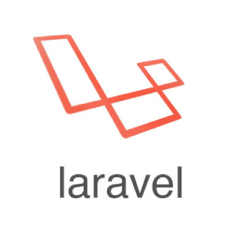
What is it all about?
Laravel is a web application framework with expressive, elegant syntax that eases common tasks frequently used in web projects such as authentication, routing, sessions, queueing, and caching.
Video & Images
Images
Key Features
Simple & fast routing engine: All Laravel routes are defined in your route files, which are located in the routes directory. These files are automatically loaded by the framework. Powerful dependency injection container: The Laravel service container is a powerful tool for managing class dependencies and performing dependency injection. Multiple back-ends for session and cache storage: Laravel ships with a variety of session backends that are accessed through an expressive, unified API which is also used for various caching backends. Expressive & intuitive database ORM: The Eloquent ORM included with Laravel provides a beautiful, simple ActiveRecord implementation for working with your database. Database agnostic schema migrations: Migrations are typically paired with Laravel's schema builder to easily build your application's database schema. Robust background job processing: Laravel queues provide a unified API across a variety of different queue backends that allow you to defer the processing of a time consuming task, drastically speeding up web requests to your application. Real-time event broadcasting: Laravel makes it easy to "broadcast" your events over a WebSocket connection. Broadcasting your Laravel events allows you to share the same event names between your server-side code and your client-side JavaScript application.
Resources
Resource Type |
Link |
---|---|
Wikipedia | https://en.wikipedia.org/wiki/Laravel |
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment