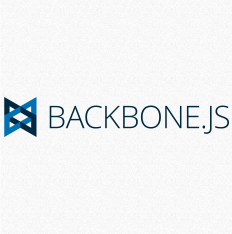
What is it all about?
Backbone.js is a JavaScript library inspired by a RESTful JSON interface that provides a VM architecture model-view-controller which is famous for its lightness and depends only on the JavaScript library underscore.js.
Key Features
A rich API Used for programming of single-page Web applications and Web applications synchronicity. Models and Views - The single most important thing that Backbone can help you with is keeping your business logic separate from your user interface. Collections - A Collection helps you deal with a group of related models, handling the loading and saving of new models to the server and providing helper functions for performing aggregations or computations against a list of models. View Rendering - Each View manages the rendering and user interaction within its own DOM element. If you're strict about not allowing views to reach outside of themselves, it helps keep your interface flexible — allowing views to be rendered in isolation in any place where they might be needed. Routing with URLs - In rich web applications, we still want to provide linkable, bookmarkable, and shareable URLs to meaningful locations within an app. Use the Router to update the browser URL whenever the user reaches a new "place" in your app that they might want to bookmark or share. Conversely, the Router detects changes to the URL — say, pressing the "Back" button — and can tell your application exactly where you are now.
Resources
Resource Type |
Link |
---|---|
toturial | http://adrianmejia.com/blog/2012/09/11/backbone-dot-js-for-absolute-beginners-getting-started/ |
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment