Proc FS
/*
* $Id: hello2.c,v 1.8 2001/07/18 22:28:18 rubini Exp $
*/
#include <linux/init.h>
#include <linux/module.h>
#include <linux/moduleparam.h>
#include <linux/kernel.h> /* printk() */
#include <linux/proc_fs.h>
#include <asm/uaccess.h>
#define MYNAME "driver/my_proc_file"
static struct proc_dir_entry *myproc = NULL;
static char msg[255] = "hello";
module_param_string(msg,msg,255,0);
int myreadtest(char *page,char **start,off_t off,int count,int *eof,void *data)
{
printk("page=%x off=%d count=%d \n",page,off,count);
int len=sprintf(page+off -1,"msg=%s\n",msg);
printk("len after=%d\n",len);
if(len <= off+count)
*eof = 1;
*start = page +off;
len-=off;
if(len > count) len=0;
if(len < 0) len=0;
printk("len=%d\n",len);
return len;
}
int myread(char *page,char **start,off_t off,int count,int *eof,void *data)
{
int len =0;
if(off <= 0){
len=sprintf(page,"msg=%s\n",msg);
}
return len;
}
int mywrite(struct file *file, const char *buffer, unsigned long count,
void *data)
{
printk("buffer=%x\n",buffer);
if ( copy_from_user(msg, buffer, count) ) {
return -EFAULT;
}
strcpy(msg+count,data);
msg[count] = 0;
return count;
}
static int
hello_init (void)
{
printk (KERN_INFO "Hello loaded sucessfuly.\n");
myproc = create_proc_entry(MYNAME,0,NULL);
myproc->read_proc = myreadtest;
myproc->write_proc = mywrite;
myproc->data = "custom data";
return 0;
}
static void
hello_cleanup (void)
{
printk (KERN_INFO "hello unloaded succefully.\n");
remove_proc_entry(MYNAME, NULL);
}
module_init (hello_init);
module_exit (hello_cleanup);
MODULE_LICENSE("Dual BSD/GPL");
Recent Stories
Top DiscoverSDK Experts
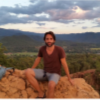
Mendy Bennett
Experienced with Ad network & Ad servers.
Mobile | Ad Networks and 1 more
View Profile
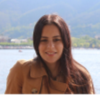
Karen Fitzgerald
7 years in Cross-Platform development.
Mobile | Cross Platform Frameworks
View Profile
X
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}Now comparing:
{{product.ProductName | createSubstring:25}} X
{{CommentsModel.TotalCount}} Comments
Your Comment