Classes And Objects In PHP
A Few Words First:
A class is sort of a container, meant to make programming easier and more efficient.
Creating A Class:
In order to crease a class in PHP, we use the following syntax:
<?php
Class YourClassName
{
}
?>
Creating An Object Inside A Class:
An object is a variable which has access to the class. In order to create an object inside a class in PHP, we use the following syntax:
$VariableName = new YourClassName();. For example:
<?php
class YourClassName
{
}
$VariableName = new YourClassName();
?>
Creating Properties:
Properties are variables which are associated with the class’s subject, and are declared at the top of the class. Properties can be declared as public, private and protected. For example:
<?php
class Uncharted4
{
public $Franchise = “Uncharted”;
public $YearOfRelease = 2016;
public $Genre = “Action”;
}
$Object = new Uncharted4();
?>
As you can see in the code example above, it is also possible to define an initial value for each of the variables inside the class.
Accessing Properties Inside A Class:
In order to access properties inside our class, we need to use the variable which has access to to the contents inside the class. It is done in the following syntax:
$VariableName->VariableInsideClass. For example:
<?php
class Uncharted4
{
public $Franchise = “Uncharted (Series)”;
public $YearOfRelease = 2016;
public $Genre = “Action”;
}
$Object = new Uncharted4();
echo $Object->Franchise;
?>
And the output will be:
Uncharted (Series)
Public properties can also be overwritten:
<?php
class Uncharted4
{
public $Franchise = “Uncharted (Series)”;
public $YearOfRelease = 2016;
public $Genre = “Action”;
}
$Object = new Uncharted4();
echo $Object->Franchise.”\n”;
echo $Object->Franchise = “Whatever...”;
?>
And the output will be:
Uncharted (Series)
Whatever...
Creating Constants Inside Of A Class:
Constants are data types which their value cannot be changed during the program once defined. These are being used for setting limits to the program more efficiently, while also making the code easier to read. To make a constant’s name easier to recognize as one, all the letters in the name will be written in capital letters and the words will be divided from each other by an underline. In PHP, we define constants using this syntax:
const YOUR_CONSTANT_NAME = DesiredValue;. For example:
<?php
class Uncharted4
{
const PLATFORMS_OF_RELEASE = “Playstation 4”;
public $Franchise = “Uncharted (Series)”;
public $YearOfRelease = 2016;
public $Genre = “Action”;
}
$Object = new Uncharted4();
?>
Accessing Constants Which are Inside Of A Class:
There are two ways to access a constant that is inside a class.
The first way is by using the variable with the access to the class, along with a double colon sign (‘::’), in this syntax: $VariableName::YOUR_CONSTANT_NAME;. For example:
<?php
class Uncharted4
{
const PLATFORMS_OF_RELEASE = “Playstation 4”;
public $Franchise = “Uncharted (Series)”;
public $YearOfRelease = 2016;
public $Genre = “Action”;
}
$Object = new Uncharted4();
echo $Object::PLATFORMS_OF_RELEASE;
?>
The second way is just like the first one, but we use the class name instead of the variable. For example:
<?php
class Uncharted4
{
const PLATFORMS_OF_RELEASE = “Playstation 4”;
public $Franchise = “Uncharted (Series)”;
public $YearOfRelease = 2016;
public $Genre - “Action”;
}
$Object = new Uncharted4();
echo Uncharted4::PLATFORMS_OF_RELEASE;
?>
In both cases the output will be:
Playstation 4
Creating Methods:
Methods are functions inside of a class, and are used for the same purposes as functions. Methods also have the same syntax as functions:
function FunctionName()
{
YourActions
}
For example:
<?php
class Uncharted4
{
const PLATFORMS_OF_RELEASE = “Playstation 4”;
public $Franchise = “Uncharted (Series)”;
public $YearOfRelease = 2016;
public $Genre = “Action”;
public function GetFranchise()
{
}
public function SetFranchise($TempFranchise)
{
}
}
$Object = new Uncharted4();
?>
Accessing And Setting The Value Of A Property:
In order to access and set a property’s value, we’ll use the pseudo-variable ‘$this’. For example:
<?php
class Uncharted4
{
const PLATFORMS_OF_RELEASE = “Playstation 4”;
public $Franchise = “Uncharted (Series)”;
public $YearOfRelease = 2016;
public $Genre = “Action”;
public function GetFranchise()
{
return $this->Franchise;
}
public function SetFranchise($TempFranchise)
{
$this->Franchise = $TempFranchise;
}
}
$Object = new Uncharted4();
?>
Accessing Methods:
In order to access the methods in a class, we’ll use the variable with the access to it, and use the methods through it. For example:
<?php
class Uncharted4
{
const PLATFORMS_OF_RELEASE = “Playstation 4”;
public $Franchise = “Uncharted (Series)”;
public $YearOfRelease = 2016;
public $Genre = “Action”;
public function GetFranchise()
{
return $this->Franchise;
}
public function SetFranchise($TempFranchise)
{
$this->Franchise = $TempFranchise;
}
}
$Object = new Uncharted4();
$Object->SetFranchise(“God Of War”);
echo $Object->Franchise;
?>
We can also access the value through the ‘GetFranshice’ method instead of the property itself:
<?php
class Uncharted4
{
const PLATFORMS_OF_RELEASE = “Playstation 4”;
public $Franchise = “Uncharted (Series)”;
public $YearOfRelease = 2016;
public $Genre = “Action”;
public function GetFranchise()
{
return $this->Franchise;
}
public function SetFranchise($TempFranchise)
{
$this->Franchise = $TempFranchise;
}
}
$Object = new Uncharted4();
$Object->SetFranchise(“God Of War”);
echo $Object->GetFranchise();
?>
In both ways the output will be:
God Of War
Constructors:
Constructors are functions for the automation of the initialization of an object, when one is created. Constructors are created in the following syntax:
Function __construst();
{
YourActions
}
For example:
<?php
class Uncharted4
{
const PLATFORMS_OF_RELEASE = “Playstation 4”;
public $Franchise = “Uncharted (Series)”;
public $YearOfRelease = 2016;
public $Genre = “Action”;
function __construst()
{
echo “A Thief’s End”;
}
public function GetFranchise()
{
return $this->Franchise;
}
public function SetFranchise($TempFranchise)
{
$this->Franchise = $TempFranchise;
}
}
$Object = new Uncharted4();
?>
And the output will be:
A Thief’s End
Keep in mind that before the ‘construct’ there is a double underline.
We can also use constructors to set values to properties inside the class. For example:
<?php
class Uncharted4
{
const PLATFORMS_OF_RELEASE = “Playstation 4”;
public $Franchise;
public $YearOfRelease;
public $Genre;
function __construst()
{
$this->Franchise = “Uncharted (Series)”;
$this->YearOfRelease = 2016;
$this->Genre = “Action”;
}
public function GetFranchise()
{
return $this->Franchise;
}
public function SetFranchise($TempFranchise)
{
$this->Franchise = $TempFranchise;
}
}
$Object = new Uncharted4();
echo $Object->GetFranchise();
?>
And the output will be:
Uncharted (Series)
We can also print the parameter itself instead of the method.
Passing Initial Values:
In order to pass initial values into a constructor, we should first set properties inside of the constructor, and then pass default values to them:
<?php
class Uncharted4
{
const PLATFORMS_OF_RELEASE = “Playstation 4”;
public $Franchise;
public $YearOfRelease;
public $Genre;
function __construst($TempFranchise - “”, $TempYearOfRelease = “”, $TempGenre = “”)
{
$this->Franchise = $TempFranchise;
$this->YearOfRelease = $TempYearOfRelease;
$this->Genre = $TempGenre;
}
public function GetFranchise()
{
return $this->Franchise;
}
public function SetFranchise($TempFranchise)
{
$this->Franchise = $TempFranchise;
}
}
$Object = new Uncharted4(“Uncharted (Series)”, 2016, “Action”);
echo $Object->GetFranchise();
?>
And the output will be:
Uncharted (Series)
Inheritance:
The meaning of inheritance is when a class can inherit all of the methods and properties of another class. In this way we can add the things we want in the child class, without it appearing in the parent class as well. In the child class’s title we add ‘extends ParentClassName’. For example:
<?php
class Person
{
const AVG_LIFE_SPAN = 79;
public $firstName;
public $lastName;
public $yearBorn;
function __construct($tempFirst = "", $tempLast = "", $tempYear = "")
{
echo "Person Constructor".PHP_EOL;
$this->firstName = $tempFirst;
$this->lastName = $tempLast;
$this->yearBorn = $tempYear;
}
public function getFirstName()
{
return $this->firstName.PHP_EOL;
}
public function setFirstName($tempName)
{
$this->firstName = $tempName;
}
public function getFullName()
{
echo "Person->getFullName()".PHP_EOL;
return $this->firstName." ".$this->lastName.PHP_EOL;
}
}
class Author extends Person
{
public $penName = "Mark Twain";
public function getPenName()
{
return $this->penName.PHP_EOL;
}
public function getFullName()
{
echo "Author->getFullName()".PHP_EOL;
return $this->firstName." ".$this->lastName.PHP_EOL;
}
}
$newAuthor = new Author("Samuel Langhorne", "Clemens", 1899);
echo $newAuthor->getFullName();
?>
And the output will be:
Person Constructor
Author->getFullName()
Samuel Langhorne Clemens
As you can see, if the child class has methods with identical names as some methods in the parent class, the former will overwrite the latter’s data with its own. Also, ‘.PHP_EOL’ is just like ‘\n’, as they both do the same thing.
Protected Properties:
Unlike public properties, protected properties/methods can only be accessed from within the class. If we try to access a protected property/method from outside the class which it is stored in, we will get an error message. But, there is a way to access a protected property/method from outside its class - using a public method is able to do so. For example:
<?php
class Person
{
const AVG_LIFE_SPAN = 79;
protected $firstName;
protected $lastName;
protected $yearBorn;
function __construct($tempFirst = "", $tempLast = "", $tempYear = "")
{
$this->firstName = $tempFirst;
$this->lastName = $tempLast;
$this->yearBorn = $tempYear;
}
public function getFirstName()
{
return $this->firstName.PHP_EOL;
}
public function setFirstName($tempName)
{
$this->firstName = $tempName;
}
protected function getFullName()
{
return $this->firstName." ".$this->lastName.PHP_EOL;
}
}
class Author extends Person
{
protected $penName = "Mark Twain";
public function getPenName()
{
return $this->penName.PHP_EOL;
}
public function getCompleteName()
{
return $this->firstName." ".$this->lastName." a.k.a ".$this->penName.PHP_EOL;
}
}
$newAuthor = new Author("Samuel Langhorne", "Clemens", 1899);
echo $newAuthor->getCompleteName();
?>
And the output will be:
Samuel Langhorne Clemens a.k.a Mark Twain
Private Properties:
Private properties/methods cannot be found by functions outside their class - as if they were never declared. In order to access them, we need to call a function which resides in the same class as the desired private properties/methods. For example:
<?php
class Person
{
const AVG_LIFE_SPAN = 79;
private $firstName;
private $lastName;
private $yearBorn;
function __construct($tempFirst = "", $tempLast = "", $tempYear = "")
{
$this->firstName = $tempFirst;
$this->lastName = $tempLast;
$this->yearBorn = $tempYear;
}
public function getFirstName()
{
return $this->firstName.PHP_EOL;
}
public function setFirstName($tempName)
{
$this->firstName = $tempName;
}
protected function getFullName()
{
return $this->firstName." ".$this->lastName.PHP_EOL;
}
}
class Author extends Person
{
private $penName = "Mark Twain";
public function getPenName()
{
return $this->penName.PHP_EOL;
}
public function getCompleteName()
{
return $this->getFullName()." a.k.a ".$this->penName.PHP_EOL;
}
}
$newAuthor = new Author("Samuel Langhorne", "Clemens", 1899);
echo $newAuthor->getCompleteName();
?>
And the output will be:
Samuel Langhorne Clemens
a.k.a Mark Twain
Static Properties And Methods:
Static properties and methods don’t depend on other objects or influenced by them. A static property has to have an initial value set, and both static methods and properties need to be self-contained - they must rely on nothing (including other classes, methods or properties) but themselves and/or other static properties/methods, in order to achieve their coding tasks. Static methods/properties are written in the following syntax:
DesiredClassification static MethodOrPropertyRegularSyntax;. For exapmple:
<?php
class Person
{
const AVG_LIFE_SPAN = 79;
private $firstName;
private $lastName;
private $yearBorn;
function __construct($tempFirst = "", $tempLast = "", $tempYear = "")
{
$this->firstName = $tempFirst;
$this->lastName = $tempLast;
$this->yearBorn = $tempYear;
}
public function getFirstName()
{
return $this->firstName.PHP_EOL;
}
public function setFirstName($tempName)
{
$this->firstName = $tempName;
}
protected function getFullName()
{
return $this->firstName." ".$this->lastName.PHP_EOL;
}
}
class Author extends Person
{
public static $centuryPopular = "19th";
private $penName = "Mark Twain";
public function getPenName()
{
return $this->penName.PHP_EOL;
}
public function getCompleteName()
{
return $this->getFullName()." a.k.a ".$this->penName.PHP_EOL;
}
public static function getCenturyAuthorWasPopular()
{
}
}
?>
Accessing Static Properties/Methods:
In order to access a static property/method, well write the name of the class with a double colon sign (‘::’) right after it, and then the static property/method which we want to access. The syntax is: ClassName::StaticPropertyOrMethod;. For example:
<?php
class Person
{
const AVG_LIFE_SPAN = 79;
private $firstName;
private $lastName;
private $yearBorn;
function __construct($tempFirst = "", $tempLast = "", $tempYear = "")
{
$this->firstName = $tempFirst;
$this->lastName = $tempLast;
$this->yearBorn = $tempYear;
}
public function getFirstName()
{
return $this->firstName.PHP_EOL;
}
public function setFirstName($tempName)
{
$this->firstName = $tempName;
}
protected function getFullName()
{
return $this->firstName." ".$this->lastName.PHP_EOL;
}
}
class Author extends Person
{
public static $centuryPopular = "19th";
private $penName = "Mark Twain";
public function getPenName()
{
return $this->penName.PHP_EOL;
}
public function getCompleteName()
{
return $this->getFullName()." a.k.a ".$this->penName.PHP_EOL;
}
public static function getCenturyAuthorWasPopular()
{
}
}
echo Author::$centuryPopular;
?>
And the output will be:
19th
When we want to access a static property, we do have to use the dollar sign, but we don’t have to do so when we want to access a static method.
If we want to return a static property using a static method, we’ll have to add the word ‘self’ before the ‘::’ sign, in the following syntax: return self::$PropertyName;. For example:
<?php
class Person
{
const AVG_LIFE_SPAN = 79;
private $firstName;
private $lastName;
private $yearBorn;
function __construct($tempFirst = "", $tempLast = "", $tempYear = "")
{
$this->firstName = $tempFirst;
$this->lastName = $tempLast;
$this->yearBorn = $tempYear;
}
public function getFirstName()
{
return $this->firstName.PHP_EOL;
}
public function setFirstName($tempName)
{
$this->firstName = $tempName;
}
protected function getFullName()
{
return $this->firstName." ".$this->lastName.PHP_EOL;
}
}
class Author extends Person
{
public static $centuryPopular = "19th";
private $penName = "Mark Twain";
public function getPenName()
{
return $this->penName.PHP_EOL;
}
public function getCompleteName()
{
return $this->getFullName()." a.k.a ".$this->penName.PHP_EOL;
}
public static function getCenturyAuthorWasPopular()
{
return self::$centuryPopular;
}
}
echo Author::getCenturyAuthorWasPopular();
?>
And the output will be:
19th
One more thing we need to remember is when we want to access from an inherited class a static property/method that is stored in a parent class, we will write the word ‘parent’ instead of the ‘self’ word.
Include File:
To include another file in out PHP script, we’ll write the word ‘include’, followed by the desired file’s name (with its extension) with single or double quotes before and after it (it doesn’t matter which). It will be done in the following syntax: include ‘DesiredFileNameWithExtension’;. For example:
<?php
include ‘Person.php’;
include ‘Author.php’;
$newAuthor = new Author("Samuel Langhorne", "Clemens", 1899);
echo $newAuthor->getCompleteName();
?>
If we want to include a file which has classes and function calls in it, it is better to use the ‘include_once’ command instead of the regular ‘include’ command:
<?php
include ‘Person.php’;
include_once ‘Author.php’;
$newAuthor = new Author("Samuel Langhorne", "Clemens", 1899);
echo $newAuthor->getCompleteName();
?>
If we try to use the ‘include_once’ command for the same file more than once, we’ll get an error message.
We can also use the ‘include’ command on links to files in the internet - we just have to make sure to give the correct path.
Require File:
The ‘require’ and ‘require_once’ commands work the same way and have the same syntax as the ‘include’ and ‘include_once’ commands. The difference is the behavior of the script with each of the commands, in case the file cannot be found in a particular location. When using ‘include’ and ‘include_once’ commands, we will receive two error messages, but besides that the rest of the script will keep running normally. When using the ‘require’ and ‘require_once’, we will get the same two error messages and nothing else, and the script will terminate in that point.
Recent Stories
Top DiscoverSDK Experts
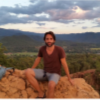
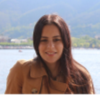
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment