
About the Author
My name is Md. Sabuj Sarker. I am a Software Engineer, Trainer and Writer. I have over 10 years of experience in software development, web design and development, training, writing and some other cool stuff including few years of experience in mobile application development. I am also an open source contributor. Visit my github repository with username SabujXi.
Recent Stories
Top DiscoverSDK Experts
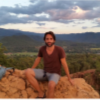
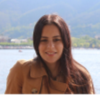
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment