Operators And Control Structures In PHP
Arithmetic Operators:
Arithmetic operators are built-in mathematical actions in PHP. There are the four basic actions: ‘+’ for addition, ‘-’ for subtraction, ‘*’ for multiplication, and ‘/’ for division. There are also other mathematical actions, such as:
Modulus (‘%’), which is meant to return the remainder of a number divided by another number. For example:
<?php
echo 10 % 4;
?>
And the output will be:
2
One of the uses for Modulus in a program is to determine whether a number is odd or even, by using Modulus by 2 (Number % 2) - if the result is 1 the number is odd, and if the result is 0 then the number is even.
Another arithmetic action we have in PHP is the Exponentiation (‘**’), which takes a number and puts it to the a power of another number. For example:
<?php
echo 3 ** 3;
?>
And the output will be:
27
Exponentiation can also handle large numbers, and also floating numbers.
Incrementing/Decrementing Operators:
The incrementing operator (‘++’) increases a variable’s value (in case it is numerical - integer or floating) by 1. For example:
<?php
$VariableName = 3;
$VariableName++;
echo $VariableName;
?>
And the output will be:
4
The decrementing operator (‘--’) decreases a variable’s value (in case it is numerical - integer or floating) by 1. For example:
<?php
$VariableName = 3;
$VariableName--;
echo $VariableName;
?>
And the output will be:
2
In both incrementing and decrementing operators the sign can be written before or after the variable’s name.
We can also print the variable with the incrementing/decrementing operator together. For example:
<?php
$VariableName = 5;
echo ++$VariableName;
?>
And the output will be:
6
Keep in mind that is this way the incrementing/decrementing operator must be before the variable, or it will change the value only after it was printed.
Assignment Operators:
The idea behind assignment operators is being able to avoid constantly using the same variable name. For example, if we want to increase a variable’s numerical value by more than 1, we’ll do it this way:
<?php
$VariableName = 7;
$VariableName += 6;
echo $VariableName;
?>
And the output will be:
13
This method works with subtraction, multiplication, division, modulus and exponentiation as well.
String Operator:
In order to concatenate two strings into a single variable, we’ll use a string operator. It is done in the following syntax $VariableName .= “NewString”;. For example:
<?php
$VariableName = ‘Nathan;
$VariableName .= “ Drake”;
echo $VariableName;
?>
And the output will be:
Nathan Drake
Comparison Operators:
Using comparison operators lets us compare between two values at the same time, using the boolean command ‘var_dump()’.
The ‘Equal’ comparison operator - meant for checking if two values are equal to each other:
<?php
var_dump(8 == “8”);
?>
And the output will be:
bool(true)
The ‘Identical’ comparison operator - meant for checking if two values are equal and of the same type:
<?php
var_dump(8 === “8”);
?>
And the output will be:
bool(false)
The ‘Not Equal’ comparison operator is meant for checking of two value differ from each other. It can be written in two ways.
The first way:
<?php
var_dump(16 <> 9);
?>
And the second way:
<?php
var_dump(16 != 9);
?>
In both cases, the output will be:
bool(true)
The ‘Not Identical’ comparison operator, just like the ‘Identical’ comparison operator, compares the types of two values. But unlike the latter, the former is meant for checking if two given values are not identical. For example:
<?php
var_dump(20 !== “20”);
?>
And the output will be:
bool(true)
The ‘Greater Than’ comparison operator is meant for checking of the value on the left is greater than the one on the right. For example:
<?php
var_dump(3 > 3);
?>
And the output will be:
bool(false)
The ‘Greater Than Or Equal’ To is meant for checking if the value on the left is greater than or equal to the one on the right. For example:
<?php
var_dump(3 >= 3);
?>
And the output will be:
bool(true)
The ‘Less Than’ comparison operator is meant for checking if the value on the left is less than the one on the right. For example:
<?php
var_dump(18 < 17);
?>
And the output will be:
bool(false)
The ‘Less Than Or Equal To’ comparison operator the meant for checking if the value on the left is less than or equal to the one on the right. For example:
<?php
var_dump(18 <= 17);
?>
And the output will be:
bool(false)
Spaceship Operator:
The spaceship operator is also a comparison operator, but is different from the one above. Unlike the previous comparison operators which return a boolean value, the spaceship comparison operator returns one of the following values: -1, 0, 1. The spaceship comparison operator doesn’t use the ‘var_dump()’ command, unlike the previous comparison operators. The spaceship comparison operator is meant for checking whether the value on the left is greater, equal to or less than the one on the right. For example:
<?php
echo 14 <=> 14;
?>
And the output will be:
0
Another example:
<?php
echo 13 <=> 14;
?>
And the output will be:
-1
One more example:
<?php
echo 14 <=> 13;
?>
And the output will be:
1
As we can see, the returned result will be ‘-1’ if the value on the right is greater, ‘1’ if the value on the left is greater, and ‘0’ if both values are equal.
Logical Operators:
Logical operators are meant for checking if one condition, or more, are met - depends on what we define.
The ‘And’ logical operator is meant for checking if two given conditions are met at the same time. There are two syntaxes for this operator.
The first syntax (‘and’):
<?php
$VariableName = (13 < 14);
$VariableName2 = (16 == 16);
var_dump($VariableName and $VariableName2);
?>
And the second syntax (‘&&’):
<?php
$VariableName = (13 < 14);
$VariableName2 = (16 == 16);
var_dump($VariableName && $VariableName2);
?>
In both cases, the output will be:
bool(true)
Keep in mind that the returned result will be ‘bool(true)’ only when both conditions are met.
The ‘Or’ logical operator is meant for checking if at least one out of given conditions is met. There are two syntaxes for this operator.
The first syntax (‘or’):
<?php
$VariableName = (13 < 14);
$VariableName2 = (16 == 15);
var_dump($VariableName or $VariableName2);
?>
And the second syntax (‘||’):
<?php
$VariableName = (13 < 14);
$VariableName2 = (16 == 15);
var_dump($VariableName || $VariableName2);
?>
In both cases, the output will be:
bool(true)
Keep in mind that the returned result will be ‘bool(false)’ only when both conditions are not met.
The ‘Not’ logical operator gets a variable’s value (it must be a boolean value) and return the opposite value. For example:
<?php
$VariableName = (13 < 14);
$VariableName2 = (16 == 15);
var_dump(!$VariableName);
?>
And the output will be:
bool(false)
If Statement:
The ‘If’ statement’s job is to execute a certain block of code, but only if a certain condition is met. The ‘If’ statement is written in the following syntax:
<?php
If (YourCondition)
{
YourAction
}
?>
For example:
<?php
$Singers = [“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”, “Bono”];
$Count = count($Singers);
If($Count > 0)
{
echo “There is a total of ”.$Count.” singer(s).”;
}
?>
And the output will be:
There is a total of 6 singer(s).
Keep in mind that the block of code will execute only if the given condition is met. Otherwise, the block of code inside the ‘If’ statement will be skipped, and nothing will happen.
After the ‘If’ statement, we can add an ‘Else’ statement. The ‘Else’ statement is meant for executing in cases which in the condition given in the ‘If’ statement above it were not met. The ‘Else’ statement if written in the following syntax:
else
{
YourActions
}
For example:
<?php
$Singers = [“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”, “Bono”];
$Singers = [];
$Count = count($Singers);
If($Count > 0)
{
echo “There is a total of ”.$Count.” singer(s).”;
}
else
{
echo “There are no singers.”;
}
?>
And the output will be:
There are no singers.
Else If:
The ‘Else If’ statement is a combination between the ‘If’ and the ‘Else’ statements. It is meant to provide an additional alternate condition in cases which in the one in the ‘If’ statement was not met. It is written in the following syntax:
elseif(YourCondition)
{
YourActions
}
For example:
<?php
$Singers = [“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”, “Bono”];
$Count = count($Singers);
If($Count == 1)
{
echo “There is 1 Singer.”;
}
elseif($Count > 1)
{
echo “There is a total of ”.$Count.” singers.”;
}
else
{
echo “There are no singers.”;
}
?>
And the output will be:
There is a total of 6 Singers.
Keep in mind that you can chain many ‘Else If’ statements as you want - there is no limitation.
Switch Statement:
The ‘Switch’ statement is like an if-else system, but it has a few differences. The ‘Switch’ statement is used in the following syntax:
switch($VariableName)
{
case DesiredValue:
YourActions
break;
case DesiredValue2:
YourActions
break;
default:
YourActions
break;
}
For example:
<?php
$Singers = [“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”, “Bono”];
$Singers = [“Freddie Mercury”];
$Count = count($Singers);
switch($Count)
{
case 0:
echo “There are no singers.”.PHP_EOL;
break;
case 1:
echo “There is 1 Singer.”.PHP_EOL;
break;
default:
echo “There is a total of ”.$Count.” singers.”.PHP_EOL;
break;
}
?>
And the output will be:
There is 1 Singer.
Keep in mind that we can remove the ‘break;’ mark to let the ‘Switch’ statement run the given actions for each match it finds. For example:
<?php
$Singers = [“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”, “Bono”];
$Singers = [];
$Count = count($Singers);
switch($Count)
{
case 0:
echo “There are no singers.”.PHP_EOL;
case 1:
echo “There is 1 Singer.”.PHP_EOL;
default:
echo “There is a total of ”.$Count.” singers.”.PHP_EOL;
}
?>
And the output will be:
There are no singers.
There is 1 Singer.
There is a total of 0 singers.
The ‘Switch’ statement can also work with the ‘Spaceship’ comparison operator. For example:
<?php
switch (3 <=> 8)
{
case 1:
echo “Greater Than”;
break;
case 0:
echo “Equal”;
break;
case -1:
echo “Less Than”;
break;
}
?>
And the output will be:
Less Than
Ternary Operator:
The ternary operator is a one-lined replacement for a short and simple If-Else system, which saves the result into a variable. The ternary is used in the following syntax:
$VariableName = (YourCondition) ? ValueIfTrue : ValueIfFalse;. For example:
<?php
$Singers = [“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”, “Bono”];
$Count = count($Singers);
$Outcome = ($Count > 0) ? “Singer Total: “.$Count : “No Singers”;
echo $Outcome;
?>
And the output will be:
Singer Total: 6
Null Coalesce Operator:
The null coalesce operator makes the ternary operator even simpler. It is written in the following syntax: $VariableName = $VariableName2 ?? ValueIfFalse;. The meaning of this code line is that if the second variable’s value is set, then return the value, otherwise return the other result. For example:
<?php
$Singers = [“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”, “Bono”];
$Count = count($Singers);
$Outcome = $Count ?? “Count unavailable”;
echo $Outcome;
?>
And the output will be:
6
Keep in mind that you can chain as many variables as you want. The null coalesce operator will check every one of them one by one until it finds a set value or reaches the default value. For example:
<?php
$Singers = [“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”, “Bono”];
$Outcome = $Count ?? $VariableName2 ?? “Count unavailable”;
echo $Outcome;
?>
And the output will be:
Count unavailable
The null coalesce operator is good for testing variables for defined/undefined values, and also for handling data that comes from web pages.
While Loop:
The ‘While’ loop runs the block of code in it over and over as long as a certain condition is met, and ceases to run it and continues onward with the script when the given condition is no longer met. It is written in the following syntax:
while(YourCondition)
{
YourActions
VariableValueChange
}
For example:
<?php
$MusicIsawesome = true;
while($MusicIsawesome)
{
echo “Music Is awesome! :)”.PHP_EOL;
$MusicIsawesome = false;
}
?>
And the output will be:
Music Is awesome! :)
Another example:
<?php
$Number = 0;
while($Number < 6)
{
echo “Music Is awesome! :)”.PHP_EOL;
$Number++;
}
?>
And the output will be:
Music is awesome! :)
Music is awesome! :)
Music is awesome! :)
Music is awesome! :)
Music is awesome! :)
Music is awesome! :)
We can remove the ‘$Number++;’ line from the script, but that will cause an infinite loop.
For Loop:
The ‘For’ loop is meant to make the ‘While’ loop’s syntax require less lines of code. It is written in the following syntax:
for(Variable; Condition; VariableValueChange)
{
YourActions
}
For example:
<?php
for($Number = 0; $Number < 6; $Number++)
{
echo "Music is awesome!".PHP_EOL;
}
?>
And the output will be:
Music is awesome!
Music is awesome!
Music is awesome!
Music is awesome!
Music is awesome!
Music is awesome!
Alternate Syntax For Control Structures:
For each control structure the is another syntax, which can replace the curly brackets which was used in the previous parts of this article. In the alternate syntax, each starting line end with a colon (‘:’) sign, and the replacing word will be one of the following: ‘endfor’, ‘endwhile’, ‘endif’, ‘endforeach’, and ‘endswitch’ (depends on which control structure is used). For example:
<?php
$MusicIsAwesome = true;
$Singers = ["Freddie Mercury", "Axl Rose", "Michael Jackson", "Mick Jagger", "Sting", "Bono"];
$Count = count($Singers);
if($Count > 0) :
echo "There is a total of ".$Count." singer(s).".PHP_EOL;
else :
echo "There are no singers.".PHP_EOL;
endif;
while($MusicIsAwesome) :
echo "Music is awesome! :)".PHP_EOL;
$MusicIsAwesome = false;
endwhile;
for($i = 1; $i <= 6; $i++) :
echo "Music is awesome! :)".PHP_EOL;
endfor;
?>
And the output will be:
There is a total of 6 singer(s).
Music is awesome! :)
Music is awesome! :)
Music is awesome! :)
Music is awesome! :)
Music is awesome! :)
Music is awesome! :)
Music is awesome! :)
There are two benefits in using this syntax for control structures. The first and less important benefit is getting a simpler look for the control structures, and the second and more important benefit is better compatibility when using the PHP along with HTML codes.
Recent Stories
Top DiscoverSDK Experts
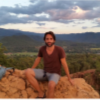
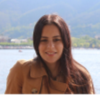
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment