UART Example
Target Side
/*
* uartTask1.c
*
* Created on: May 15, 2010
* Author: root
*/
#include<unistd.h>
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<time.h>
#include <sys/prctl.h>
#include <sched.h>
#include <fcntl.h>
#include <termios.h>
#include "app.h"
#define DEVICE_FILE ("/dev/ttyS2")
struct termios options;
void set_port(int fd)
{
int status;
// Get current settings for the port
tcgetattr(fd, &options);
// Set baud rate = 1200
cfsetispeed(&options, B115200);
cfsetospeed(&options, B115200);
// Set 8 bits, no parity
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
// Raw input
options.c_lflag &= ~(IEXTEN |ICANON | ECHO | ECHOE | ISIG );
// Check and strip parity bit.
options.c_iflag = 0;
// Disable software flow control
options.c_iflag &= ~(BRKINT | ICRNL | INPCK | ISTRIP | IXON | IXOFF | IXANY);
// Output to be raw
options.c_oflag &= ~(OPOST | OLCUC | ONLCR | OCRNL );
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = sizeof(uart_buffer_t);
// Set new options for port
status = tcsetattr(fd, TCSANOW, &options);
}
void uartTask1()
{
int msgCount = 0,count = 0;
int fd;
struct timespec waitTime;
uart_buffer_t buf_out,buf_in;
struct sched_param param;
param.sched_priority = 10;
// sched_setscheduler(0,SCHED_FIFO,¶m);
waitTime.tv_nsec = 20 * MILI_SECOND;
waitTime.tv_sec = 0;
prctl(PR_SET_NAME,"UART1");
printf("starting...%d\n",sizeof(uart_buffer_t));
//sleep(20); // test purpose , wait to change the uart plug
// create a test data
memcpy(buf_in.buf ,"aaaaaaaaaabbbbbbbbbb"
"cccccccccc1111111111dddddddddd"
"eeeeeeeeee12345678901234567890"
"12345678901234567890",UART_BUFFER_SIZE );
buf_in.len = UART_BUFFER_SIZE;
// open serial device
fd=open(DEVICE_FILE,O_RDWR | O_NOCTTY );
// flush old input and output data if exists
tcflush(fd,TCIOFLUSH);
set_port(fd);
puts("uartTask1 started");
while(1)
{
int s;
nanosleep(&waitTime,NULL);
msgCount++;
buf_in.msgNum = msgCount;
buf_in.checksum = checksum(buf_in.buf,UART_BUFFER_SIZE);
//send message
s=write(fd,&buf_in,sizeof(uart_buffer_t));
//wait for message to arrive
read(fd,&buf_out,sizeof(uart_buffer_t));
if(++count == 400)
{
//dump arrived message
printf("Got Msg number=%d from UART\n=============\n",buf_out.msgNum);
puts((char*)buf_out.buf);
if(checksum(buf_out.buf,buf_out.len) == buf_out.checksum)
{
puts("message ok");
}
else
{
puts("error in checksum");
}
count = 0;
}
}
}
Host side
/*
============================================================================
Name : PcUARTApp.c
Author :
Version :
Copyright : Your copyright notice
Description : Hello World in C, Ansi-style
============================================================================
*/
/*
* uartTask1.c
*
* Created on: May 15, 2010
* Author: root
*/
#include<unistd.h>
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<time.h>
#include <sys/prctl.h>
#include <fcntl.h>
#include <termios.h>
#pragma pack(1)
typedef struct {
int msgNum;
unsigned char buf[100];
short int len;
short int checksum;
}buffer_t;
#pragma pack()
struct termios options;
void set_port(int fd)
{
int status;
// Get current settings for the port
tcgetattr(fd, &options);
// Set baud rate = 1200
// Set 8 bits, no parity
options.c_cflag = B115200 | CRTSCTS | CS8 | CLOCAL | CREAD;
// Check and strip parity bit.
options.c_iflag &= ~(BRKINT | ICRNL | INPCK | ISTRIP | IXON);
options.c_oflag &= (~OPOST);
options.c_lflag &= ~(ECHO | ICANON | IEXTEN | ISIG);
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = sizeof(buffer_t);
// Set new options for port
status = tcsetattr(fd, TCSANOW, &options);
}
int main()
{
int b;
int fd;
buffer_t buf1;
printf("starting...%d\n",sizeof(buffer_t));
fd=open("/dev/ttyS0",O_RDWR | O_NOCTTY );
set_port(fd);
tcflush(fd,TCIOFLUSH);
puts("start ......");
while(1)
{
b=read(fd,&buf1,sizeof(buffer_t));
if(b > 0)
{
printf("Msg number=%d\n=============\n",buf1.msgNum);
puts(buf1.buf);
write(fd,&buf1,sizeof(buffer_t));
}
}
}
Recent Stories
Top DiscoverSDK Experts
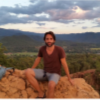
Mendy Bennett
Experienced with Ad network & Ad servers.
Mobile | Ad Networks and 1 more
View Profile
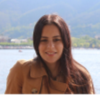
Karen Fitzgerald
7 years in Cross-Platform development.
Mobile | Cross Platform Frameworks
View Profile
X
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}Now comparing:
{{product.ProductName | createSubstring:25}} X
{{CommentsModel.TotalCount}} Comments
Your Comment