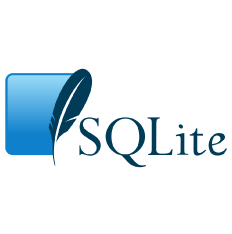
What is it all about?
SQLite is a relational database system.It supports ACID, and implements most of SQL (Standard Query Language). Unlike many other relational databases, SQLite whole system is linked to the application that uses it, so a separate ODBC connection, the database manager or database server is not required. The database itself can be regarded as entirely computer memory, or stored in a single file that is locked in for a period of transactions.
Key Features
◾Transactions are atomic, consistent, isolated, and durable (ACID) even after system crashes and power failures. ◾Zero-configuration - no setup or administration needed. ◾Full SQL implementation with advanced features like partial indexes and common table expressions. ◾A complete database is stored in a single cross-platform disk file. Great for use as an application file format. ◾Supports terabyte-sized databases and gigabyte-sized strings and blobs. (Seelimits.html.). ◾Small code footprint: less than 500KiB fully configured or much less with optional features omitted. ◾Simple, easy to use API.Written in ANSI-C. TCL bindings included. Bindings for dozens of other languages available separately. Well-commented source code with 100% branch test coverage. ◾Available as a single ANSI-C source-code file that is easy to compile and hence is easy to add into a larger project. ◾Self-contained: no external dependencies. Cross-platform: Android, *BSD, iOS, Linux, Mac, Solaris, VxWorks, and Windows (Win32, WinCE, WinRT) are supported out of the box. Easy to port to other systems. ◾Sources are in the public domain. Use for any purpose. ◾Comes with a standalone command-line interface (CLI) client that can be used to administer SQLite databases.
Resources
Resource Type |
Link |
---|---|
introduction | http://zetcode.com/db/sqlite/introduction/ |
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment