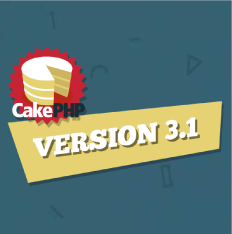
What is it all about?
CakePHP is a fast PHP framework for developers which commonly uses for design patterns like Associative Data Mapping, Front Controller and MVC.
CakePHP is designed to make common web-development tasks simple, and easy. By providing an all-in-one toolbox to get you started the various parts of CakePHP work well together or separately.
Key Features
* Build Quickly: Use code generation and scaffolding features to rapidly build prototypes. * No Configuration: No complicated XML or YAML files. Just setup your database and you're ready to bake. * Friendly License: CakePHP is licensed under the MIT license which makes it perfect for use in commercial applications. * Batteries Included: The things you need are built-in. Translations, database access, caching, validation, authentication, and much more are all built into one of the original PHP MVC frameworks. * Clean MVC Conventions: Instead of having to plan where things go, CakePHP comes with a set of conventions to guide you in developing your application. * Secure: CakePHP comes with built-in tools for input validation, CSRF protection, Form tampering protection, SQL injection prevention, and XSS prevention, helping you keep your application safe & secure.
Resources
Resource Type |
Link |
---|---|
Wikipedia | https://en.wikipedia.org/wiki/CakePHP |
CakePHP 3.4 Red Velvet Cookbook | https://book.cakephp.org/3.0/en/index.html |
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment