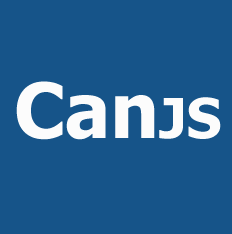
What is it all about?
CanJS is a flexible JavaScript framework designed to develop applications easily and fast. supports almost all of its MVC functionality through plugins.
Key Features
* CanJS is flexible. Unlike other frameworks, it's designed to work in almost any situation. You can readily use third party plugins, modify the DOM with jQuery directly, and even use alternate DOM libraries like Zepto and Dojo. CanJS supports all browsers, including IE8. * CanJS is powerful. Create custom elements with one and two-way bindings. Easily define the behavior of observable objects and their derived values. Avoid memory leaks with smart model stores and smart event binding. * CanJS is fast. It is only about 20k. Its live-binding updates only what needs to be updated without requiring a "diff". * CanJS is friendly. Our fulltime and core team are extremely active on gitter chat and on the forums and always wanting to help.
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment