Algorithms Examples
Binary Search
#include <conio.h>
#include <stdio.h>
#define T_SIZE 5
#define NOTFOUND -1
int binary_search (int [], int, int);
void insert_values(int []);
void main()
{
int i,x=1;
int t[T_SIZE];
clrscr();
insert_values(t);
while (x!=0)
{ printf("\nEnter a search value (0 to stop) ==>");
scanf ("%d",&x);
i=binary_search(t,x,T_SIZE);
if (i == NOTFOUND )
printf("not found");
else
printf("found at location %d ", i);
}
}
/*-------------------------------------------------*/
/* binary search */
/*-------------------------------------------------*/
int binary_search(int v[] , int x, int v_size )
{
int u,m,l;
l=0;
u=v_size -1;
while (l<=u)
{ m=(u+l)/2;
if ( v[m]<x )
l=m+1;
else
if ( v[m]>x )
u=m-1;
else return m;
}
return NOTFOUND;
}
/*-----------------------------------------------*/
/* insert values into an array t */
/*-----------------------------------------------*/
void insert_values(int t[])
{
int i;
printf ("Enter %d sorted values\n\n",T_SIZE );
for (i=0 ; i<T_SIZE; i++)
{
printf("%d==> ",i);
scanf("%d",&t[i]);
if (i>0 && t[i]<t[i-1])
{
printf("The input is not sorted TRy again!!");
i--;
}
}
return;
}
Bubble Sort
#include <conio.h>
#include <stdio.h>
#define T_SIZE 8
void bubble_sort (int [], int);
void insert_values(int [],int);
void main()
{
int i;
int t[T_SIZE];
clrscr();
insert_values(t,T_SIZE);
bubble_sort(t,T_SIZE);
for (i=0;i<T_SIZE;i++)
printf("\n%d",t[i]);
}
/*-----------------------------------------------*/
/* insert values into an array */
/*-----------------------------------------------*/
void insert_values(int v[],int v_size)
{
int i;
printf ("Enter %d values\n\n",v_size );
for (i=0 ; i<v_size; i++)
{
printf("%d==> ",i);
scanf("%d",&v[i]);
}
return;
}
/*----------------------------------------------------*/
/* bubble sort */
/*----------------------------------------------------*/
void bubble_sort(int v[],int v_size)
{
int i,j;
int x;
for(i=v_size - 1; i>=1 ; i--)
for (j=0 ; j<=i-1;j++)
if(v[j] > v[j+1] )
{ x=v[j];
v[j]=v[j+1];
v[j+1]=x;
}
return ;
}
Improved Bubble Sort
#include <conio.h>
#include <stdio.h>
#define T_SIZE 8
void improved_bubble_sort (int [], int);
void insert_values(int [],int);
void main()
{
int i;
int t[T_SIZE];
clrscr();
insert_values(t,T_SIZE);
improved_bubble_sort(t,T_SIZE);
for (i=0;i<T_SIZE;i++)
printf("\n%d",t[i]);
}
/*-----------------------------------------------*/
/* insert values into an array */
/*-----------------------------------------------*/
void insert_values(int v[],int v_size)
{
int i;
printf ("Enter %d values\n\n",v_size );
for (i=0 ; i<v_size; i++)
{
printf("%d==> ",i);
scanf("%d",&v[i]);
}
return;
}
/*----------------------------------------------------*/
/* improved bubble sort */
/*----------------------------------------------------*/
void improved_bubble_sort(int v[],int v_size)
{
int i,j;
int x;
int sw=1;
for(i=v_size - 1; i>=1 && sw==1 ; i--)
{
sw=0;
for (j=0 ; j<=i-1;j++)
if(v[j] > v[j+1] )
{ x=v[j];
v[j]=v[j+1];
v[j+1]=x;
sw=1;
}
}
return ;
}
Incremented Min Sort
#include <conio.h>
#include <stdio.h>
#define T_SIZE 7
void min_sort (int [], int);
void insert_values(int [],int);
void main()
{
int i;
int t[T_SIZE];
clrscr();
insert_values(t,T_SIZE);
min_sort(t,T_SIZE);
for (i=0;i<T_SIZE;i++)
printf("\n%d",t[i]);
}
/*-----------------------------------------------*/
/* insert values into an array */
/*-----------------------------------------------*/
void insert_values(int v[],int v_size)
{
int i;
printf ("Enter %d values\n\n",v_size );
for (i=0 ; i<v_size; i++)
{
printf("%d==> ",i);
scanf("%d",&v[i]);
}
return;
}
/*----------------------------------------------------*/
/* min sort */
/*----------------------------------------------------*/
void min_sort(int v[],int v_size)
{
int i,j;
int x;
for(i=0; i<=v_size - 2 ; i++)
for (j=i+1 ; j<=v_size -1;j++)
if(v[i] > v[j] )
{ x=v[i];
v[i]=v[j];
v[j]=x;
}
return ;
}
Sequential Search
#include <conio.h>
#include <stdio.h>
#define T_SIZE 5
#define NOTFOUND -1
int sequential_search (int [], int, int);
void insert_values(int []);
void main()
{
int i,x=1;
int t[T_SIZE];
clrscr();
insert_values(t);
while (x!=0)
{
printf("\nEnter a search value (0 to stop) ==>");
scanf ("%d",&x);
i=sequential_search(t,x,T_SIZE);
if (i == NOTFOUND )
printf("not found");
else
printf("found at location %d ", i);
}
}
/*-------------------------------------------------*/
/* sequential search */
/*-------------------------------------------------*/
int sequential_search(int v[] , int x, int v_size )
{
int i;
for (i=0;i<v_size;i++)
if ( v[i] == x )
return i;
return NOTFOUND;
}
/*-----------------------------------------------*/
/* insert values into an array t */
/*-----------------------------------------------*/
void insert_values(int t[])
{
int i;
printf ("Enter %d sorted values\n\n",T_SIZE );
for (i=0 ; i<T_SIZE; i++)
{
printf("%d==> ",i);
scanf("%d",&t[i]);
}
return;
}
merge and merge sort
#include<stdio.h>
#include<stdlib.h>
#include<mem.h>
#include<conio.h>
void merge(int a[],int na,int b[],int nb,int c[])
{
int ia=0,ib=0,ic=0;
while ((ia<na)&&(ib<nb))
if (a[ia]<b[ib])
c[ic++]=a[ia++];
else
c[ic++]=b[ib++];
while (ia<na)
c[ic++]=a[ia++];
while (ib<na)
c[ic++]=b[ib++];
}
void merge_sort(int key[])
{
int j,k,m,w[8];
for (k=1;k<8;k*=2){
for (j=0;j<8;j+=2*k)
merge(key+j,k,key+j+k,k,w+j);
for (j=0;j<8;++j)
key[j]=w[j];
}
}
void main()
{
clrscr();
int c[8]={1,8,4,9,1,3,12,5};
int i;
merge_sort(c);
for (i=0;i<8;i++)
printf("%d\n",c[i]);
}
Recursive merge sort
#include<stdio.h>
#include<stdlib.h>
#include<mem.h>
#include<conio.h>
void sort(int a[],int n);
void merge(int a[],int na,int b[],int nb,int c[]);
void merge_sort(int a[],int n,int tmp[]);
void merge(int a[],int na,int b[],int nb,int c[])
{
int ia=0,ib=0,ic=0;
while ((ia<na)&&(ib<nb))
if (a[ia]<b[ib])
c[ic++]=a[ia++];
else
c[ic++]=b[ib++];
while (ia<na)
c[ic++]=a[ia++];
while (ib<nb)
c[ic++]=b[ib++];
}
void sort(int a[],int n)
{
int *tmp;
int i;
tmp=(int *)calloc(n,sizeof(int));
merge_sort(a,n,tmp);
free(tmp);
}
void merge_sort(int a[],int n,int tmp[])
{
int m=n/2;
if (n<=1) return;
merge_sort(a,m,tmp);
merge_sort(a+m,n-m,tmp);
merge(a,m,a+m,n-m,tmp);
memcpy(a,tmp,n*2);
}
void main()
{
clrscr();
int c[16]={4,1,4,8,12,5,3,6,12,23,42,2,7,3};
int i;
sort(c,16);
for (i=0;i<16;i++)
printf("%d\n",c[i]);
}
QSort Example
#include<stdio.h>
#include<conio.h>
void qsort(int a[],int n);
void main()
{
int i,a[8]={1,5,3,8,2,9,4,3};
qsort(a,8);
for (i=0;i<8;i++)
printf("%d\n",a[i]);
}
void qsort(int a[],int n)
{
int p,b,t,temp;
if(n<2) return;
if (n==2)
{
if (a[0]>a[1])
{
temp=a[0];
a[0]=a[1];
a[1]=temp;
}
return;
}
temp=a[0];
a[0]=a[n/2];
a[n/2]=temp;
p=a[0];
b=1;
t=n-1;
while(b<=t)
{
while(a[t]>=p && t>0) --t;
while(a[b]<p && b<n) ++b;
if (b<t)
{
temp=a[b];
a[b++]=a[t];
a[t--]=temp;
}
}
temp=a[0];
a[0]=a[t];
a[t]=temp;
qsort(a,t);
qsort(a+b,n-b);
}
qsort function example
#include<stdio.h>
#include<stdlib.h>
int comp(const void *a,const void *b)
{
const int *x = (int *)a;
const int *y = (int *)b;
int d= *x - *y;
return d>0?1:d==0?0:-1;
}
void main()
{
int i,d[]={2,7,3,9,1,5,2,8,6,4,1,7,3,43,23,54,65,76,88,5};
qsort(d,20,2,comp);
for (i=0;i<20;i++)
printf("%d\n",d[i]);
}
Using Strings
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int comp( const void *a, const void *b)
{
return( strcmp((char *)a,(char *)b) );
}
void main(void)
{
char name[20][15];
int x,n=5;
for(x=0;x<5;x++)
gets(name[x]);
qsort(name,n,sizeof(name[0]),comp);
for (x=0;x<5;x++)
printf("%s\n",name[x]);
}
Recursive Binary Search
#include <conio.h>
#include <stdio.h>
#define T_SIZE 5
#define NOTFOUND -1
int binary_search (int,int [], int, int);
void insert_values(int []);
void main()
{
int i,x=1;
int t[T_SIZE];
clrscr();
insert_values(t);
while (x!=0)
{ printf("\nEnter a search value (0 to stop) ==>");
scanf ("%d",&x);
i=binary_search(x,t,0,T_SIZE-1);
if (i == NOTFOUND )
printf("not found");
else
printf("found at location %d ", i);
}
}
/*-------------------------------------------------*/
/* binary search */
/*-------------------------------------------------*/
int binary_search(int x,int v[] , int b, int t)
{
int m=(b+t)/2;
if (x<v[b] || x>v[t])
return NOTFOUND;
if (x==v[m])
return m;
if (x<v[m])
return binary_search(x,v,b,m-1);
return binary_search(x,v,m+1,t);
}
/*-----------------------------------------------*/
/* insert values into an array t */
/*-----------------------------------------------*/
void insert_values(int t[])
{
int i;
printf ("Enter %d sorted values\n\n",T_SIZE );
for (i=0 ; i<T_SIZE; i++)
{
printf("%d==> ",i);
scanf("%d",&t[i]);
if (i>0 && t[i]<t[i-1])
{
printf("The input is not sorted TRy again!!");
i--;
}
}
return;
}
Recent Stories
Top DiscoverSDK Experts
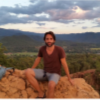
Mendy Bennett
Experienced with Ad network & Ad servers.
Mobile | Ad Networks and 1 more
View Profile
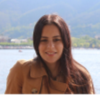
Karen Fitzgerald
7 years in Cross-Platform development.
Mobile | Cross Platform Frameworks
View Profile
X
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}Now comparing:
{{product.ProductName | createSubstring:25}} X
{{CommentsModel.TotalCount}} Comments
Your Comment