Arrays In PHP
A Few Words First:
Arrays are collections of data types. They can contain any type, and all types together at the same time). In arrays, every value has a related key (key value pair). Arrays can be created with ‘array()’ or ‘array[]’.
Indexed Arrays:
In PHP, we can create an array and print it:
<?php
$VariableName = array(“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”);
print_r($VariableName);
?>
And the output will be:
Array
(
[0] => Freddie Mercury
[1] => Axl Rose
[2] => Michael Jackson
[3] => Mick Jagger
[4] => Sting
)
We can also define a variable as an array without using the word “array”. To do so, we just open a square parentheses (‘[]’) instead of the previous syntax, and put the values in it.
Associative Arrays:
In arrays, we can also replace the numbers of the keys with string values, It is done this way:
<?php
$VariableName = array(
“Shooter” => “Crysis”,
“RPG” => “The Legend Of Zelda”,
“Adventure” => “Pokemon”
);
print_r($VariableName);
?>
And the output will be:
Array
(
[Shooter] => Crysis
[RPG] => The Legend Of Zelda
[Adventure] => Pokemon
)
We can also assign integer numbers as keys, but there is a trick with it - every value in the array which doesn’t have a key manually assigned to it will get the following number to the last integer key before it. For example:
<?php
$VariableName = array(
“Shooter” => “Crysis”,
21 => “The Legend Of Zelda”,
“Adventure” => “Pokemon”,
“Uncharted”
);
print_r($VariableName);
?>
The output will be:
Array
(
[Shooter] => Crysis
[21] => The Legend Of Zelda
[Adventure] => Pokemon
[22] => Uncharted
)
Accessing The Values Inside An Array:
We can access any value in an array by its key assigned to it (0, 1, 2, 3...). To do so, we use the ‘echo’ command in the following syntax: echo ArrayVariableName[ValueKeyNumber];. For example:
<?php
$VariableName = array(“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”);
echo $VariableName[2];
?>
And The output will be:
Michael Jackson
Keep in mind that the counting starts from 0.
Another thing you should remember is that in order to display a value from an associative array, you’ll have to input the manually given value of the desired value, between single quotes (‘), in order for the command to work properly. For example:
<?php
$VariableName = array(
“Shooter” => “Crysis”,
“RPG” => “The Legend Of Zelda”,
“Adventure” => “Pokemon”
);
echo $VariableName[‘RPG’];
?>
And the output will be:
The Legend Of Zelda
Checking If A Certain Key Exists In The Array:
Sometimes we want to check of a certain key exists in a certain array. This is done in the following example: echo array_key_exists(DesiredKey, TargetArray);. For example:
<?php
$VariableName = array(“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”);
echo array_key_exists(6, $VariableName);
?>
And typically for a boolean, the output will be empty, as it’s value is “False”. But if, for example, we search an existing key, we should get the output “1”, as typical for a boolean variable with the value of “True”. For example:
<?php
$VariableName = array(“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”);
echo array_key_exists(2, $VariableName);
?>
And the output is indeed:
1
Keep in mind that this method works for both indexed and associative arrays.
Checking If A Certain Value Exists In The Array:
In order to check if a certain value exists in the array, we use the ‘in_array()’ command, in this syntax: in_array(DesiredValue, TargetArray, OptionalBooleanVariable);. For example:
<?php
$VariableName = array(
“Freddie Mercury”,
“Axl Rose”,
“Michael Jackson”,
“Mick Jagger”,
“Sting”,
);
echo in_array(“Mick Jagger”, $VariableName);
?>
And the output will be:
1
The third optional boolean variable is meant for finding out if the checked value is also of the same type as the identically typed value in the checked array. For example:
<?php
$VariableName = array(
“Freddie Mercury”,
“Axl Rose”,
“Michael Jackson”,
“Mick Jagger”,
“Sting”,
37
);
echo in_array(37, $VariableName, true);
?>
And the output will be:
1
Keep in mind that if, for example, we would write “37” instead of 37, the function wouldn’t return anything to display, because it would recognize the “37” as a string instead of an integer.
Adding New Items To An Array:
There are two methods for adding new items to an array. The first one is using the ‘array_push()’ command, in the following syntax: array_push(TargetArray, DesiredValue);. For example:
<?php
$VariableName = array(“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”);
array_push($VariableName, “Bono”);
print_r($VariableName);
?>
The second method is using square brackets (‘[]’), in the following syntax:
TargetArray[] = DesiredValue;. For example:
<?php
$VariableName = array(“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”);
$VariableName[] = “Bono”;
print_r($VariableName);
?>
In both cases, the output will be:
Array
(
[0] => Freddie Mercury
[1] => Axl Rose
[2] => Michael Jackson
[3] => Mick Jagger
[4] => Sting
[5] => Bono
)
The second method is preferred for use, because if the array has not been declared yet, it will automatically declare it, while with the first method a error message will be displayed.
Also, the ‘array_push()’ command will only add indexed values, while the square brackets will also work for associative values. This is how to add an associative value to an associative array:
<?php
$VariableName = array(
“Shooter” => “Crysis”,
“RPG” => “The Legend Of Zelda”,
“Adventure” => “Pokemon”
);
$VariableName[‘Action’] = “Uncharted”;
print_r($VariableName);
?>
And the output will be:
Array
(
[Shooter] => Crysis
[RPG] => The Legend Of Zelda
[Adventure] => Pokemon
[Action] = Uncharted
)
Removing A Value From An Array:
There are two methods in PHP for removing a value from an array.
The first method is using the ‘array_pop()’ command. In this method we can also save the remove value to a variable by the way. For example:
<?php
$VariableName = array(“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”);
$VariableName2 = array_pop($VariableName);
echo $VariableName2;
echo “\n”;
print_r($VariableName);
?>
And the output will be:
Sting
Array
(
[0] => Freddie Mercury
[1] => Axl Rose
[2] => Michael Jackson
[3] => Mick Jagger
)
Keep in mind that with this method we can only remove the last value from an array.
The second method is using the ‘unset()’ command, in the following syntex:
unset(TargetArray[KeyOfDesiredValue]);. For example:
<?php
$VariableName = array(“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”);
unset($VariableName[2]);
print_r($VariableName);
?>
And the output will be:
Array
(
[0] => Freddie Mercury
[1] => Axl Rose
[2] => Mick Jagger
[3] => Sting
)
Keep in mind that with this method we can choose the value in the array which we want to remove. Also remember that the counting starts from 0.
Also, the second method works for both indexed and associative arrays (so does the first one), and is also capable of handling multiple values and arrays at the same time (unlike the first method). For example:
<?php
$VariableName = array(“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”);
$VariableName2 = array(
“Shooter” => “Crysis”,
“RPG” => “The Legend Of Zelda”,
“Adventure” => “Pokemon”,
“Action” => “Uncharted”
);
unset($VariableName[3], $VariableName2[‘Adventure’]);
print_r($VariableName);
Echo “\n”;
print_r($VaroableName2);
?>
And the output will be:
Array
(
[0] => Freddie Mercury
[1] => Axl Rose
[2] => Michael Jackson
[4] => Sting
)
Array
(
[Shooter] => Crysis
[RPG] => The Legend Of Zelda
[Action] => Uncharted
)
Sorting Arrays:
There are three main methods for sorting arrays in PHP.
The first methods is using the ‘sort()’ command, to sort the values in a array alphabetically. For example:
And the output will be:
Array
(
[0] => Axl Rose
[1] => Freddie Mercury
[2] => Michael Jackson
[3] => Mick Jagger
[4] => Sting
)
Keep in mind that this method will also work for associative arrays, but during the process it will convert them to indexed arrays (replace the string values with integers).
The second method is using the ‘asort()’ command, to sort the values in an array alphabetically while preserving the key-value relationship. For example:
<?php
$VariableName = array(“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”);
asort($VariableName);
print_r($VariableName);
?>
And the output will be:
Array
(
[1] => Axl Rose
[0] => Freddie Mercury
[2] => Michael Jackson
[3] => Mick Jagger
[4] => Sting
)
This method is ideal for associative arrays, but not very practical for indexed arrays.
The third method is using the ‘’ command, to to sort the values in an array by key value while preserving the key-value relationship. For example:
<?php
$VariableName = array(
“Shooter” => “Crysis”,
“RPG” => “The Legend Of Zelda”,
“Adventure” => “Pokemon”,
“Action” => “Uncharted”
);
ksort($VariableName);
print_r($VariableName);
?>
And the output will be:
Array
(
[Action] => Uncharted
[Adventure] => Pokemon
[RPG] => The Legend Of Zelda
[Shooter] => Crysis
)
Counting The Number Of Elements In An Array:
In some cases, we need to count the elements in a certain array. For this purpose, PHP has the built-in ‘count()’ command, which is used in this syntax:
count(TargetArray, OptionalBooleanVariable);. For example:
<?php
$VariableName = array(“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”);
echo count($VariableName);
?>
And the output will be:
5
In some cases we need to count the elements in a multidimensional array (an array of arrays). For this purpose, we have an optional boolean variable. For example:
<?php
$VariableName = [
“Rock” => array(
“Queen” => array(“Bohemian Rhapsody”, “Love Of My Life”),
“Guns N’ Roses” => array(“Sweet Child O’ Mine”, “November Rain”),
“The Rolling Stones” => array(“Beast Of Burden”, “Angie”)
),
“Pop” => array(
“Michael Jackson” => array(“Billie Jean”, “Bad”),
“The Beach Boys” => array(“Good Vibrations”, “Wouldn’t It Be Nice”),
“Stevie Wonder” => array(“Isn’t She Lovely”, “I Was Made To Love Her”)
)
];
echo count($VariableName, 1);
?>
And the output will be:
20
Instead of the “1”, we can put the ‘COUNT_RECURSIVE’ PHP defined constant - We will get the same results.
Keep in mind that all PHP defined constants are written fully with uppercase letters.
Foreach Loops:
Foreach loops are designed especially for arrays, and are meant to perform a defined action on every element in the array. This is the syntax for foreach loops:
<?
$VariableName = array(“Value1”, “Value2”, “Value3”, “Value4”, “Value5”);
foreach(ArrayName as $VariableName2)
{
YourActions
}
?>
For example:
<?php
$VariableName = array(“Freddie Mercury”, “Axl Rose”, “Michael Jackson”, “Mick Jagger”, “Sting”);
Foreach ($VariableName as $VariableName2)
{
echo $VariableName2.”\n”;
}
?>
And the output will be:
Freddie Mercury
Axl Rose
Michael Jackson
Mick Jagger
Sting
There is another, optional variable, which is meant to use the keys in the array as well (in case we are using an associative array). It is placed in the syntax this way:
foreach(TargetArray as $KeyVariable => $VariableName). For example:
<?php
$VariableName = array(
“Shooter” => “Crysis”,
“RPG” => “The Legend Of Zelda”,
“Adventure” => “Pokemon”,
“Action” => “Uncharted”
);
foreach ($VariableName as $VariableName2 => $VariableName1)
{
Echo $VariableName1.” (“.$VariableName2.”)\n”;
}
?>
And the output will be:
Crysis (Shooter)
The Legend Of Zelda (RPG)
Pokemon (Adventure)
Uncharted (Action)
Multi-Dimensional Arrays:
Multi-Dimensional arrays are printed just like any other array - with the ‘print_r()’ command. For example:
<?php
$VariableName = [
“Rock” => array(
“Queen” => array(“Bohemian Rhapsody”, “Love Of My Life”),
“Guns N’ Roses” => array(“Sweet Child O’ Mine”, “November Rain”),
“The Rolling Stones” => array(“Beast Of Burden”, “Angie”)
),
“Pop” => array(
“Michael Jackson” => array(“Billie Jean”, “Bad”),
“The Beach Boys” => array(“Good Vibrations”, “Wouldn’t It Be Nice”),
“Stevie Wonder” => array(“Isn’t She Lovely”, “I Was Made To Love Her”)
)
];
print_r($VariableName);
?>
And the output will be:
Array
(
[Rock] => Array
(
[Queen] => Array
(
[0] => Bohemian Rhapsody
[1] => Love Of My Life
)
[Guns N’ Roses] => Array
(
[0] => Sweet Child O’ Mine
[1] => November Rain
)
[The Rolling Stones] => Array
(
[0] => Beast Of Burden
[1] => Angie
)
)
[Pop] => Array
(
[Michael Jackson] => Array
(
[0] => Billie Jean
[1] => Bad
)
[The Beach Boys] => Array
(
[0] => Good Vibrations
[1] => Wouldn’t It Be Nice
)
[Stevie Wonder] => Array
(
[0] => Isn’t She Lovely
[1] => I Was Made To Love Her
)
)
)
However, we are also able to access certain values in the array. The idea is just to put square brackets around every inner array you want to choose from. For example:
<?php
$VariableName = [
“Rock” => array(
“Queen” => array(“Bohemian Rhapsody”, “Love Of My Life”),
“Guns N’ Roses” => array(“Sweet Child O’ Mine”, “November Rain”),
“The Rolling Stones” => array(“Beast Of Burden”, “Angie”)
),
“Pop” => array(
“Michael Jackson” => array(“Billie Jean”, “Bad”),
“The Beach Boys” => array(“Good Vibrations”, “Wouldn’t It Be Nice”),
“Stevie Wonder” => array(“Isn’t She Lovely”, “I Was Made To Love Her”)
)
];
print_r($VariableName[‘Rock’][‘The Rolling Stones’][0]);
?>
And the output will be:
Beast Of Burden
Recent Stories
Top DiscoverSDK Experts
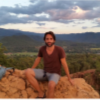
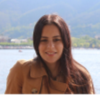
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment