Arbitrary-Length Integer Addition in Javascript
Note that this isn't the most efficient way to do it, but it is simple and fast to code, and the logic should be easy to understand.
/* Note that this reverses the order - intentional, to preserve place ordering
takes in an arbitrary length string (though limited to an int32 length in practise)
*/
var readNumFromString = function(s) {
return s.split("").map(Number).reverse();
};
/* adds two arrays created by readNumFromString */
var addNumArrays = function(a,b) {
let ret = [];
let carry = 0;
let i = 0;
/* shorter one first */
if (a.length > b.length)
return addNumArrays(b,a);
/* add up to the length of the shorter */
for (i = 0; i < a.length; i++)
{
let t = a[i] + b[i] + carry;
ret[i] = t % 10;
carry = (t > 9 ? 1 : 0);
}
/* handle the undefined values properly, since they don't cast */
while (i < b.length)
{
let t = b[i] + carry;
ret[i++] = t % 10;
carry = (t > 9 ? 1 : 0);
}
/* possible carry digit at the end */
if (carry == 1)
ret[i] = 1;
return ret;
};
/* turns the arrays back into strings */
var printNumToString = function(a) {
return a.reverse().join("");
};
Recent Stories
Top DiscoverSDK Experts
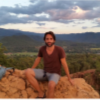
Mendy Bennett
Experienced with Ad network & Ad servers.
Mobile | Ad Networks and 1 more
View Profile
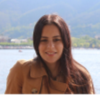
Karen Fitzgerald
7 years in Cross-Platform development.
Mobile | Cross Platform Frameworks
View Profile
X
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}Now comparing:
{{product.ProductName | createSubstring:25}} X
{{CommentsModel.TotalCount}} Comments
Your Comment