Creating Service
We can use Android Binder to communicate between apps. We need to create a service on the provider side and use Android Interface Definition Language (AIDL) to communicate with it
Example AIDL:
package com.example.myservicelib;
interface IMyService
{
int add(int a,int b);
int sub(int a,int b);
int mul(int a,int b,int c);
int sum(in int []arr);
}
Service Code
package com.example.service1;
import android.os.RemoteException;
import com.example.myservicelib.IMyService;
public class ServiceImp extends IMyService.Stub{
@Override
public int mul(int a, int b,int c) throws RemoteException {
// TODO Auto-generated method stub
return a*b*c;
}
@Override
public int add(int a, int b) throws RemoteException {
// TODO Auto-generated method stub
return a+b;
}
@Override
public int sub(int a, int b) throws RemoteException {
// TODO Auto-generated method stub
return a-b;
}
@Override
public int sum(int[] arg0) throws RemoteException {
// TODO Auto-generated method stub
return 0;
}
}
And the Service class:
package com.example.service1;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
public class MyService extends Service{
@Override
public IBinder onBind(Intent intent) {
// TODO Auto-generated method stub
return new ServiceImp();
}
}
Manifest File
<service android:name=".MyService">
<intent-filter >
<action android:name="com.example.service1.MyService" />
</intent-filter>
</service>
Client Code
package com.example.myclient;
import com.example.myservicelib.IMyService;
import android.os.Bundle;
import android.os.IBinder;
import android.os.RemoteException;
import android.app.Activity;
import android.content.ComponentName;
import android.content.Intent;
import android.content.ServiceConnection;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends Activity implements ServiceConnection {
IMyService myservice;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button b=(Button)findViewById(R.id.button1);
b.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
try {
int res=myservice.add(33, 44);
Toast.makeText(MainActivity.this, "res="+res, Toast.LENGTH_LONG).show();
} catch (RemoteException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
});
}
@Override
protected void onStart() {
// TODO Auto-generated method stub
super.onStart();
bindService(new Intent("com.example.service1.MyService"), this, BIND_AUTO_CREATE);
}
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
// TODO Auto-generated method stub
myservice = IMyService.Stub.asInterface(service);
}
@Override
public void onServiceDisconnected(ComponentName name) {
// TODO Auto-generated method stub
}
}
Recent Stories
Top DiscoverSDK Experts
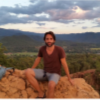
Mendy Bennett
Experienced with Ad network & Ad servers.
Mobile | Ad Networks and 1 more
View Profile
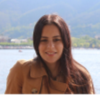
Karen Fitzgerald
7 years in Cross-Platform development.
Mobile | Cross Platform Frameworks
View Profile
X
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}Now comparing:
{{product.ProductName | createSubstring:25}} X
{{CommentsModel.TotalCount}} Comments
Your Comment