A Comparison of Different Javascript Transpilers
A transpiler is a source-to-source compiler that basically translates the source-code written in one programming language to the source-code of another programming language. Javascript (also often known as ECMAScript) has been a part of us since its initial release in 1995. If you have ever touched the web development, then you know for sure that it is often used along with HTML and CSS. Anecdotal evidence suggests that no matter how many updates an old language gets, it always seems outdated or old-fashioned, especially when compared to modern newly created languages.
Developers understand pretty well that almost every website on the internet is powered by Javascript, or at least use certain utilities that are powered by Javascript. Creating a new programming language or trying to replace it with another language is next to impossible, as it will take years to convert the old code to new code let alone the sudden breaking of projects that will happen.
Thankfully, we have witnessed several new programming languages that compiles (read: transpiles) to Javascript, which means you can write your new code in an extremely modern and elegant looking language like CoffeeScript, TypeScript, etc. through the tools like Babel (that turns your ES6+ code into ES5 friendly code) and others. This created an additional problem: You search Javascript Transpilers, and you get several of them, so which one do you choose?
In this article, I am going to highlight different Javascript transpilers and how they differ from each other, so that you can strategically decide the best option for your next Javascript project. Are you ready?
Which Language Should I Choose?
1) CoffeeScript
If you have used Ruby, Python and Haskell, then you are aware of their syntactic sugar features (https://en.wikipedia.org/wiki/Syntactic_sugar). Programmers always miss modern OOP (Object-Oriented Programming) features in Javascript. Thankfully, CoffeeScript adds new features that dramatically shortens the code and enhances the readability. For example, in CoffeeScript, almost everything is an expression that includes if, switch, for expressions in a similar fashion to Python, Ruby, etc., which means it returns a value (unlike Javascript, which don't return the value!), so it means you can operate on a function and assign the return value of a function to another function in a single line!
area = (x, y) -> x * y // area is a function name
square = (x) -> area(x, x) // assigning the return value of area to function 'square'
CoffeeScript allows you to write code easily without boilerplate code, because you don't have to write parentheses and commas. The cool thing about CoffeeScript that despite allowing you to write in simple language, you get exactly the same performance as Javascript. Why? Because the code compiles into Javascript eventually, which you can see yourself and compare it with actual Javascript code, you won't see much difference!
So, now let's write some code with Coffeescript!
CoffeeScript is a compiler itself, so you need to first install it (assuming you have Node.js installed on your computer, if you don't have it, then download it from here (https://nodejs.org/en/download/)):
$npm install -g coffee-script
Once it is installed, now you can use the "coffee" command.
You can use CoffeeScript with Node.js, in fact, CoffeeScript is available with Node.js along with V8 Javascript virtual machine, so if you have Node.js installed, you don't need to install CoffeeScript separately.
Create a new file "sample.coffee" and then write the following code
for i in [0..5]
console.log "Hello, World! #{i}"
Now, run the following command:
$coffee sample.coffee
You will see the results on the console screen!
You can also embed the code into HTML, which runs the CoffeeScript code through the help of jQuery, so create a new file called "index.html" and write the following code in it:
<html>
<head>
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js">
</script>
<script
src="//jashkenas.github.com/coffee-script/extras/coffee-script.js">
</script>
<script type="text/coffeescript">
gcd = (a,b) -> if (b==0) then a else gcd(b, a % b)
$("#button").click ->
a = $("#a").val()
b = $("#b").val()
$("#c").html gcd(a,b)
</script>
</head>
<body>
A: <input type="text" id="a"/><br/>
B: <input type="text" id="b"/><br/>
<input type="button" value="Calculate GCD" id="button"/> <br/>
C: <span id="c"/>
</body>
</html>
Opening it on the browser will show you the results (i.e., a small GCD calculator!). So, now let's talk about some of its features....
Assignments are done in a very similar fashion:
number = 30
isActive = true
name = "John"
Due to its dynamic typed nature, you don't need to write the data types yourself. There is no need for curly-braces or semi-colons, as it follows the Python's convention:
if isActive and isEnabled
doIt()
else
stopIt()
The cool thing about CoffeeScript is its functions (I have mentioend it earlier), because you can write simple functions in a way as if you are writing a variable:
hello = (name = "Richard") ->
console.log("Hello, #{name}!")
hello() # prints "Hello, Richard!"
hello("John") # prints "Hello, John!"
Pros
- You get a huge amount of syntactic sugar features which not only increase the readability, but also allow you to enjoy the language more.
- It uses indentation (new line) similar to Python rather than braces, so you will see a dramatic increase in readability.
- No need to write a semicolon as the statement terminators, because they are optional.
- You can write functions in lambda-like syntax, rather than classical C way, so if you ever loved functional programming features, then you will fall in love with CoffeeScript.
- There is a huge community behind its development as many developers already moved to CoffeeScript.
Cons
- It is dynamically typed just like Javascript, so it doesn't provide compile-time type safety on its own.
- It has a larger learning curve, especially for someone who don't know the basics of web development.
- In CoffeeScript, the syntax of variable initialization and reassignment is the same, so you can accidentally make the mistake.
- The Javascript code isn't valid enough in CoffeeScript, so often its integration is difficult and takes effort.
2) TypeScript
If you are a fan of Microsoft, then you ought to know that they have created a superset of Javascript --- TypeScript, which simply adds the optional static typing and class-based object-oriented programming to the language. The cool thing about is that any Javascript will be valid in TypeScript, so you can easily integrate your old Javascript code, unlike the other transpiling languages that break the original Javascript!
So, what sets it apart from CoffeeScript? It may not be possible to understand it without seeing the code, so let's write some code in TypeScript!
You need to first install the TypeScript globally through npm:
$npm install -g typescript
TypeScript files are normally written in .ts files. So, create a file "main.ts" and write the following code:
var client: string = 'John', // String
age: number = 16 // Numeric
function speak(name: string, age: number): void {
console.log(name + "'s age is " + age);
}
speak(client, age);
Now run the following command
$tsc main.ts
It will translate the TypeScript code to Javascript (main.js) code, which you can see by opening the file on the broswer.
So, now let's talk about its features!
As I have mentioned earlier that TypeScript has static-typing, so for example, if you see the compiled Javascript code in our previous example, you will see that it doesn't have any static typing as Javascript doesn’t have it!
TypeScript has added the class-based OOP features in a fashion of Java, as people already understands the Java pretty well:
class Menu {
items: Array<string>;
pages: number;
// Simple Constructor in a fashion of Java
constructor(listofitems: Array<string>, totalnoofpages: number) {
this.items = listofitems;
this.pages = totalnoofpages;
}
list(): void {
console.log("Menu.....");
for(var i=0; i<this.items.length; i++) {
console.log(this.items[i]);
}
}
}
var usefulMenu = new Menu(["apple","orange","banana"], 1);
usefulMenu.list();
Here, you can see how easy it is to create a new class with a constructor and the list that does the operation within it
Pros
- Every piece of Javascript code is a valid TypeScript code which means these two languages integrate better than other languages.
- It follows the future ES6 Javascript standard, so the knowledge can be reused in building modern Javascript applications.
- It can run on the server through Node.js
- As it is developed by Microsoft, it chose to follow the syntax and direction of C#, so you will love this language if you are used to C#.
- It has static typing unlike CoffeeScript, so you get better compile-check, plus you also get better support on Visual Studio.
Cons
- C#-like syntax may not be suitable for many programmers who prefer Ruby-like syntax of CoffeeScript.
- It is not 100% compatible with ES6 yet, especially when compared to Babel
3) Babel (ES6 to ES5)
ECMAScript2015 is the latest version of Javascript, which significantly changes the language. It is considered a major update over the last ES5 which was introduced in 2009. However, not every Javascript engine support ES6. Fortunately, many Frameworks support the Babel -- which is a transpiler that converts the ES6 code into ES5 code which means you can enjoy the benefits of ES6 without breaking your previous ES5 code!
This (http://ccoenraets.github.io/es6-tutorial/setup-babel/) is the best tutorial on setting up the Babel on your system (it is long, so make sure you have enough time for it!)
You can also use the "Try it out" (https://babeljs.io/repl/) page online that converts the ES6 code to ES5 code in real-time!
I have already touched the Babel in my previous article on React, so I won't talk about the language, but know that unlike TypeScript, Babel is simply a transpiler and not a language on its own, which means it simply transpiles the ES6 standard to previous ES5 standard, thus allowing you to use newer Javascript features in old Javascript environments!
[1,2,3].map(n => n + 1);
// Expression bodies
var odds = evens.map(v => v + 1);
var nums = evens.map((v, i) => v + i);
Pros
- It allows you to write code in next generation ES6 Javascript, so your code will be valid in the future.
- Babel has the native support for React's JSX extensions and Flow type annotations.
- As it focuses entirely on ES6, it has the greatest level of compatibility with ES6.
- Unlike TypeScript which forces you to write code in TypeScript alone, Babel allows you to write code in either ES5 or ES6 as they both can be seamlessly integrated together.
Cons
- Due to the large learning curve, many newbie developers may dislike the necessary steps to do the simple tasks.
- Not every environment and tools support ES6, so you often need to rely on the developers' experience to deal with your problems.
4) Dart
Dart is a class-based object oriented programming language developed by Google. Due to its similar syntax (in several areas) to Javascript, it can optionally transcompile to Javascript, in fact, most people learn Dart solely as an alternative to Javascript, however, unlike previous languages, it is an entirely different language developed for different purposes outside the task of transcompiling to Javascript!
In order to install the Dart, you need to first install the Chocolatey. You can get the instructions on how to install Chocolatey from here(https://chocolatey.org/install). Basically, you need to run the following command (if you are using Windows) in the command-line (as an administrator):
@powershell -NoProfile -ExecutionPolicy Bypass -Command "iex ((New-Object System.Net.WebClient).DownloadString('https://chocolatey.org/install.ps1'))" && SET "PATH=%PATH%;%ALLUSERSPROFILE%\chocolatey\bin"
Once the Chocolately is installed, then run the following commands
$choco install dart-sdk -version 1.19.1.
$choco install dartium -version 1.19.1.
For more instructions, see this (https://www.dartlang.org/install/windows#chocolatey). During installation, it will ask if you want to run the script, so write "Y" and enter to run the script (do the same again if it asks again!).
Basically, you are installing Dart SDK and Dartium which allows you to build web applications. The Dart SDK contains pub tools that have everything, including dart2js --- The Dart-to-Javascript Compiler.
Once the installation is completed on CMD, then you need to close your CMD and then open it. Create a new file "test.dart" on your desktop and write the following code (and then save the file):
void main() {
print('Hello, World!');
}
Now (assuming your CMD is open already) change the directory of CMD to the desktop:
$ cd c:/Users/<yourname>/Desktop
$ dart2js --out=test.js test.dart
You can now see the compiled "test.js" file on your desktop! If you use WebStorm, then you don't need to install Dart manually.
So, the question is: What sets Dart apart from other languages? First of all Dart isn't designed solely for transpiling to Javascript, so many of its features may not be suitable for someone coming from Javascript. However, the language is beautiful and elegant on its own.
printNumber(num aNumber) {
print('The number is $aNumber.');
}
main() {
var number = 42;
printNumber(number);
}
You can see that it follows the structure of C/C++/C# in the sense that the program's execution starts in the main() function.
You can read more about Dart language here (https://www.dartlang.org/samples) and then test the examples as much as you want.
Pros
- Dart is not just a transpiler to Javascript, but also an entirely different language with various tools, like a rich editor (including support for WebStorm), its own virtual machine and finally a compiler to Javascript.
- It is developed by Google, so you get the benefits of huge support and backing from several developers.
- It is open-source licensed under a BSD license, which means you can modify the entire language as per your needs without giving a penny to its original authors.
Cons
- It was not designed solely for transpiling to Javascript, so you won't like it if you simply a need an alternative to Javascript.
- It can be very difficult to grasp its new features because of a long learning curve.
5) Elm
Elm is a functional programming language designed specifically for web browser-based graphical user interfaces. It competes with graphical user interface libraries like React, althought its highly sophisticated features can be used to build any kind of applications. Elm has always been the fan of interpolation between languages, thanks to the influence it has gained from Java, it can easily interpolate with Javascript.
In order to test the language, you need to first install it through running the following command:
$npm install -g elm
Now, create a folder called "elm-test" and then create a file called "test.elm". Copy the following code into test.elm:
import Html exposing (div, button, text)
import Html.App exposing (beginnerProgram)
import Html.Events exposing (onClick)
main =
beginnerProgram { model = 0, view = view, update = update }
view model =
div []
[ button [ onClick Decrement ] [ text "-" ]
, div [] [ text (toString model) ]
, button [ onClick Increment ] [ text "+" ]
]
type Msg = Increment | Decrement
update msg model =
case msg of
Increment ->
model + 1
Decrement ->
model - 1
Now run the following command:
$elm-make elm-test/test.elm
It will create an index.html file with the code, but as we are transpiling to Javascript, run the following command:
$elm-make elm-test/test.elm --output=main.js
This will create the transpiled version of Elm. For more information on interpolation, please see here (https://guide.elm-lang.org/interop/javascript.html), especially if you are going to call back and forth between Javascript and Elm.
import Html exposing (text)
main =
text "Hello, World!"
If you notice the syntax of the language, you will realize that is actually very similar to React, but the difference is that you can do the tasks of plain Javascript with Elm.
import Html exposing (text)
add x y =
x + y
factorial n =
List.product [1..n]
main =
text (toString (add 1 (factorial 4)))
This allows you to integrate other Javascript libraries as well, and so you are not restricted with GUI development only.
Pros
- Elm is specifically designed for graphical user interfaces, so it may be a better option for people who are coming from React.
- Its interpolation to Javascript not only allows you to transcompile Elm code to Javascript, but also call the functions back and forth between these two languages.
- Elm integrates seamlessly with Node.js and other Javascript libraries, as the codebase of Elm is very short.
- Its functional programming features will be attractive to those coming from Haskell background.
Cons
- It has a hard learning curve, especially compared with React.
- You need to learn about new stuff like FFI/Ports, etc., so integrating it to already established projects may take some time.
- Its functional programming features will be annoying to those who have been programming in non-functional languages.
Conclusion
The culture of transpiling (the idea of translating your code to another language... who thought it could be possible?) is arguably a very revolutionary and innovative for today's fast-moving world and it is helpful for the general "geeks" or software development community that had to rely on life-hacks and shortcuts within a boundary of a language to do several tasks as quickly as possible. Now the boundary is broken, so too the limitation to your creativity!
I have personally chosen CoffeeScript (due to its more readable syntax) and TypeScript (due to its compatibility with ES6), but you may need to try out different languages yourself and see which fits your taste!
Thus so far, I have compared different Javascript transpilers in a way as to keep you engaged while programming in Javascript and not get bored with web development!
If you have any further questions regarding any Transpiler, then please ask me in the comment section below! I would love to answer them for you!
Recent Stories
Top DiscoverSDK Experts
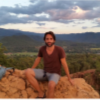
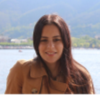
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment