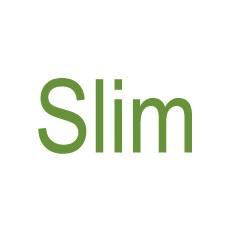
What is it all about?
Slim is a PHP micro framework that enabling quickly writing of simple and powerful web applications and APIs. Slim is easy to use and optimized for beginners as for advanced. Includes convenient and highly documented.
Key Features
* HTTP Router: Slim provides a fast and powerful router that maps route callbacks to specific HTTP request methods and URIs. It supports parameters and pattern matching. * Middleware: Build your application with concentric middleware to tweak the HTTP request and response objects around your Slim app. * PSR-7 Support: Slim supports any PSR-7 HTTP message implementation so you may inspect and manipulate HTTP message method, status, URI, headers, cookies, and body. * Dependency Injection: Slim supports dependency injection so you have complete control of your external tools. Use any Container-Interop container.
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment