Using Pipes
Child Code
#include<stdio.h>
#include<unistd.h>
#include<stdlib.h>
int main(int argc,char *argv[])
{
char buf[11];
int pipedesc;
puts("child started");
pipedesc = atoi(argv[0]);
while(1)
{
read(pipedesc,buf,10);
puts(buf);
sleep(2);
}
return 0;
}
Parent Code
#include<stdio.h>
#include<unistd.h>
#include<stdlib.h>
int main(int argc,char *argv[])
{
char buf[11]="hello";
int pipedesc,i=0;
pipedesc = atoi(argv[0]);
puts("parent started:");
while(1)
{
i++;
sprintf(buf,"hello:%d",i);
write(pipedesc,buf,10);
sleep(2);
}
return 0;
}
Running All
#include<stdio.h>
#include<unistd.h>
int main()
{
int arr[2];
char argv[30];
pipe(arr);
if(fork())
{
puts("starting parent");
close(arr[0]);
sprintf(argv,"%d",arr[1]);
execlp("./pipeExampleParent",argv,NULL);
}
else
{
puts("starting child");
close(arr[1]);
sprintf(argv,"%d",arr[0]);
execlp("./pipeExample",argv,NULL);
}
return 1;
}
Recent Stories
Top DiscoverSDK Experts
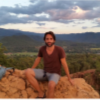
Mendy Bennett
Experienced with Ad network & Ad servers.
Mobile | Ad Networks and 1 more
View Profile
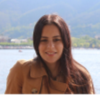
Karen Fitzgerald
7 years in Cross-Platform development.
Mobile | Cross Platform Frameworks
View Profile
X
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}Now comparing:
{{product.ProductName | createSubstring:25}} X
{{CommentsModel.TotalCount}} Comments
Your Comment